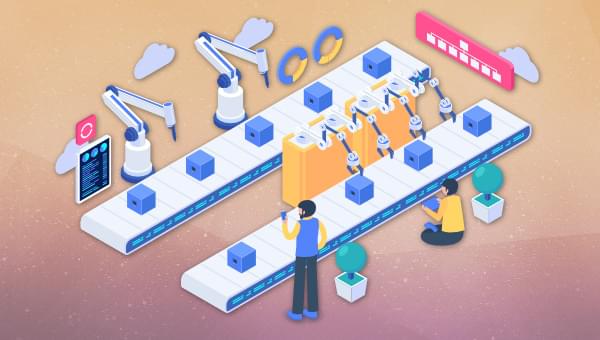
WebAssembly and JavaScript are two pivotal technologies in modern web development, each with distinct strengths and applications. This article provides a comparison of WebAssembly and JavaScript, examining their performance, portability, ease of use, security, and community support. Additionally, it explores the latest trends and innovations, helping you understand the evolving landscape of web development. By the end of this article, you’ll have a clear understanding of the scenarios where each technology excels and how to leverage their capabilities for your web development projects.
WebAssembly vs JavaScript: A Head-to-head Comparison
Performance
WebAssembly (Wasm) typically outperforms JavaScript for specific tasks due to its binary instruction format, which allows for near-native speed execution in modern web browsers. This low-level nature of Wasm enables efficient execution by browser engines, making it particularly advantageous for performance-intensive tasks like number crunching, data processing, and game rendering. Despite its speed benefits, Wasm is designed to complement rather than replace JavaScript. JavaScript remains the dominant language for web development because of its ease of use, flexibility, and extensive ecosystem, while WebAssembly is leveraged for tasks that require enhanced performance.
Portability and compatibility
Both Wasm and JavaScript are highly portable, working across all major browsers and platforms.
Wasm has a unique advantage: it supports multiple programming languages. You can write in C, C++, Rust, and more, compile to Wasm, and run it on the Web. This opens the door for more developers and allows code reuse from other environments.
On the other hand, JavaScript enjoys universal support and boasts a massive ecosystem of frameworks and libraries, making it the default for most web projects.
Ease of use
JavaScript’s low learning curve and dynamic nature make it beginner-friendly. With extensive documentation and a vibrant community, JavaScript is accessible and productive. Tools and frameworks abound, simplifying development.
WebAssembly, while powerful, is more complex. It requires knowledge of languages like C++ or Rust and an understanding of the compilation process. Its ecosystem is also still maturing, offering fewer resources than JavaScript.
Security
Both Wasm and JavaScript run in sandboxed environments, safeguarding the host system. However, JavaScript’s dynamic nature can lead to vulnerabilities like cross-site scripting (XSS) if not handled properly. Wasm’s binary format and structure make it more resistant to certain attacks, such as code injection. But as with any technology, best practices are essential to ensure security.
Community and ecosystem
JavaScript’s community is enormous, with millions of developers and a mature ecosystem of libraries, frameworks, and tools. Resources like Stack Overflow, GitHub, and numerous online courses provide ample support.
WebAssembly’s community, while smaller, is growing fast. Organizations like the Bytecode Alliance are expanding the ecosystem with new tools and libraries, and as more companies adopt Wasm for high-performance applications, this trend is expected to continue.
What’s Trending with WebAssembly and JavaScript
Adoption and usage
WebAssembly is making waves in 2024, with adoption up by 23% from last year. Industries like gaming, finance, and healthcare are using Wasm to build high-performance web apps that require real-time processing.
Meanwhile, JavaScript remains the web development kingpin, with over 60% of developers using it regularly. Its versatility and extensive ecosystem make it indispensable for a wide range of applications.
Innovations and updates
WebAssembly has had a big year. The WebAssembly System Interface (WASI) now makes it easier to run Wasm outside the browser, opening up new use cases like server-side applications and IoT devices. The WebAssembly component model has also improved, making modularization and reuse of Wasm modules more efficient.
JavaScript continues to evolve with new ECMAScript proposals. In 2024, features like enhanced pattern matching, better async programming capabilities, and improved modularity have been standardized. These updates aim to make JavaScript more robust and versatile, addressing common developer pain points.
Tooling and frameworks
WebAssembly’s tooling has come a long way. Projects like Wasmtime and Wasmer have simplified running Wasm on servers, and tools like wasm-pack make integrating Wasm into web apps easier. The Bytecode Alliance is actively developing new libraries and tools to support Wasm development.
JavaScript frameworks like React, Vue and Angular keep getting better, with updates focused on performance, developer experience, and modern web standards integration. New frameworks and tools continue to emerge, showcasing the dynamic nature of the JavaScript ecosystem.
Real-World Success Stories
Many companies are reaping the benefits of WebAssembly. AutoDesk has used Wasm to boost the performance of its CAD tools, delivering a faster and more responsive user experience. Financial institutions are leveraging Wasm for real-time complex calculations, enhancing the speed and accuracy of their trading platforms.
JavaScript remains a powerhouse in web development. Companies like Airbnb and Netflix rely on JavaScript to build smooth, interactive front-end interfaces. The versatility of JavaScript allows these companies to quickly iterate and deploy new features, maintaining their competitive edge.
Example Code
WebAssembly example: A simple addition function
This example shows how to use WebAssembly with Rust and JavaScript to perform a simple addition of two numbers.
1.Rust Code (src/lib.rs)
<code class="rust language-rust"> <span class="token attribute attr-name">#[no_mangle]</span> <span class="token keyword">pub</span> <span class="token keyword">extern</span> <span class="token string">"C"</span> <span class="token keyword">fn</span> <span class="token function-definition function">add</span><span class="token punctuation">(</span>a<span class="token punctuation">:</span> <span class="token keyword">i32</span><span class="token punctuation">,</span> b<span class="token punctuation">:</span> <span class="token keyword">i32</span><span class="token punctuation">)</span> <span class="token punctuation">-></span> <span class="token keyword">i32</span> <span class="token punctuation">{</span> a <span class="token operator">+</span> b <span class="token punctuation">}</span> </code>
Explanation in simple terms:
#[no_mangle]
. This attribute tells the Rust compiler not to alter the name of the function, ensuring it can be called from other languages or environments.pub extern "C" fn add(a: i32, b: i32) -> i32
. This defines a public function with C linkage, which can be called from JavaScript or any other language that can interact with WebAssembly. The function takes twoi32
(32-bit integer) arguments and returns their sum.
2. Compiling to WebAssembly
<code class="bash language-bash">rustup target <span class="token function">add</span> wasm32-unknown-unknown cargo build --target wasm32-unknown-unknown --release </code>
rustup target add wasm32-unknown-unknown
. Adds the WebAssembly target to your Rust toolchain.cargo build --target wasm32-unknown-unknown --release
. Compiles the Rust code to WebAssembly in release mode, generating a.wasm
file.
3.JavaScript integration
<code class="javascript language-javascript"><span class="token keyword">async</span> <span class="token keyword">function</span> <span class="token function">loadWasm</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span> <span class="token keyword">const</span> response <span class="token operator">=</span> <span class="token keyword control-flow">await</span> <span class="token function">fetch</span><span class="token punctuation">(</span><span class="token string">'path/to/your.wasm'</span><span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token keyword">const</span> buffer <span class="token operator">=</span> <span class="token keyword control-flow">await</span> response<span class="token punctuation">.</span><span class="token method function property-access">arrayBuffer</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token keyword">const</span> <span class="token punctuation">{</span> instance <span class="token punctuation">}</span> <span class="token operator">=</span> <span class="token keyword control-flow">await</span> <span class="token known-class-name class-name">WebAssembly</span><span class="token punctuation">.</span><span class="token method function property-access">instantiate</span><span class="token punctuation">(</span>buffer<span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token console class-name">console</span><span class="token punctuation">.</span><span class="token method function property-access">log</span><span class="token punctuation">(</span>instance<span class="token punctuation">.</span><span class="token property-access">exports</span><span class="token punctuation">.</span><span class="token method function property-access">add</span><span class="token punctuation">(</span><span class="token number">2</span><span class="token punctuation">,</span> <span class="token number">3</span><span class="token punctuation">)</span><span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token punctuation">}</span> <span class="token function">loadWasm</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span> </code>
Explanation in simple terms:
- Fetching the WebAssembly file. This part of the code fetches the
.wasm
file we created from the web server. - Using the WebAssembly function:
response.arrayBuffer()
. Prepares the WebAssembly file for use.WebAssembly.instantiate(buffer)
. Loads the WebAssembly file so we can use its functions.instance.exports.add(2, 3)
. Calls theadd
function from the WebAssembly file with the numbers2
and3
, and prints the result (5
).
Where to put this code:
- Rust Code. Save this in a file named
lib.rs
in your Rust project’ssrc
folder. - Compiling Command. Run the compile commands in your terminal.
- JavaScript Code. Save this in a
.JavaScript
file, likeloadWasm.JavaScript
, and link it in your HTML file.
JavaScript example: Fetching data from an API
This example demonstrates how to use JavaScript to fetch data from an online service (API) and display it on a web page.
HTML (index.html):
<code class="markup language-markup"><span class="token doctype"><span class="token punctuation"><!</span><span class="token doctype-tag">DOCTYPE</span> <span class="token name">html</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>html</span> <span class="token attr-name">lang</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token punctuation">"</span>en<span class="token punctuation">"</span></span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>head</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>meta</span> <span class="token attr-name">charset</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token punctuation">"</span>UTF-8<span class="token punctuation">"</span></span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>meta</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token punctuation">"</span>viewport<span class="token punctuation">"</span></span> <span class="token attr-name">content</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token punctuation">"</span>width=device-width, initial-scale=1.0<span class="token punctuation">"</span></span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>title</span><span class="token punctuation">></span></span>Fetch API Example<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>title</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"></</span>head</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>body</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>div</span> <span class="token attr-name">id</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token punctuation">"</span>data<span class="token punctuation">"</span></span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>div</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>script</span> <span class="token attr-name">src</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token punctuation">"</span>app.JavaScript<span class="token punctuation">"</span></span><span class="token punctuation">></span></span><span class="token script"></span><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>script</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"></</span>body</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"></</span>html</span><span class="token punctuation">></span></span> </code>
JavaScript (app.JavaScript):
<code class="javascript language-javascript"><span class="token keyword">async</span> <span class="token keyword">function</span> <span class="token function">fetchData</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span> <span class="token keyword">const</span> response <span class="token operator">=</span> <span class="token keyword control-flow">await</span> <span class="token function">fetch</span><span class="token punctuation">(</span><span class="token string">'https://api.example.com/data'</span><span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token keyword">const</span> data <span class="token operator">=</span> <span class="token keyword control-flow">await</span> response<span class="token punctuation">.</span><span class="token method function property-access"><span class="token maybe-class-name">JavaScripton</span></span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token dom variable">document</span><span class="token punctuation">.</span><span class="token method function property-access">getElementById</span><span class="token punctuation">(</span><span class="token string">'data'</span><span class="token punctuation">)</span><span class="token punctuation">.</span><span class="token property-access">innerText</span> <span class="token operator">=</span> <span class="token maybe-class-name">JavaScriptON</span><span class="token punctuation">.</span><span class="token method function property-access">stringify</span><span class="token punctuation">(</span>data<span class="token punctuation">,</span> <span class="token keyword null nil">null</span><span class="token punctuation">,</span> <span class="token number">2</span><span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token punctuation">}</span> <span class="token function">fetchData</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span> </code>
Explanation in simple terms:
- HTML structure. The HTML file sets up a simple webpage with a section (
) to display the fetched data. It also includes the JavaScript file (
app.JavaScript
). - Fetching data with JavaScript.
fetch('https://api.example.com/data')
. This line tells the browser to request data from an online address (API).await response.JavaScripton()
. Converts the received data into a format (JavaScriptON) that we can easily work with.document.getElementById('data').innerText = JavaScriptON.stringify(data, null, 2)
. Displays the fetched data on the web page, formatted nicely.
Where to put this code:
- HTML file. Save this code in a file named
index.html
. - JavaScript file. Save the JavaScript code in a file named
app.JavaScript
and make sure it’s linked in the HTML file as shown.
Summary
- WebAssembly example. Shows how to add two numbers using Rust and WebAssembly, and then use this function in a web page with JavaScript.
- JavaScript example. Demonstrates fetching data from an API and displaying it on a web page.
Both examples illustrate how you can integrate powerful functionalities into your web projects using modern web development techniques.
WebAssembly vs JavaScript: Pros and Cons
Pros of WebAssembly
1. Blazing fast performance
Wasm’s most significant advantage is its exceptional speed. It executes code at near-native speeds, making it ideal for computationally intensive tasks like gaming, 3D rendering, image/video processing, and scientific simulations. This performance boost is achieved through several factors, including:
- Ahead-of-time (AOT) compilation. Wasm code is compiled before it reaches the browser, resulting in faster execution compared to JavaScript’s just-in-time (JIT) compilation.
- Static typing. Wasm’s static typing system allows for aggressive code optimizations during compilation, further enhancing performance.
- Direct access to hardware. Wasm provides a low-level virtual machine that allows developers to tap into hardware capabilities like SIMD (Single Instruction, Multiple Data) instructions, unlocking the full potential of modern processors.
2. Polyglot programming
Wasm isn’t limited to a single programming language. It supports multiple languages, including C, C++, Rust, Go, and more. This flexibility empowers developers to leverage their existing skillsets and choose the language that best suits their project requirements.
3. Enhanced security
Wasm runs in a sandboxed environment, providing an additional layer of security compared to JavaScript. This isolation helps prevent malicious code from accessing sensitive data or compromising system resources.
Cons of WebAssembly
1. Limited ecosystem
Despite its growing popularity, Wasm’s ecosystem is still relatively young compared to JavaScript. The number of libraries, frameworks, and tools specifically designed for Wasm is limited, which might require additional effort from developers.
2. Steeper learning curve
Working with Wasm often involves dealing with low-level concepts like memory management and compilation, which can be challenging for developers accustomed to high-level languages.
3. Browser compatibility
While major browsers support Wasm, there might be slight variations in implementation and features across different browsers, which could necessitate additional testing and adjustments.
Pros of JavaScript
JavaScript is the backbone of interactive web experiences. It powers everything from simple animations to complex single-page applications. Its ubiquity, vast ecosystem, and ease of use have solidified its position as the most popular web development language. Here are some of its pros.
- Ubiquitous and versatile
JavaScript is supported by virtually every web browser, making it the most accessible language for web development. It’s not only used for frontend development but also for server-side development (Node), mobile applications (React Native), and even desktop applications (Electron).
- Vast ecosystem
The JavaScript ecosystem is incredibly rich, offering a plethora of libraries (React, Vue, Angular), frameworks, and tools for every imaginable task. This abundance of resources streamlines development and empowers developers to build complex applications quickly.
- Easy to learn
JavaScript’s syntax is relatively forgiving and easy to grasp, making it an excellent choice for beginners. The extensive community support and online tutorials further facilitate the learning process.
Cons of JavaScript
- Performance bottlenecks
While JavaScript engines have significantly improved over the years, it can still face performance limitations when dealing with computationally intensive tasks. This is where Wasm shines.
- Single-threaded nature
JavaScript is inherently single-threaded, meaning it can only execute one task at a time. Although mechanisms like Web Workers and asynchronous programming mitigate this limitation, it can still pose challenges for certain applications.
Choosing between Wasm and JavaScript depends on your project’s needs. If you need top-notch performance for tasks like gaming or complex simulations, Wasm is your best bet. If you prioritize ease of use, broad compatibility, and access to a vast ecosystem, JavaScript is the way to go.
Future Outlook
WebAssembly’s future is bright, with increasing adoption and a growing ecosystem. More tools and libraries will make Wasm more accessible to developers, and its potential outside the browser is vast, from server-side applications to IoT.
JavaScript will continue to dominate web development. Ongoing standardization and a vibrant community will ensure JavaScript adapts to new challenges. As web applications become more complex, JavaScript’s versatility and extensive ecosystem will remain invaluable.
Conclusion
WebAssembly and JavaScript each have their own strengths and play crucial roles in modern web development. Wasm excels in performance-critical scenarios, while JavaScript is indispensable for interactive and responsive web applications. Understanding their differences and latest trends helps developers make informed decisions and use the best tools for their projects.
As we advance, it’s essential for developers to experiment with both WebAssembly and JavaScript. Staying updated with these technologies will equip you to tackle the challenges of modern web development. Whether you’re building high-performance apps with Wasm or dynamic interfaces with JavaScript, the future of web development is full of exciting possibilities.