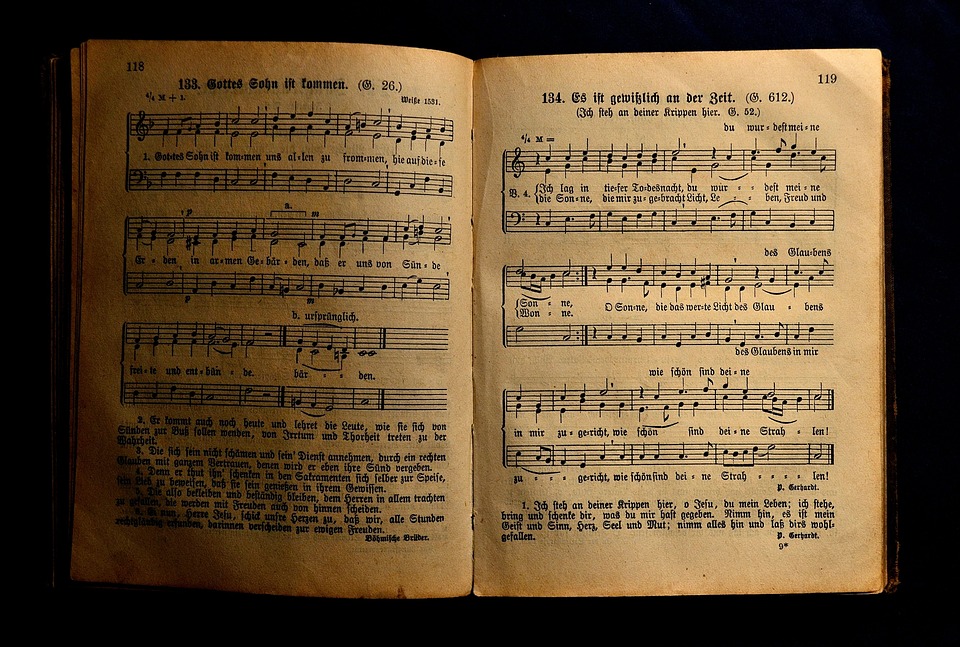
JavaScript split method is a powerful tool that allows developers to easily separate a string into an array of substrings based on a specified separator. This method can be incredibly useful for a variety of tasks, from parsing data to manipulating strings. However, the split method can be a bit tricky to understand for beginners, as there are a few nuances and potential pitfalls to be aware of. In this comprehensive guide, we will unravel the mysteries of the JavaScript split method, covering everything you need to know to use it effectively in your projects.
The basic syntax of the split method is as follows:
“`javascript
string.split(separator, limit)
“`
Where `string` is the original string that you want to split, `separator` is the character or regular expression that you want to use to split the string, and `limit` is an optional parameter that specifies the maximum number of splits to perform. If `separator` is omitted, the entire string will be split into individual characters.
One important thing to note about the split method is that it does not modify the original string – instead, it returns a new array of substrings. This means that you can use the split method without worrying about accidentally altering your data.
Let’s take a look at a few examples to illustrate how the split method works:
“`javascript
const str = ‘Hello, World!’;
const words = str.split(‘ ‘); // [‘Hello,’, ‘World!’]
“`
In this example, we use a space character as the separator to split the string into an array of words.
“`javascript
const str = ‘apple, banana, cherry’;
const fruits = str.split(‘, ‘); // [‘apple’, ‘banana’, ‘cherry’]
“`
Here, we use a comma and a space as the separator to split the string into an array of fruits.
It’s worth noting that the split method can also accept regular expressions as separators, allowing for more advanced splitting logic. For example:
“`javascript
const str = ‘apples, bananas, cherries’;
const fruits = str.split(/\s*,\s*/); // [‘apples’, ‘bananas’, ‘cherries’]
“`
In this case, we use a regular expression to split the string by commas, with optional whitespace before and after each comma.
One potential pitfall to be aware of when using the split method is that it can behave unexpectedly when the separator is a special character in regular expressions. For example:
“`javascript
const str = ‘1+2+3+4’;
const numbers = str.split(‘+’); // [‘1’, ‘2’, ‘3’, ‘4’]
“`
In this case, we want to split the string by the plus sign, but because the plus sign is a special character in regular expressions, it needs to be escaped with a backslash to be treated as a literal character:
“`javascript
const str = ‘1+2+3+4’;
const numbers = str.split(/\+/); // [‘1’, ‘2’, ‘3’, ‘4’]
“`
By escaping the plus sign in the regular expression, we ensure that it is treated as a literal character and correctly splits the string.
In conclusion, the JavaScript split method is a versatile and powerful tool for splitting strings into arrays of substrings. By understanding the syntax and nuances of the split method, you can effectively use it in your projects to manipulate and parse strings with ease. With the knowledge gained from this comprehensive guide, you can confidently unravel the mysteries of the JavaScript split method and harness its full potential in your development work.