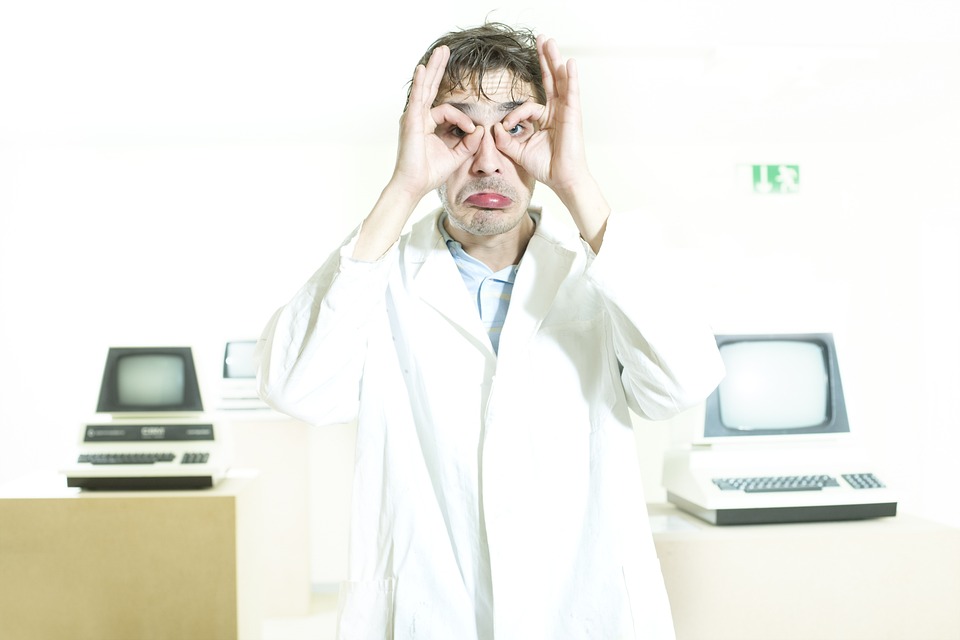
JavaScript split is a powerful method that allows developers to easily manipulate and work with strings in their code. However, it can be a bit tricky to understand and use effectively. In this comprehensive guide, we’ll unravel the mysteries of JavaScript split and show you how to use it like a pro.
What is JavaScript split?
JavaScript split is a method that allows you to split a string into an array of substrings based on a specified delimiter. This delimiter can be a single character, a string, or even a regular expression. The split method returns an array of substrings, which can then be manipulated or used in other parts of your code.
How to use JavaScript split
To use the split method in JavaScript, simply call it on a string and pass in the delimiter as an argument. For example:
let str = “Hello,world”;
let arr = str.split(“,”);
In this example, we’re splitting the string “Hello, world” based on the comma delimiter “,”. The split method will return an array with two elements: “Hello” and “world”.
You can also use regular expressions as delimiters with the split method. For example, if you want to split a string based on whitespace, you can do:
let str = “Hello world”;
let arr = str.split(/\s+/);
In this case, the split method will split the string “Hello world” based on one or more whitespace characters. The resulting array will contain two elements: “Hello” and “world”.
Handling empty strings with JavaScript split
One thing to keep in mind when using the split method is how it handles empty strings. By default, the split method will remove any empty strings from the resulting array. For example:
let str = “Hello,,world”;
let arr = str.split(“,”);
In this case, the split method will return an array with two elements: “Hello” and “world”. The empty string between the two commas has been removed from the array.
If you want to keep empty strings in the resulting array, you can pass in a second argument to the split method. For example:
let str = “Hello,,world”;
let arr = str.split(“,”, -1);
In this case, the split method will return an array with three elements: “Hello”, “”, and “world”. The second argument “-1” tells the split method to keep all empty strings in the resulting array.
Using JavaScript split for more complex tasks
While the split method is great for simple tasks like splitting a string based on a delimiter, it can also be used for more complex tasks. For example, you can use the split method to extract specific parts of a string or to manipulate the resulting array in various ways.
Overall, JavaScript split is a versatile and powerful method that can be used in a wide variety of scenarios. By understanding how it works and how to use it effectively, you can take your JavaScript coding skills to the next level. So go ahead and start unraveling the mysteries of JavaScript split today!