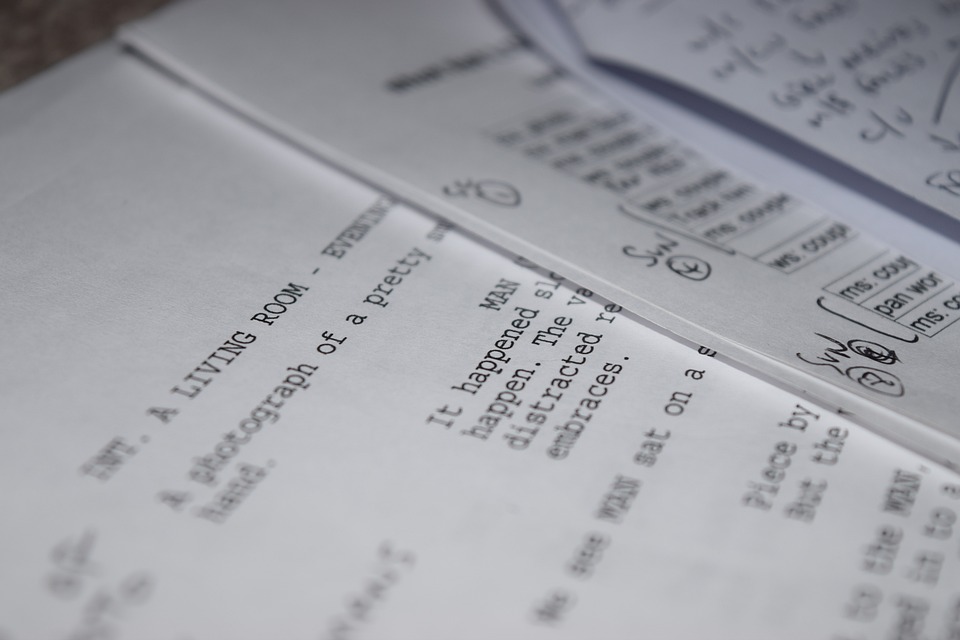
JavaScript is a powerful programming language that is widely used for creating dynamic and interactive websites. One of the key features of JavaScript is its ability to manipulate strings, which are sequences of characters such as letters, numbers, and special symbols. One useful string manipulation method in JavaScript is the split() method, which allows you to split a string into an array of substrings based on a specified delimiter.
For beginners, understanding how the split() method works can be a bit confusing. However, with some guidance and practice, you can easily unravel the mysteries of JavaScript split and use it to your advantage in your web development projects.
The syntax of the split() method is as follows:
string.split(separator, limit)
Here, “string” is the original string that you want to split, “separator” is the character or regular expression that will be used to split the string, and “limit” is an optional parameter that specifies the maximum number of elements in the resulting array.
For example, if you have the following string:
var str = “Hello, World!”;
You can split it into an array of substrings using the comma as the delimiter like this:
var arr = str.split(“,”);
The resulting array will be [“Hello”, ” World!”].
You can also use a regular expression as the separator. For example, if you want to split a string into an array of words, you can use the following code:
var str = “Hello, World!”;
var arr = str.split(/\s+/);
This will split the string based on one or more whitespace characters, resulting in an array of words [“Hello,”, “World!”].
The split() method can also be used to split a string into individual characters. For example, if you want to split a string into an array of characters, you can use an empty string as the separator like this:
var str = “Hello, World!”;
var arr = str.split(“”);
This will result in an array of individual characters [“H”, “e”, “l”, “l”, “o”, “,”, ” “, “W”, “o”, “r”, “l”, “d”, “!”].
In addition to splitting strings, the split() method can also be used to manipulate and reorganize the resulting array of substrings. For example, you can reverse the order of the substrings in the array like this:
var str = “Hello, World!”;
var arr = str.split(“”).reverse().join(“”);
This will first split the string into an array of characters, then reverse the order of the characters, and finally join them back together into a new string “Hello, World!”.
In conclusion, the split() method in JavaScript is a powerful tool for manipulating strings and extracting useful information from them. By understanding the syntax and various ways in which the split() method can be used, you can enhance your web development skills and create more dynamic and interactive websites. So, don’t be afraid to dive into the mysteries of JavaScript split and experiment with different examples to see how it can benefit your coding projects.