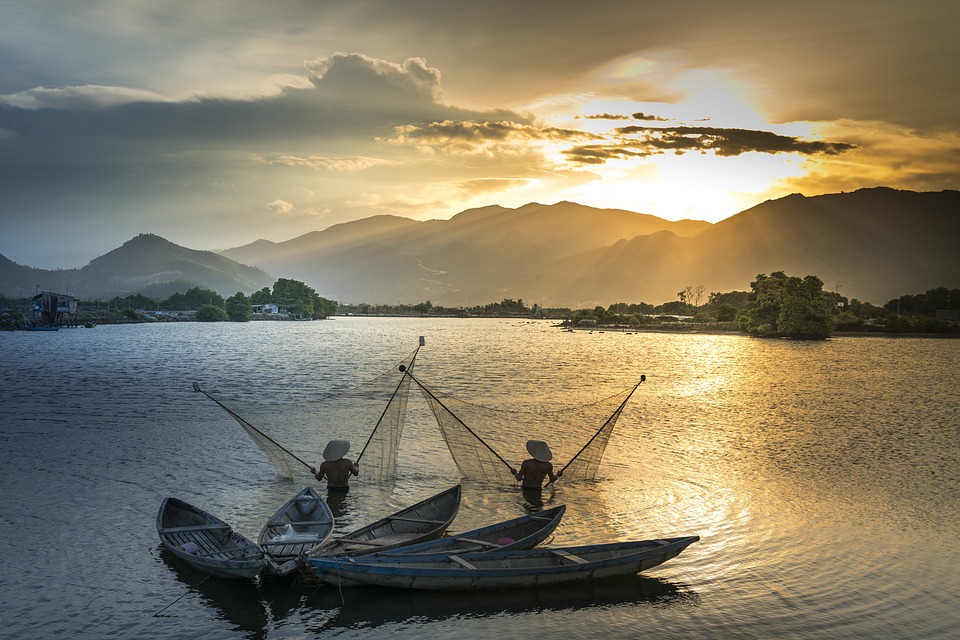
Concurrency is a crucial aspect of modern software development, allowing developers to efficiently utilize the resources of modern multi-core processors. In the Go programming language, concurrency is a first-class feature, making it easy to write highly performant and concurrent programs.
Go’s built-in support for concurrency comes in the form of goroutines and channels. Goroutines are lightweight threads that are managed by the Go runtime, allowing developers to easily spawn thousands of them without worrying about the overhead of traditional threads. Channels, on the other hand, provide a way for goroutines to communicate with each other, enabling synchronization and data sharing between concurrent tasks.
To unlock the full potential of concurrency in Go, developers need to understand how to effectively use goroutines and channels in their programs. Here are some tips to get started:
1. Use goroutines for parallelism: Goroutines are perfect for running tasks in parallel, making it easy to take advantage of multi-core processors. By using the `go` keyword before a function call, developers can spawn a new goroutine to execute that function concurrently with the rest of the program.
2. Communicate using channels: Channels provide a safe and efficient way for goroutines to communicate with each other. By sending and receiving data through channels, developers can coordinate the execution of concurrent tasks and share data between them. Channels can also be used to synchronize goroutines, ensuring that certain tasks are completed in a specific order.
3. Avoid shared mutable state: One of the biggest challenges in concurrent programming is dealing with shared mutable state. In Go, developers should strive to minimize shared state between goroutines, as it can lead to race conditions and other concurrency bugs. Instead, consider using channels to pass data between goroutines, or use tools like the `sync` package to safely access shared resources.
4. Use the `sync` package for synchronization: The `sync` package in Go provides a set of synchronization primitives, such as mutexes and wait groups, to help developers coordinate access to shared resources. By using these tools effectively, developers can ensure that their concurrent programs are free from data races and other synchronization issues.
5. Leverage the `select` statement: The `select` statement in Go allows developers to wait on multiple channels simultaneously, making it easy to handle multiple concurrent tasks in a non-blocking manner. By using `select`, developers can create highly responsive and efficient concurrent programs that can handle multiple input and output operations concurrently.
Overall, Go’s support for concurrency makes it a powerful language for writing highly performant and scalable software. By understanding how to effectively use goroutines and channels, developers can unlock the full potential of concurrency in Go and take advantage of the full power of modern multi-core processors.