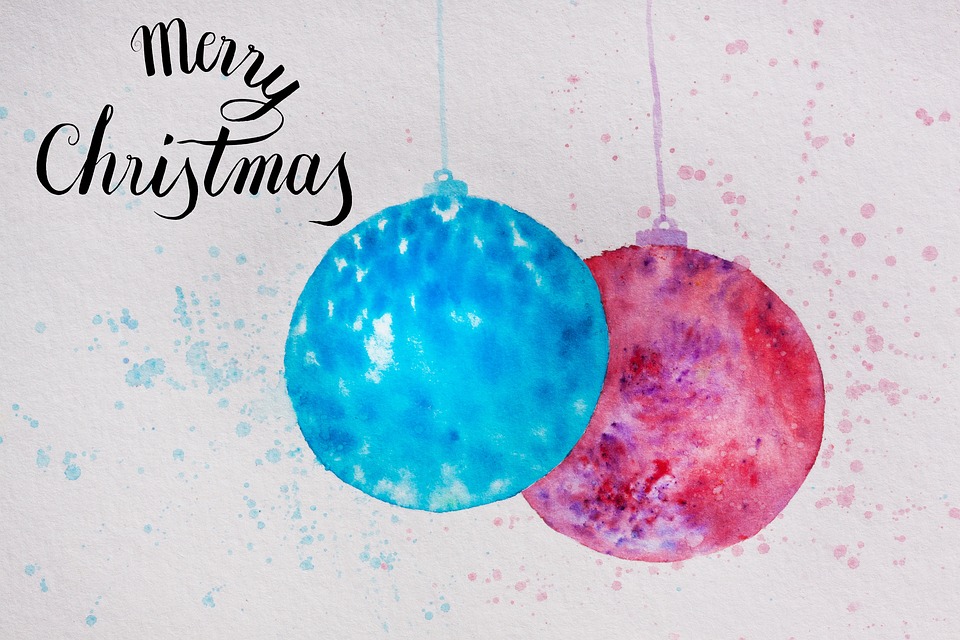
JavaScript is a powerful programming language that is widely used in web development. One of the most useful features of JavaScript is the Map object, which allows you to store key-value pairs and easily retrieve them later. In this article, we will explore how to unlock the power of JavaScript Map and use it effectively in your projects.
What is a JavaScript Map?
A JavaScript Map is a collection of key-value pairs where each key is unique. This makes it a useful data structure for storing and retrieving data based on a specific key. Maps are similar to objects in JavaScript, but they have some key differences. One of the main differences is that Maps can have any type of key, whereas objects can only have string keys.
Creating a JavaScript Map
To create a new Map in JavaScript, you can simply use the Map constructor like this:
“`javascript
const myMap = new Map();
“`
You can also initialize a Map with an array of key-value pairs like this:
“`javascript
const myMap = new Map([
[‘key1’, ‘value1’],
[‘key2’, ‘value2’],
[‘key3’, ‘value3’]
]);
“`
Adding and Retrieving Values from a Map
To add a new key-value pair to a Map, you can use the set() method like this:
“`javascript
myMap.set(‘key4’, ‘value4’);
“`
To retrieve a value from a Map, you can use the get() method like this:
“`javascript
const value = myMap.get(‘key4’);
“`
Iterating over a Map
You can easily iterate over the key-value pairs in a Map using the forEach() method like this:
“`javascript
myMap.forEach((value, key) => {
console.log(`${key}: ${value}`);
});
“`
You can also use the keys() and values() methods to get an iterator for the keys or values in a Map like this:
“`javascript
const keysIterator = myMap.keys();
const valuesIterator = myMap.values();
“`
Using Maps in Practice
Maps are a versatile data structure that can be used in various scenarios in web development. For example, you can use Maps to store user preferences, cache data for faster retrieval, or create a lookup table for mapping values to keys.
Here is a simple example of how you can use a Map to store user preferences in a web application:
“`javascript
const userPreferences = new Map();
userPreferences.set(‘theme’, ‘dark’);
userPreferences.set(‘language’, ‘english’);
userPreferences.set(‘fontSize’, ‘medium’);
console.log(userPreferences.get(‘theme’)); // Output: dark
“`
In conclusion, JavaScript Maps are a powerful tool that can help you organize and retrieve data efficiently in your web development projects. By understanding how to create, add, retrieve, and iterate over Maps, you can unlock their full potential and take your coding skills to the next level.