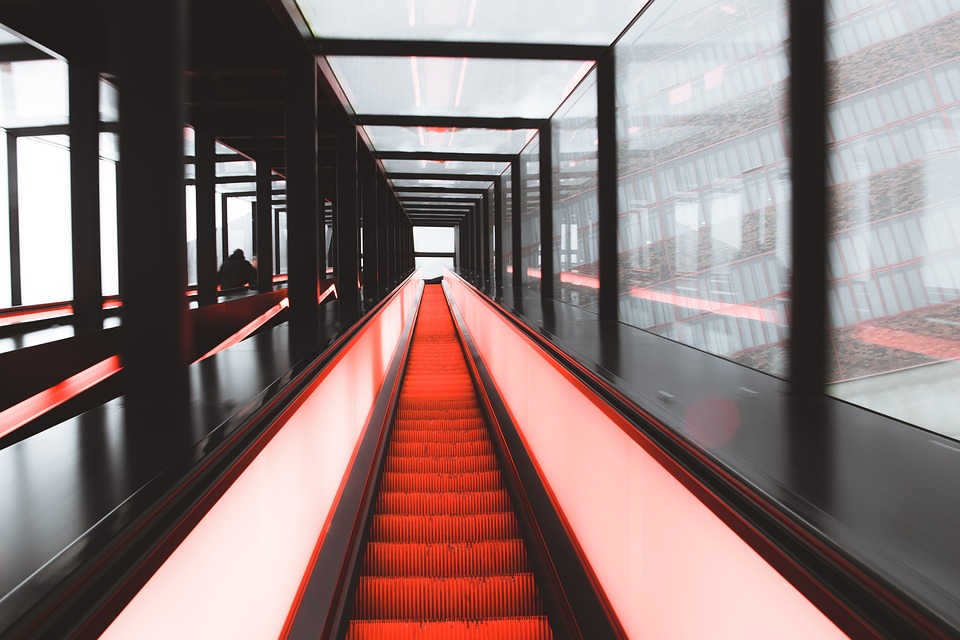
The Go programming language, also known as Golang, is a powerful and versatile language that is gaining popularity among developers for its efficiency and simplicity. Developed by Google, Go is designed to be fast, reliable, and easy to use, making it an ideal choice for building scalable and high-performance applications.
If you’re new to Go or looking to expand your skills, there are several must-try examples that can help you unlock the full potential of this powerful language. From basic syntax to more advanced concepts, these examples will help you become a proficient Go developer in no time.
1. Hello World
Like any programming language, the first program you should write in Go is the classic “Hello World” program. This simple example will introduce you to the basic syntax of Go and show you how to write and run a basic program. Here’s a simple “Hello World” program in Go:
“`go
package main
import “fmt”
func main() {
fmt.Println(“Hello, World!”)
}
“`
2. Variables and Data Types
Variables and data types are essential concepts in any programming language. In Go, you can declare variables using the `var` keyword and specify the data type. Here’s an example that demonstrates how to declare variables and use different data types in Go:
“`go
package main
import “fmt”
func main() {
var name string = “John”
var age int = 30
var isStudent bool = true
var salary float64 = 50000.50
fmt.Println(“Name:”, name)
fmt.Println(“Age:”, age)
fmt.Println(“Is Student:”, isStudent)
fmt.Println(“Salary:”, salary)
}
“`
3. Functions
Functions are reusable blocks of code that perform a specific task. In Go, you can define functions using the `func` keyword. Here’s an example that demonstrates how to define and call a function in Go:
“`go
package main
import “fmt”
func add(a, b int) int {
return a + b
}
func main() {
result := add(10, 20)
fmt.Println(“Result:”, result)
}
“`
4. Arrays and Slices
Arrays and slices are fundamental data structures in Go that allow you to store multiple values of the same type. Here’s an example that demonstrates how to declare and use arrays and slices in Go:
“`go
package main
import “fmt”
func main() {
// Array
var numbers [5]int
numbers[0] = 10
numbers[1] = 20
numbers[2] = 30
numbers[3] = 40
numbers[4] = 50
fmt.Println(“Array:”, numbers)
// Slice
var fruits = []string{“Apple”, “Banana”, “Orange”, “Mango”}
fmt.Println(“Slice:”, fruits)
}
“`
5. Concurrency
One of the key features of Go is its built-in support for concurrency. Goroutines are lightweight threads that allow you to run multiple tasks concurrently. Here’s an example that demonstrates how to create and run a Goroutine in Go:
“`go
package main
import (
“fmt”
“time”
)
func printNumbers() {
for i := 1; i