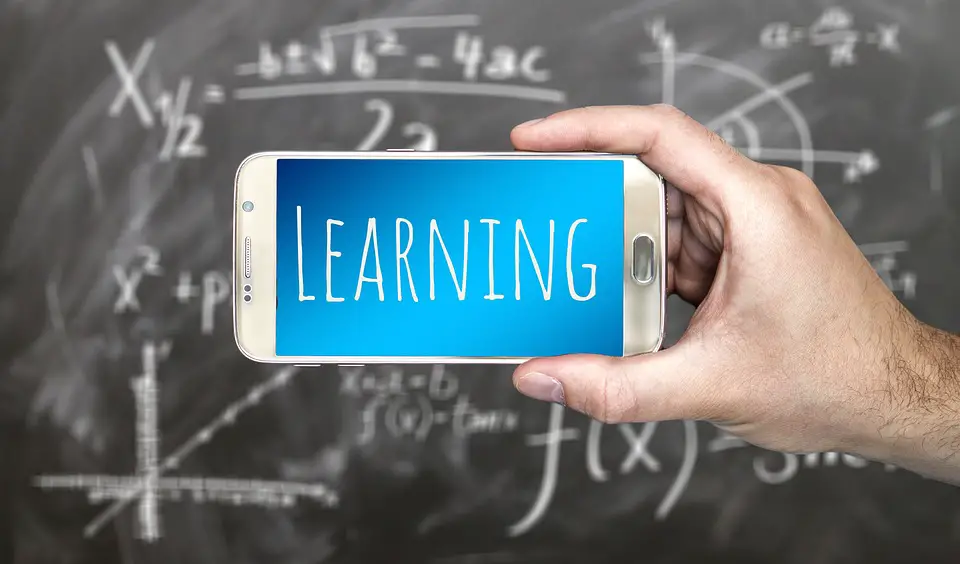
Python is a versatile and powerful programming language that is widely used by developers around the world. One of the key features that makes Python so popular is its extensive support for data structures, including lists. Python lists are a fundamental data structure that allows you to store and manipulate collections of items in a flexible and efficient way.
In this comprehensive guide, we will explore the power of Python lists and how you can unleash their full potential in your programming projects.
Creating Lists
To create a list in Python, you simply enclose a comma-separated sequence of items within square brackets. For example:
my_list = [1, 2, 3, 4, 5]
You can also create lists of different data types, such as strings, integers, and even other lists:
mixed_list = [1, “hello”, 3.14, [4, 5, 6]]
Accessing Elements
You can access individual elements in a list by using indexing. Indexing in Python starts at 0, so the first element in a list has an index of 0, the second element has an index of 1, and so on. For example:
print(my_list[0]) # Output: 1
print(my_list[2]) # Output: 3
You can also use negative indexing to access elements from the end of the list:
print(my_list[-1]) # Output: 5
print(my_list[-2]) # Output: 4
Slicing Lists
Python lists support slicing, which allows you to extract a subset of elements from a list. You can specify a start index, an end index, and a step size to create a slice of the list. For example:
print(my_list[1:4]) # Output: [2, 3, 4]
print(my_list[::2]) # Output: [1, 3, 5]
Modifying Lists
Python lists are mutable, which means you can modify their contents after they have been created. You can change individual elements, add new elements, remove elements, and more. For example:
my_list[2] = 10 # Change the third element to 10
print(my_list) # Output: [1, 2, 10, 4, 5]
my_list.append(6) # Add a new element to the end of the list
print(my_list) # Output: [1, 2, 10, 4, 5, 6]
my_list.pop(1) # Remove the second element from the list
print(my_list) # Output: [1, 10, 4, 5, 6]
Iterating Over Lists
Python lists are iterable, which means you can easily loop over them using a for loop. This allows you to perform operations on each element in the list. For example:
for item in my_list:
print(item)
List Comprehensions
Python lists also support list comprehensions, which provide a concise and powerful way to create lists based on existing lists. List comprehensions allow you to apply a function or condition to each element in a list and create a new list based on the results. For example:
squared_list = [x**2 for x in my_list]
print(squared_list)
Filtering Lists
You can use list comprehensions to filter elements in a list based on a condition. This allows you to create a new list that contains only the elements that meet the specified criteria. For example:
even_numbers = [x for x in my_list if x % 2 == 0]
print(even_numbers)
Sorting Lists
Python lists have a built-in sort() method that allows you to sort the elements in a list in ascending order. You can also specify a reverse=True argument to sort the list in descending order. For example:
my_list.sort()
print(my_list)
my_list.sort(reverse=True)
print(my_list)
Conclusion
Python lists are a powerful and versatile data structure that can be used in a wide variety of programming tasks. By mastering the various features and capabilities of Python lists, you can unleash their full potential and greatly enhance your programming skills. Experiment with the examples provided in this guide and explore the many ways in which you can leverage the power of Python lists in your projects.