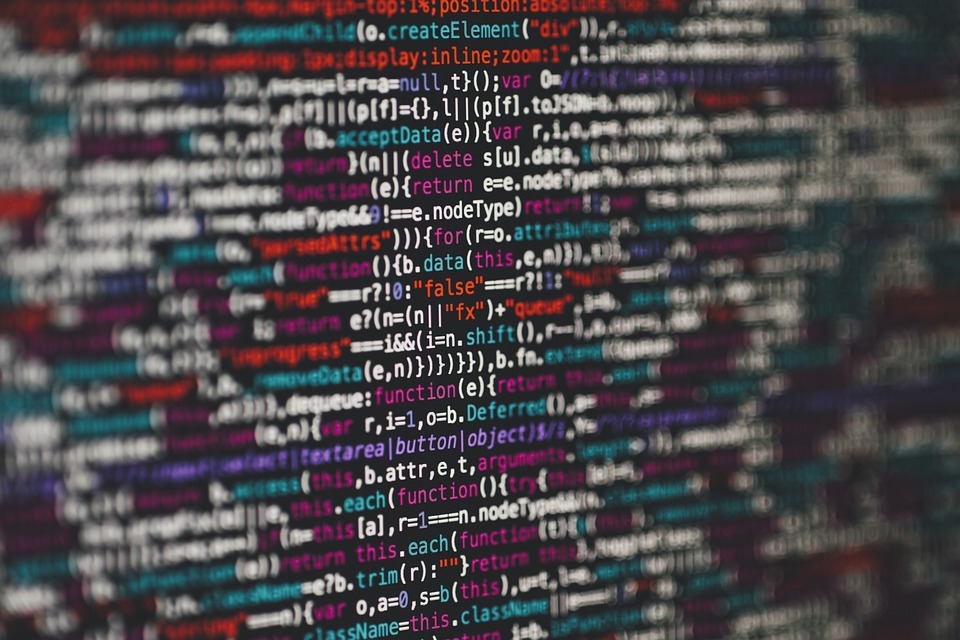
JavaScript arrays are a powerful data structure that allows developers to store and manipulate collections of data. With their flexibility and versatility, arrays are a fundamental part of any JavaScript programmer’s toolkit. In this article, we will explore some tips and tricks for working with JavaScript arrays to unleash their full potential.
1. Initializing an Array:
To create a new array in JavaScript, you can use the array literal syntax, which uses square brackets to enclose a list of elements. For example:
“`javascript
let colors = [‘red’, ‘blue’, ‘green’];
“`
You can also use the Array constructor to create an empty array or an array with a specific length. For example:
“`javascript
let numbers = new Array(5); // creates an array with a length of 5
“`
2. Accessing Array Elements:
You can access individual elements of an array by using square bracket notation with the index of the element you want to access. Remember that arrays in JavaScript are zero-indexed, meaning that the first element is at index 0. For example:
“`javascript
let colors = [‘red’, ‘blue’, ‘green’];
console.log(colors[0]); // prints ‘red’
“`
3. Adding and Removing Elements:
Arrays in JavaScript are dynamic, meaning you can add or remove elements from them at any time. To add elements to the end of an array, you can use the `push()` method. For example:
“`javascript
let fruits = [‘apple’, ‘banana’];
fruits.push(‘orange’);
console.log(fruits); // prints [‘apple’, ‘banana’, ‘orange’]
“`
To remove elements from the end of an array, you can use the `pop()` method. For example:
“`javascript
let fruits = [‘apple’, ‘banana’, ‘orange’];
fruits.pop();
console.log(fruits); // prints [‘apple’, ‘banana’]
“`
4. Iterating over Arrays:
There are several ways to iterate over the elements of an array in JavaScript. One common method is to use a `for` loop with the array’s `length` property. For example:
“`javascript
let numbers = [1, 2, 3, 4, 5];
for (let i = 0; i