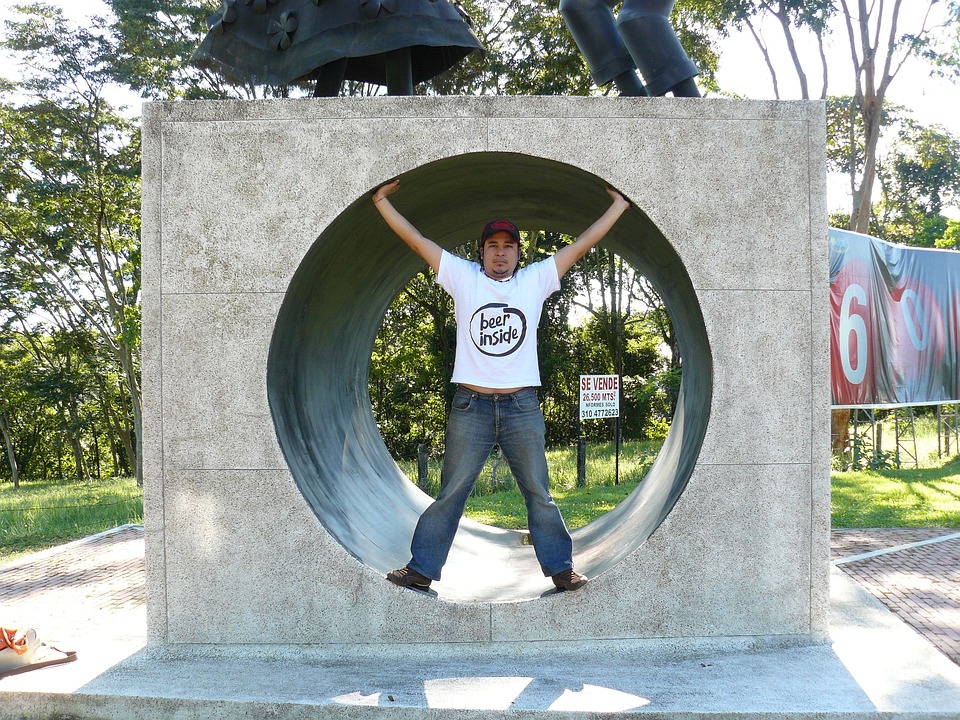
Python list comprehensions are a powerful and concise way to create lists in Python. They allow you to create lists in a single line of code, making your code more readable and efficient. In this article, we will explore the ins and outs of Python list comprehensions and how to use them effectively in your code.
List comprehensions in Python are a syntactic construct that allows you to create lists based on existing lists or other iterable objects. The basic syntax of a list comprehension is as follows:
“` python
new_list = [expression for item in iterable if condition]
“`
In this syntax, the expression is the value that will be added to the new list, the item is the element from the iterable that will be used to create the new list, and the condition is an optional filter that can be used to include only certain elements in the new list.
For example, let’s say we have a list of numbers and we want to create a new list that contains only the even numbers from the original list. We can use a list comprehension to achieve this in a single line of code:
“` python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [num for num in numbers if num % 2 == 0]
print(even_numbers)
“`
This will output `[2, 4, 6, 8, 10]`, which is the new list containing only the even numbers from the original list.
List comprehensions can also be nested, allowing you to create more complex lists. For example, let’s say we have a list of lists and we want to flatten it into a single list. We can use a nested list comprehension to achieve this:
“` python
nested_lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened_list = [num for sublist in nested_lists for num in sublist]
print(flattened_list)
“`
This will output `[1, 2, 3, 4, 5, 6, 7, 8, 9]`, which is the flattened list created from the original list of lists.
List comprehensions are not only concise and readable, but they are also more efficient than traditional for loops. They are faster because they are implemented in C under the hood, whereas traditional for loops are implemented in Python. This makes list comprehensions a great choice for performance-critical code.
In conclusion, Python list comprehensions are a powerful tool for creating lists in a concise and efficient way. By understanding the syntax and how to effectively use list comprehensions, you can make your code more readable and maintainable. Whether you are filtering elements from a list, flattening a list of lists, or creating a new list based on existing lists, list comprehensions can help you achieve your goals with minimal code.