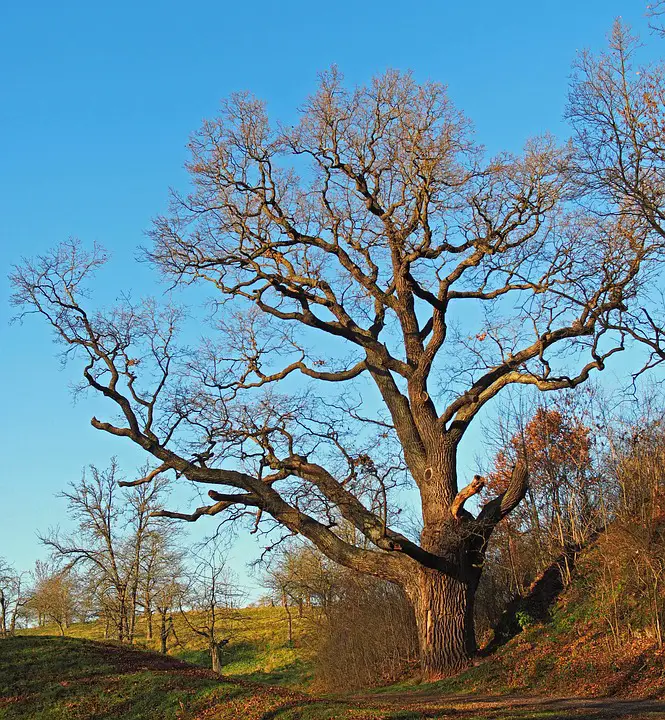
Python is a versatile and powerful programming language that is widely used in various industries, from web development to data science. One of the key features of Python is its support for object-oriented programming, which allows developers to create reusable and modular code through the use of classes and objects.
In this beginner’s guide, we will explore the fundamentals of Python classes and how they can be used to create more organized and efficient code.
What is a Class in Python?
In Python, a class is a blueprint for creating objects. It defines the properties and behavior of objects that belong to that class. For example, if we were creating a class for a car, we might define properties such as make, model, and color, and behaviors such as starting the engine and accelerating.
To create a class in Python, we use the class keyword followed by the name of the class. Here is an example of a simple class in Python:
“` python
class Car:
def __init__(self, make, model, color):
self.make = make
self.model = model
self.color = color
def start_engine(self):
print(“Engine started”)
def accelerate(self):
print(“Accelerating”)
“`
In this example, we have defined a class called Car with three properties (make, model, and color) and two methods (start_engine and accelerate). The __init__ method is a special method in Python that is called when a new object of the class is created. It is used to initialize the properties of the object.
Creating Objects from a Class
Once we have defined a class, we can create objects (instances) of that class. To create an object, we use the class name followed by parentheses. We can then access the properties and methods of the object using dot notation. Here is an example of creating an object from the Car class:
“` python
my_car = Car(“Toyota”, “Camry”, “red”)
print(my_car.make) # Output: Toyota
print(my_car.color) # Output: red
my_car.start_engine() # Output: Engine started
my_car.accelerate() # Output: Accelerating
“`
In this example, we have created an object called my_car from the Car class and accessed its properties and methods.
Inheritance and Polymorphism
One of the key features of object-oriented programming is inheritance, which allows us to create a new class based on an existing class. The new class inherits the properties and methods of the existing class and can also define its own properties and methods. This promotes code reuse and allows for more modular and organized code.
Polymorphism is another important concept in object-oriented programming, which allows objects of different classes to be treated as objects of a common superclass. This enables flexibility and extensibility in our code.
Conclusion
Understanding the fundamentals of Python classes is essential for anyone looking to become proficient in object-oriented programming. By creating classes and objects, we can create more organized and efficient code that is easier to maintain and extend. Inheritance and polymorphism further enhance the power and flexibility of object-oriented programming in Python. By mastering these concepts, developers can build robust and scalable applications in Python.