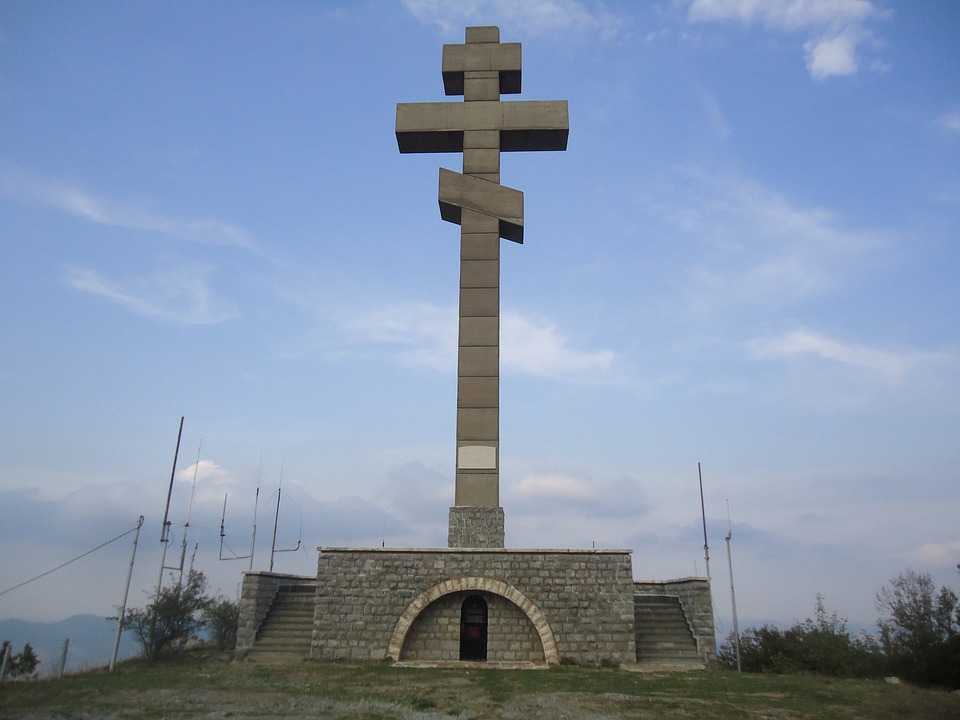
Python lists are a fundamental data structure in Python programming, allowing you to store and manipulate collections of data. However, efficiently manipulating lists can be a challenge, especially when dealing with large data sets. In this article, we will discuss some top tips for efficiently manipulating Python lists.
1. Use list comprehensions: List comprehensions are a concise and efficient way to create lists in Python. Instead of using traditional for loops, list comprehensions allow you to create lists in a single line of code. For example, instead of writing:
“`
result = []
for i in range(10):
result.append(i)
“`
You can use a list comprehension:
“`
result = [i for i in range(10)]
“`
List comprehensions are not only more readable but also more efficient as they are optimized for speed.
2. Avoid unnecessary copying: When manipulating lists, it is important to avoid unnecessary copying of lists. For example, if you need to modify a list in place, use the `extend()` method instead of concatenating lists with the `+` operator. The `extend()` method modifies the original list, while concatenating lists creates a new list.
3. Use built-in functions: Python provides a variety of built-in functions for manipulating lists, such as `map()`, `filter()`, and `reduce()`. These functions can help you perform common list operations more efficiently and concisely.
4. Use the `in` operator for membership testing: When checking if an element is present in a list, use the `in` operator instead of iterating over the list manually. The `in` operator is optimized for speed and will give you a more efficient solution.
5. Use slicing for subsetting lists: Slicing allows you to extract a subset of a list efficiently. Instead of using a for loop to iterate over a list and extract specific elements, you can use slicing to extract elements in a single line of code. For example, to extract the first three elements of a list, you can use `list[:3]`.
6. Use the `sorted()` function for sorting lists: When sorting lists, use the `sorted()` function instead of the `sort()` method. The `sorted()` function returns a new sorted list, leaving the original list unchanged. This can be useful when you need to preserve the original order of the list.
In conclusion, efficiently manipulating Python lists is essential for writing efficient and readable code. By following these top tips, you can improve the performance of your Python programs and make your code more maintainable. Practice these tips in your Python projects to become a more proficient Python programmer.