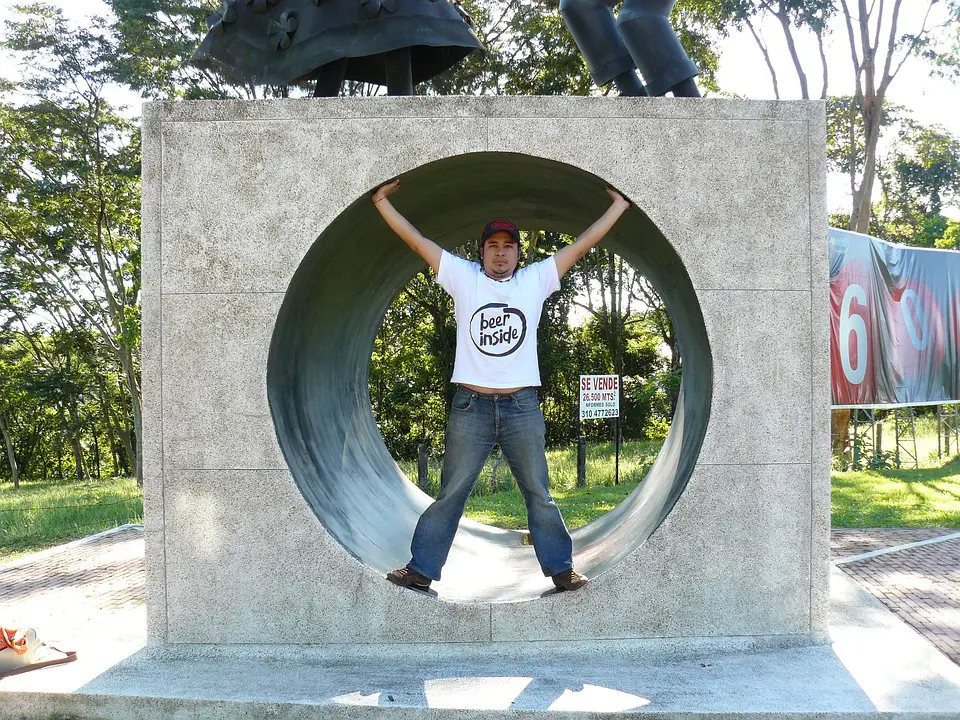
Python lists are a fundamental data structure in Python programming. They are used to store collections of items, such as integers, strings, or objects. Working with lists efficiently is crucial for writing clean and concise code. In this article, we will discuss the top 10 tips and tricks for working with Python lists.
1. Use list comprehension: List comprehension is a concise way to create lists in Python. It allows you to write compact and readable code to iterate over a sequence and apply an operation to each element. For example, you can create a list of squares of numbers from 1 to 10 using list comprehension: squares = [x**2 for x in range(1, 11)]
2. Use slicing: Slicing allows you to extract a subset of elements from a list. You can use slicing to get a specific range of elements from a list, or to reverse a list. For example, to reverse a list, you can use the following syntax: reversed_list = original_list[::-1]
3. Use the append() method: The append() method is used to add elements to the end of a list. It is a convenient way to add new items to a list. For example, you can add a new element to a list using the append() method: numbers = [1, 2, 3] numbers.append(4)
4. Use the extend() method: The extend() method is used to add multiple elements to a list. It is similar to the append() method, but it takes a list as an argument and adds all elements of the list to the original list. For example, you can add multiple elements to a list using the extend() method: numbers = [1, 2, 3] numbers.extend([4, 5, 6])
5. Use the pop() method: The pop() method is used to remove and return the last element from a list. It is a convenient way to remove elements from a list. For example, you can remove the last element from a list using the pop() method: numbers = [1, 2, 3] last_element = numbers.pop()
6. Use the index() method: The index() method is used to find the index of a specific element in a list. It returns the index of the first occurrence of the element in the list. For example, you can find the index of a specific element in a list using the index() method: numbers = [1, 2, 3] index = numbers.index(2)
7. Use the count() method: The count() method is used to count the number of occurrences of a specific element in a list. It returns the number of times the element appears in the list. For example, you can count the number of occurrences of a specific element in a list using the count() method: numbers = [1, 2, 2, 3] count = numbers.count(2)
8. Use the sort() method: The sort() method is used to sort the elements of a list in ascending order. It modifies the original list in place. For example, you can sort a list of numbers using the sort() method: numbers = [3, 1, 2] numbers.sort()
9. Use the reverse() method: The reverse() method is used to reverse the order of elements in a list. It modifies the original list in place. For example, you can reverse a list of numbers using the reverse() method: numbers = [1, 2, 3] numbers.reverse()
10. Use the copy() method: The copy() method is used to create a shallow copy of a list. It creates a new list with the same elements as the original list. For example, you can create a copy of a list using the copy() method: numbers = [1, 2, 3] copied_numbers = numbers.copy()
In conclusion, these tips and tricks will help you work more efficiently with Python lists. By using list comprehension, slicing, and built-in list methods such as append(), extend(), pop(), index(), count(), sort(), reverse(), and copy(), you can manipulate lists in Python effectively. Mastering these techniques will make you a more proficient Python programmer.