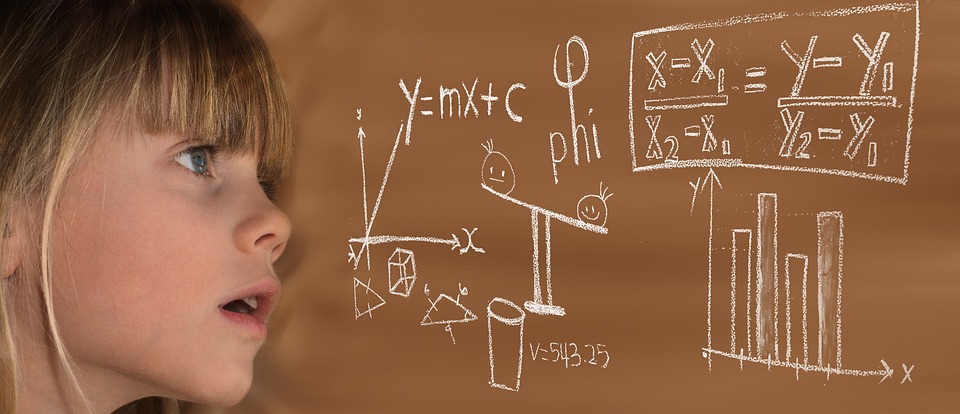
Python’s split() function is a powerful tool for efficiently manipulating strings. By using split(), you can easily break a string into smaller parts based on a specified delimiter. This can be incredibly useful for tasks such as parsing data, extracting information from text, and cleaning up messy strings.
In this ultimate guide, we will explore the various ways you can use split() to manipulate strings in Python effectively.
1. Basic Usage:
The basic usage of split() is simple. You just need to call the split() function on a string and pass in the delimiter you want to split the string by. For example:
“` python
string = “hello world”
parts = string.split(” “)
print(parts)
“`
This will output:
[‘hello’, ‘world’]2. Splitting by Multiple Delimiters:
You can also split a string by multiple delimiters by passing in a list of delimiters to the split() function. For example:
“` python
string = “apple,orange;banana”
parts = string.split(“,;”)
print(parts)
“`
This will output:
[‘apple’, ‘orange’, ‘banana’]3. Limiting the Number of Splits:
You can also limit the number of splits that split() will perform by passing in a second argument to the function. For example:
“` python
string = “one,two,three,four,five”
parts = string.split(“,”, 2)
print(parts)
“`
This will output:
[‘one’, ‘two’, ‘three,four,five’]4. Stripping Whitespace:
By default, split() will split a string based on whitespace. If you want to strip leading and trailing whitespace from each part, you can use the strip() function in combination with split(). For example:
“` python
string = ” hello world “
parts = [p.strip() for p in string.split()]
print(parts)
“`
This will output:
[‘hello’, ‘world’]5. Splitting Lines from a File:
You can also use split() to split lines from a file by reading the file line by line and splitting each line. For example:
“` python
with open(“file.txt”) as file:
lines = [line.strip() for line in file]
parts = [line.split(“,”) for line in lines]
print(parts)
“`
In conclusion, Python’s split() function is a versatile tool for efficiently manipulating strings. By mastering the various ways you can use split(), you can easily parse, extract, and clean up strings in your Python programs. So next time you need to manipulate a string, remember to reach for split() for a quick and efficient solution.