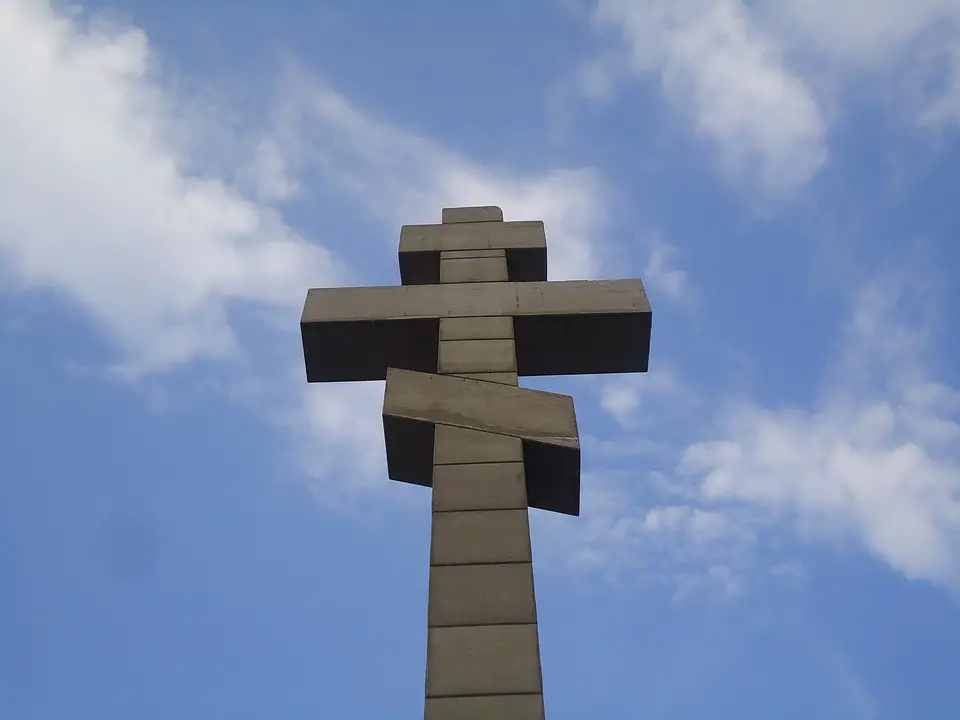
Python is a versatile and powerful programming language that is used by developers for a wide range of applications. One of the key features of Python is its ability to create and manipulate classes, which are essential for organizing and structuring code in a clear and efficient way. In this article, we will explore the ins and outs of Python classes, covering everything you need to know to effectively use them in your programs.
What is a Class in Python?
In Python, a class is a blueprint for creating objects. Objects are instances of a class, and they can have attributes (variables) and methods (functions) that define their behavior. Classes provide a way to organize code and encapsulate data and functionality in a logical and structured manner.
To define a class in Python, you use the `class` keyword followed by the class name and a colon. Here is an example of a simple class definition in Python:
“` python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f”Hello, my name is {self.name} and I am {self.age} years old.”)
“`
In this example, we have defined a `Person` class with two attributes (`name` and `age`) and a method (`greet`) that prints a message with the person’s name and age.
Creating Objects from a Class
Once you have defined a class, you can create objects (instances) of that class by calling the class name followed by parentheses. For example, to create a `Person` object, you would write:
“` python
person1 = Person(“Alice”, 30)
person2 = Person(“Bob”, 25)
“`
In this example, we have created two `Person` objects, `person1` and `person2`, with different values for the `name` and `age` attributes.
Accessing Attributes and Calling Methods
You can access the attributes of an object using dot notation. For example, to access the `name` attribute of `person1`, you would write:
“` python
print(person1.name)
“`
Similarly, you can call methods on an object using dot notation. For example, to call the `greet` method on `person1`, you would write:
“` python
person1.greet()
“`
Inheritance and Polymorphism
Python supports inheritance, which allows you to create new classes that inherit attributes and methods from existing classes. This can help you avoid duplicating code and make your programs more modular and maintainable.
To create a subclass that inherits from a superclass, you simply include the superclass name in parentheses after the subclass name in the class definition. For example, if you wanted to create a `Student` class that inherits from the `Person` class, you would write:
“` python
class Student(Person):
def __init__(self, name, age, student_id):
super().__init__(name, age)
self.student_id = student_id
def study(self, subject):
print(f”{self.name} is studying {subject}.”)
“`
In this example, the `Student` class inherits the `name` and `age` attributes and the `greet` method from the `Person` class. It also defines a new attribute (`student_id`) and a new method (`study`).
Polymorphism is another important concept in object-oriented programming that allows objects of different classes to be treated as objects of a common superclass. This can be useful for writing flexible and reusable code that can work with different types of objects.
Conclusion
Python classes are a powerful tool for organizing and structuring code in a clear and efficient way. By defining classes, creating objects, and using inheritance and polymorphism, you can write more modular, maintainable, and flexible programs. Understanding the ins and outs of Python classes is essential for any developer looking to take their coding skills to the next level. With practice and experimentation, you can leverage the full potential of Python classes in your projects.