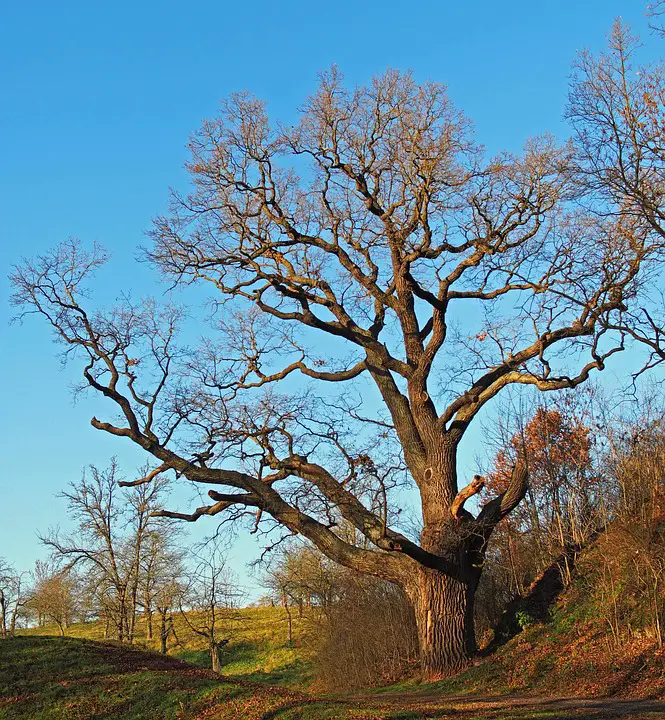
Python is a powerful programming language that is widely used for various applications, from web development to data analysis and machine learning. One of the key features of Python is its support for object-oriented programming, which allows developers to create classes and objects to represent real-world entities in their code. In this article, we will take a deep dive into Python classes and explore their ins and outs.
What is a Class in Python?
In Python, a class is a blueprint for creating objects. It defines the properties and behaviors of objects of a certain type. For example, if you were building a game, you might have a class called “Player” that defines the attributes of a player, such as their name, health, and score, as well as methods for moving, attacking, and interacting with the game world.
To create a class in Python, you use the `class` keyword followed by the class name, and then a colon. Inside the class definition, you can define attributes (variables) and methods (functions) that belong to the class. For example:
“`
class Player:
def __init__(self, name, health, score):
self.name = name
self.health = health
self.score = score
def move(self, direction):
# code to move the player in the specified direction
pass
def attack(self, target):
# code to attack the specified target
pass
“`
In this example, we have defined a `Player` class with attributes for the player’s name, health, and score, as well as methods for moving and attacking.
Creating Objects from Classes
Once you have defined a class in Python, you can create objects (instances) of that class by calling the class name as if it were a function, passing any required arguments to the class’s `__init__` method. For example:
“`
player1 = Player(“Alice”, 100, 0)
player2 = Player(“Bob”, 80, 0)
“`
In this example, we have created two `Player` objects, `player1` and `player2`, with different names and health values.
Accessing Attributes and Calling Methods
Once you have created objects from a class, you can access their attributes and call their methods using dot notation. For example:
“`
print(player1.name) # output: “Alice”
print(player2.health) # output: 80
player1.move(“up”)
player2.attack(player1)
“`
In this example, we are accessing the `name` and `health` attributes of the `player1` and `player2` objects, and calling the `move` and `attack` methods on them.
Inheritance and Polymorphism
One of the key features of object-oriented programming is inheritance, which allows you to create new classes based on existing classes. In Python, you can create a subclass that inherits from a superclass by passing the superclass name in parentheses after the subclass name. For example:
“`
class Mage(Player):
def cast_spell(self, target):
# code to cast a spell on the specified target
pass
“`
In this example, we have created a `Mage` subclass of the `Player` class, which adds a `cast_spell` method to the class.
Polymorphism is another important concept in object-oriented programming, which allows objects of different classes to be treated as objects of a common superclass. This can be useful for writing code that can work with a variety of different types of objects without needing to know their specific types. For example:
“`
def do_action(player):
player.move(“left”)
player1 = Player(“Alice”, 100, 0)
mage1 = Mage(“Merlin”, 80, 0)
do_action(player1)
do_action(mage1)
“`
In this example, we have defined a `do_action` function that takes a `Player` object as an argument and calls its `move` method. We can pass both a `Player` object and a `Mage` object to this function, demonstrating polymorphism.
Conclusion
In this article, we have explored the ins and outs of Python classes, including how to define classes, create objects from classes, access attributes and call methods on objects, and use inheritance and polymorphism to create more complex and flexible code. Object-oriented programming is a powerful paradigm that can help you organize and structure your code in a more intuitive and reusable way, and Python’s support for classes and objects makes it easy to implement. By mastering Python classes, you can take your programming skills to the next level and build more sophisticated and maintainable applications.