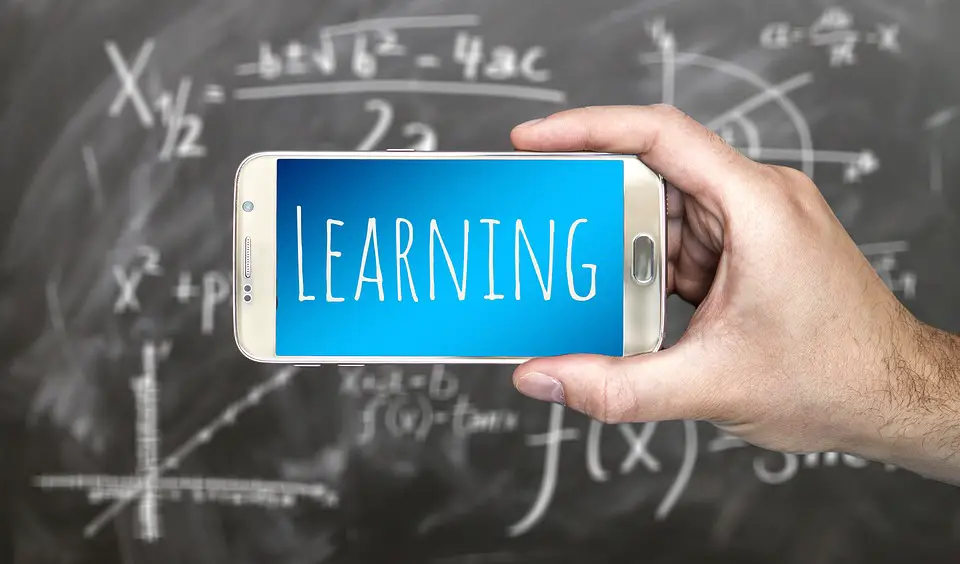
Python’s split() method is a powerful tool for breaking up strings into substrings based on a specified delimiter. Most Python developers are familiar with the basic usage of split(), but did you know that there are hidden features of split() that you may not be aware of?
One hidden feature of split() is the ability to limit the number of splits that are performed. By providing an optional second argument to split(), you can specify the maximum number of splits to make. For example, if you only want to split a string into two substrings, you can do so like this:
“` python
s = “apple,banana,orange,grape”
parts = s.split(“,”, 2)
print(parts)
“`
This will output:
“`
[‘apple’, ‘banana’, ‘orange,grape’]“`
Another hidden feature of split() is the ability to specify multiple delimiters. By providing a list of delimiters as the argument to split(), you can split a string based on any of the delimiters in the list. For example:
“` python
s = “apple,banana;orange|grape”
delimiters = [“,”, “;”, “|”]
parts = s.split(delimiters)
print(parts)
“`
This will output:
“`
[‘apple’, ‘banana’, ‘orange’, ‘grape’]“`
You can also use split() in conjunction with other string methods to achieve more complex splitting behavior. For example, you can use split() in combination with strip() to remove leading and trailing whitespace before splitting a string:
“` python
s = ” apple,banana,orange,grape “
parts = s.strip().split(“,”)
print(parts)
“`
This will output:
“`
[‘apple’, ‘banana’, ‘orange’, ‘grape’]“`
In addition, split() can be used in combination with list comprehension to perform more advanced splitting operations. For example, you can split a string into substrings and then convert each substring to uppercase in a single line of code like this:
“` python
s = “apple,banana,orange,grape”
parts = [part.upper() for part in s.split(“,”)]
print(parts)
“`
This will output:
“`
[‘APPLE’, ‘BANANA’, ‘ORANGE’, ‘GRAPE’]“`
These hidden features of split() can help you write cleaner and more efficient code when working with strings in Python. By taking advantage of these features, you can make your code more concise and readable while still achieving the desired splitting behavior. So next time you need to split a string in Python, remember to explore the hidden features of split() to see how they can improve your code.