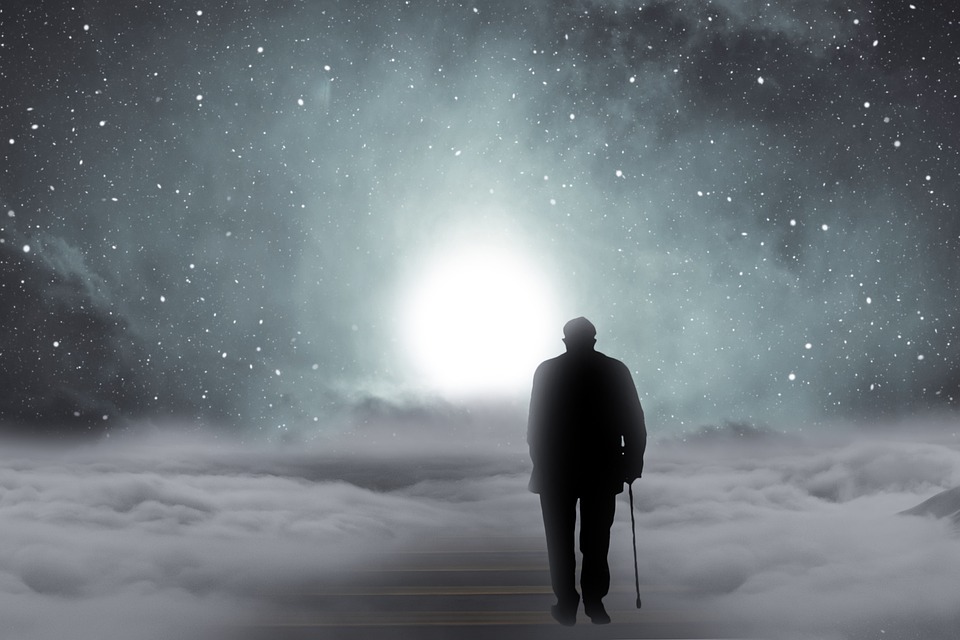
As a programming language created by Google, Go has gained popularity among developers for its simplicity, efficiency, and ease of use. With its built-in features for concurrency, garbage collection, and static typing, Go is a powerful tool for solving a wide range of programming problems. In this article, we will explore some common programming problems and demonstrate how Go can be used to solve them with real-life examples.
1. Parsing JSON data
One common task in programming is parsing JSON data to extract specific information. Go provides a simple and efficient way to work with JSON data using its encoding/json package. Let’s consider an example where we have a JSON file containing information about employees, and we want to extract the names and ages of all employees.
“`go
package main
import (
“encoding/json”
“fmt”
“os”
)
type Employee struct {
Name string `json:”name”`
Age int `json:”age”`
}
func main() {
file, err := os.Open(“employees.json”)
if err != nil {
fmt.Println(“Error opening file:”, err)
return
}
defer file.Close()
var employees []Employee
decoder := json.NewDecoder(file)
err = decoder.Decode(&employees)
if err != nil {
fmt.Println(“Error decoding JSON:”, err)
return
}
for _, emp := range employees {
fmt.Printf(“Name: %s, Age: %d\n”, emp.Name, emp.Age)
}
}
“`
2. Implementing a REST API
Another common programming task is building a REST API to expose data and functionality to other applications. Go makes it easy to create robust and performant APIs using its built-in net/http package. Let’s consider an example where we create a simple REST API to manage a list of books.
“`go
package main
import (
“encoding/json”
“fmt”
“net/http”
)
type Book struct {
ID int `json:”id”`
Title string `json:”title”`
Author string `json:”author”`
}
var books []Book
func getBooks(w http.ResponseWriter, r *http.Request) {
w.Header().Set(“Content-Type”, “application/json”)
json.NewEncoder(w).Encode(books)
}
func main() {
books = append(books, Book{ID: 1, Title: “Go Programming”, Author: “John Doe”})
http.HandleFunc(“/books”, getBooks)
fmt.Println(“Server running on port 8080”)
http.ListenAndServe(“:8080”, nil)
}
“`
3. Performing concurrent tasks
Concurrency is a key feature of Go that allows developers to execute multiple tasks concurrently, improving performance and efficiency. Let’s consider an example where we want to fetch data from multiple URLs concurrently using goroutines.
“`go
package main
import (
“fmt”
“net/http”
“sync”
)
func fetchURL(url string, wg *sync.WaitGroup) {
defer wg.Done()
resp, err := http.Get(url)
if err != nil {
fmt.Println(“Error fetching URL:”, err)
return
}
defer resp.Body.Close()
fmt.Printf(“Fetched %s\n”, url)
}
func main() {
urls := []string{“https://www.google.com”, “https://www.github.com”, “https://www.medium.com”}
var wg sync.WaitGroup
for _, url := range urls {
wg.Add(1)
go fetchURL(url, &wg)
}
wg.Wait()
fmt.Println(“All URLs fetched”)
}
“`
In this article, we have explored how Go can be used to solve common programming problems such as parsing JSON data, implementing a REST API, and performing concurrent tasks. By leveraging the features and simplicity of Go, developers can build efficient and reliable solutions for a wide range of programming challenges. Whether you are a beginner or experienced developer, Go is a versatile language that can help you tackle real-life programming problems with ease.