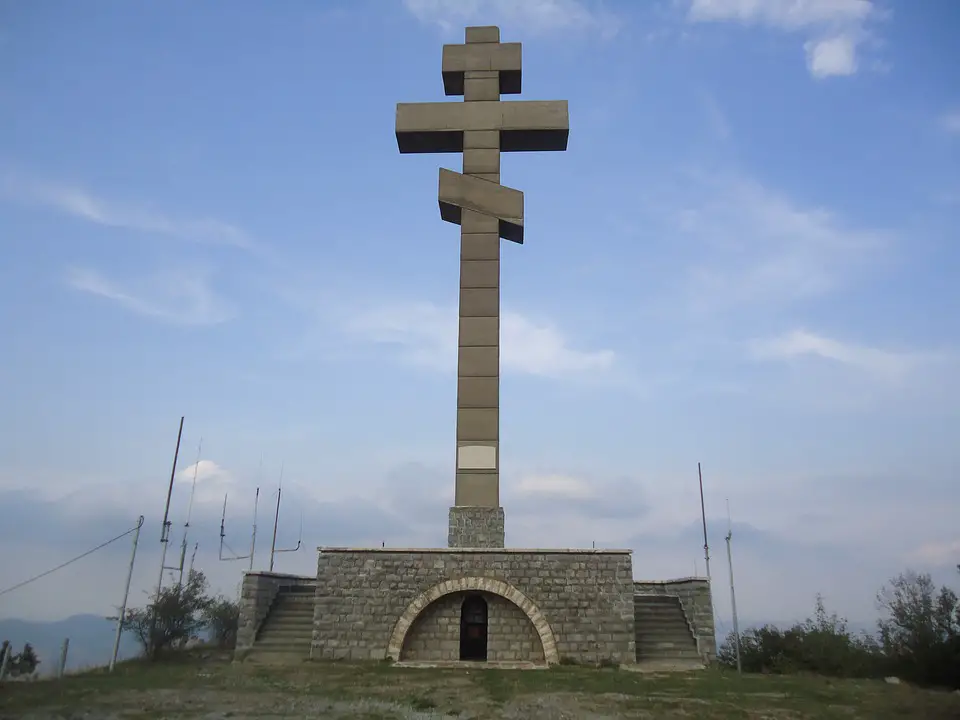
When it comes to writing clean and efficient code, one of the most important principles to follow is simplicity. The more complex your code is, the harder it is to debug, maintain, and understand. This is where Python’s split method comes in handy.
The split method is a built-in function in Python that allows you to split a string into a list based on a specified delimiter. This can be incredibly useful for parsing data, extracting information, and cleaning up messy strings. In this article, we will discuss some best practices for beginners to simplify their code using Python’s split method.
1. Use the split method to tokenize strings: One common use case for the split method is tokenizing strings. For example, if you have a sentence that you want to break down into individual words, you can use the split method with the space delimiter like this:
“` python
sentence = “Hello world”
words = sentence.split(” “)
print(words) # Output: [‘Hello’, ‘world’]
“`
2. Specify the delimiter: By default, the split method splits the string based on whitespace. However, you can specify a custom delimiter to split the string based on a specific character or sequence of characters. For example, if you have a comma-separated list of items, you can split it like this:
“` python
items = “apple,banana,orange”
items_list = items.split(“,”)
print(items_list) # Output: [‘apple’, ‘banana’, ‘orange’]
“`
3. Handle empty strings: If your string contains consecutive delimiters, the split method will return empty strings in the resulting list. You can filter out these empty strings using a list comprehension like this:
“` python
data = “1,,3,4”
cleaned_data = [x for x in data.split(“,”) if x]
print(cleaned_data) # Output: [‘1’, ‘3’, ‘4’]
“`
4. Use unpacking to assign variables: If you know the exact number of elements that will be returned by the split method, you can use unpacking to assign the variables directly. This can make your code more concise and readable. For example:
“` python
name, age = “John,30”.split(“,”)
print(name, age) # Output: John 30
“`
5. Consider using regular expressions: While the split method is great for simple cases, it may not be the best choice for complex string manipulation tasks. In such cases, you can use regular expressions to achieve more sophisticated splitting and matching. This can help you handle more complex patterns and edge cases efficiently.
In conclusion, the split method in Python is a powerful tool for simplifying your code and handling string manipulation tasks effectively. By following these best practices for beginners, you can leverage the split method to write cleaner and more readable code. Remember to experiment with different delimiters, handle empty strings gracefully, and consider using regular expressions for more complex scenarios. Happy coding!