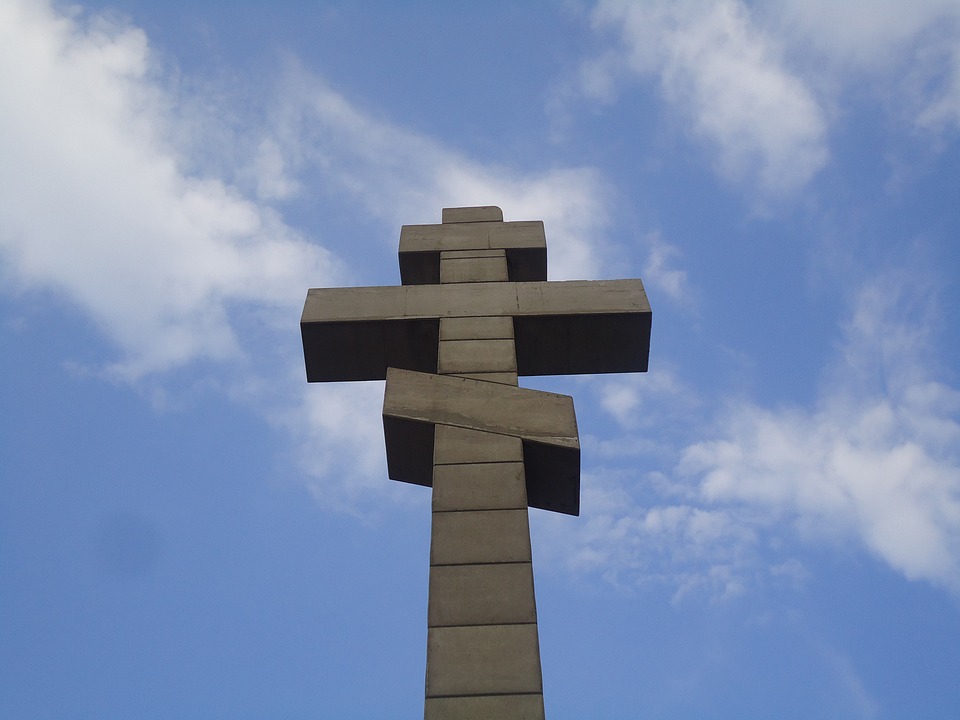
When it comes to writing clean and efficient code, one of the best tools at a programmer’s disposal is the Python split method. This simple yet powerful function allows you to easily break up strings into smaller, more manageable pieces, making your code easier to read and maintain.
In this comprehensive guide, we will explore the ins and outs of using the split method in Python and how it can help you simplify your code.
What is the split method?
The split method is a built-in function in Python that allows you to split a string into a list of substrings based on a specified delimiter. By default, the split method uses whitespace as the delimiter, but you can also specify a different delimiter if needed.
Here is a basic example of how the split method works:
“` python
sentence = “Hello, world!”
words = sentence.split()
print(words)
“`
Output:
“`
[‘Hello,’, ‘world!’]“`
In this example, the split method breaks up the string “Hello, world!” into two substrings, “Hello,” and “world!”, based on the whitespace delimiter.
Using a custom delimiter
In addition to using whitespace as the default delimiter, you can also specify a custom delimiter when using the split method. This is useful when you need to split a string based on a specific character or sequence of characters.
“` python
numbers = “1,2,3,4,5”
numbers_list = numbers.split(‘,’)
print(numbers_list)
“`
Output:
“`
[‘1’, ‘2’, ‘3’, ‘4’, ‘5’]“`
In this example, the split method splits the string “1,2,3,4,5” into a list of substrings based on the comma delimiter.
Handling multiple delimiters
You can also use the split method to handle multiple delimiters by passing a list of delimiters as an argument. This allows you to split a string based on multiple characters or sequences of characters.
“` python
sentence = “The quick brown fox jumps over the lazy dog”
words = sentence.split([‘ ‘, ‘o’])
print(words)
“`
Output:
“`
[‘The’, ‘quick’, ‘brown’, ‘fx’, ‘jumps’, ‘ver’, ‘the’, ‘lazy’, ‘dg’]“`
In this example, the split method splits the string “The quick brown fox jumps over the lazy dog” into a list of substrings based on the space and ‘o’ delimiters.
Conclusion
The split method in Python is a powerful tool that can help you simplify your code by breaking up strings into smaller, more manageable pieces. By using the split method, you can make your code more readable and maintainable, leading to a more efficient and effective programming experience.
In this comprehensive guide, we have covered the basics of using the split method in Python, as well as some advanced techniques for handling custom delimiters and multiple delimiters. By incorporating the split method into your programming toolkit, you can streamline your code and improve your overall development workflow.