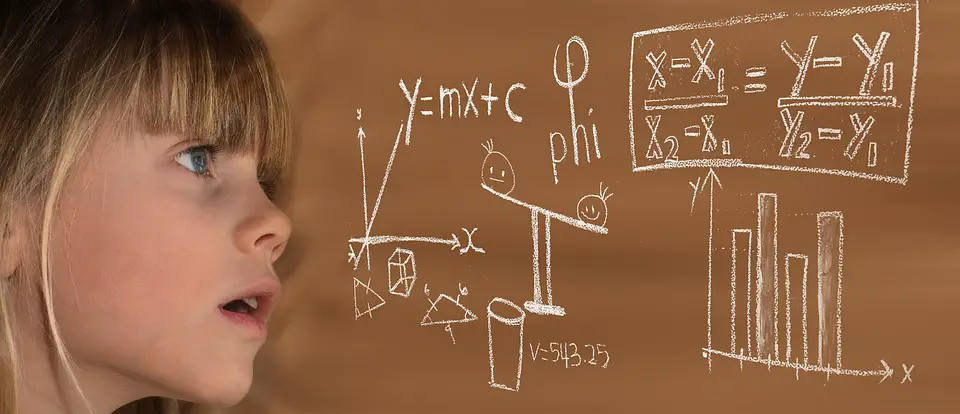
When it comes to writing clean and concise programs, one of the most powerful tools at a programmer’s disposal is the Python map function. This handy function allows you to apply a function to every item in a list or other iterable, making it easy to simplify your code and avoid repetitive loops.
Using map can make your code cleaner and more readable by eliminating the need for cumbersome for loops and conditional statements. Instead of writing out each step of a loop, you can simply define the function you want to apply and pass it to map along with the list or iterable you want to operate on.
For example, let’s say you have a list of numbers and you want to square each one. Instead of writing a loop like this:
“` python
numbers = [1, 2, 3, 4, 5]
squared_numbers = []
for num in numbers:
squared_numbers.append(num ** 2)
“`
You can achieve the same result in a single line of code using map:
“` python
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x ** 2, numbers))
“`
Not only is the second version shorter and easier to read, but it also clearly communicates the intent of the code – to square each number in the list.
In addition to making your code more concise, using map can also make it more efficient. Because map is a built-in function in Python, it is optimized for performance and can often outperform traditional loops in terms of speed and memory usage.
Another benefit of using map is that it encourages a functional programming style, which can lead to more modular and reusable code. By separating the logic of your program into small, self-contained functions that can be easily applied to different inputs, you can create code that is easier to maintain and extend.
Of course, map is just one tool in Python’s arsenal for simplifying code. Other built-in functions like filter and reduce can also be useful for streamlining your programs. And as you become more comfortable with functional programming concepts, you may find yourself reaching for these tools more and more often.
In conclusion, if you want to write clean and concise programs in Python, consider using the map function to simplify your code. By applying a function to every item in a list or iterable, you can eliminate the need for repetitive loops and conditional statements, making your code more readable and efficient. Give map a try in your next project and see how it can help you write better code.