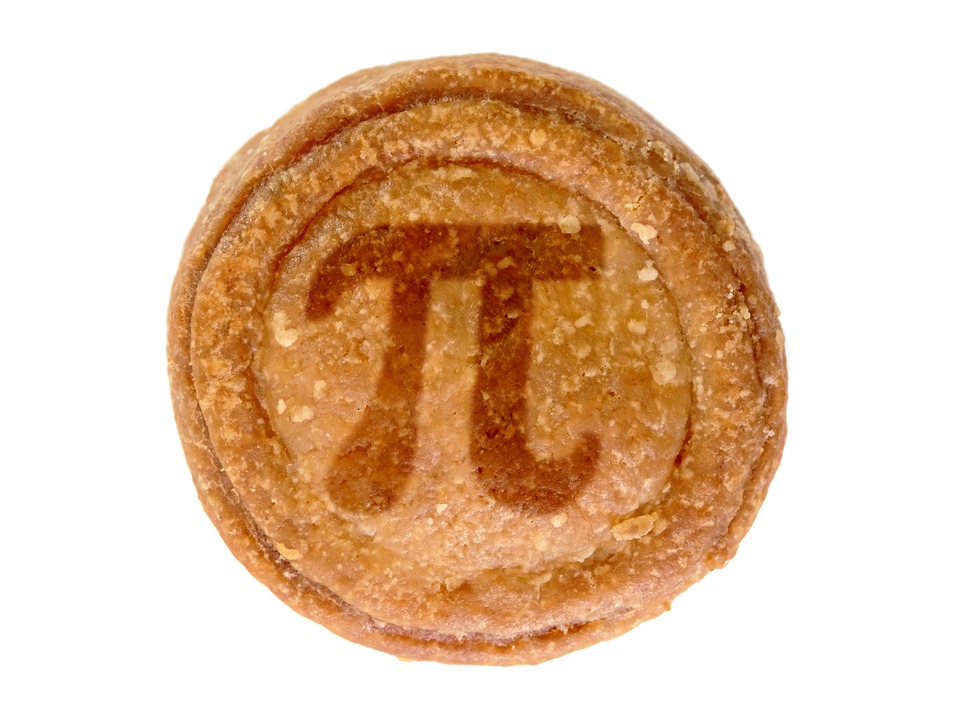
Python sets are a powerful tool that can help you efficiently manage and manipulate data in your code. Sets are similar to lists in that they are a collection of elements, but they have some key differences that make them especially useful in certain situations.
One of the main advantages of using sets in Python is that they do not allow duplicate elements. This means that if you have a list of items and you want to remove any duplicates, you can simply convert the list to a set and then back to a list to eliminate any duplicates. This can be a very useful feature when working with large amounts of data.
Another advantage of sets is that they support set operations such as union, intersection, and difference. These operations allow you to combine sets, find common elements between sets, and find elements that are unique to one set or another. These operations can be very useful when working with complex data structures and can help you quickly and efficiently manipulate your data.
To create a set in Python, you can simply use curly braces and separate the elements with commas. For example, to create a set of numbers, you can write:
my_set = 1, 2, 3, 4, 5
You can also create a set from a list by using the set() function. For example, to create a set from a list of numbers, you can write:
my_list = [1, 2, 3, 4, 5]
my_set = set(my_list)
Once you have created a set, you can use set operations to manipulate the data. For example, to find the union of two sets, you can use the | operator. To find the intersection of two sets, you can use the & operator. And to find the difference between two sets, you can use the – operator.
Sets can be a very powerful tool in Python, but they are not always the best choice for every situation. Sets are unordered collections, so if you need to preserve the order of your elements, you may want to use a list instead. Sets also do not support indexing, so if you need to access elements by their position in the set, a list may be a better choice.
Overall, sets can be a valuable tool in Python for efficiently managing and manipulating data in your code. By understanding how sets work and how to use set operations effectively, you can take advantage of the unique features that sets offer and improve the efficiency and readability of your code.