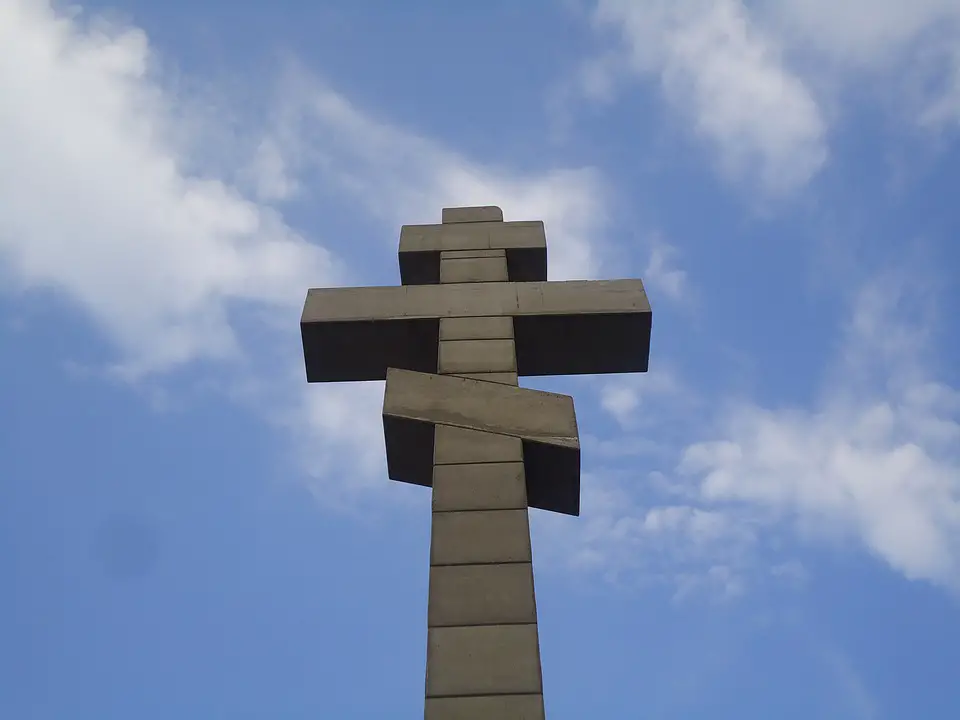
Python is one of the most popular programming languages in the world, known for its simplicity and versatility. One of the key features that sets Python apart from other languages is its powerful data structures, one of which is the set.
Sets in Python are unordered collections of unique elements. This means that a set cannot have duplicate values, and the order in which elements are added to a set is not preserved. Sets are commonly used for mathematical operations such as union, intersection, and difference.
Creating a set in Python is as simple as enclosing a list of elements in curly braces, like this:
“`
my_set = 1, 2, 3, 4, 5
“`
Alternatively, you can create an empty set using the `set()` function:
“`
empty_set = set()
“`
Sets are mutable, meaning that you can add or remove elements from a set using the `add()` and `remove()` methods:
“`
my_set.add(6)
my_set.remove(5)
“`
You can also perform common set operations such as union, intersection, and difference using built-in Python operators:
“`
set1 = 1, 2, 3
set2 = 3, 4, 5
# Union
print(set1 | set2)
# Intersection
print(set1 & set2)
# Difference
print(set1 – set2)
“`
Sets in Python are extremely useful when you need to store a collection of unique elements and perform set operations efficiently. They are particularly handy when working with large datasets where duplicates need to be eliminated.
In conclusion, Python sets are a powerful data structure that allows you to store unique elements and perform set operations with ease. Whether you are a beginner or an experienced Python programmer, understanding how sets work can help you write more efficient and concise code. So next time you need to work with a collection of unique elements, consider using a set in Python.