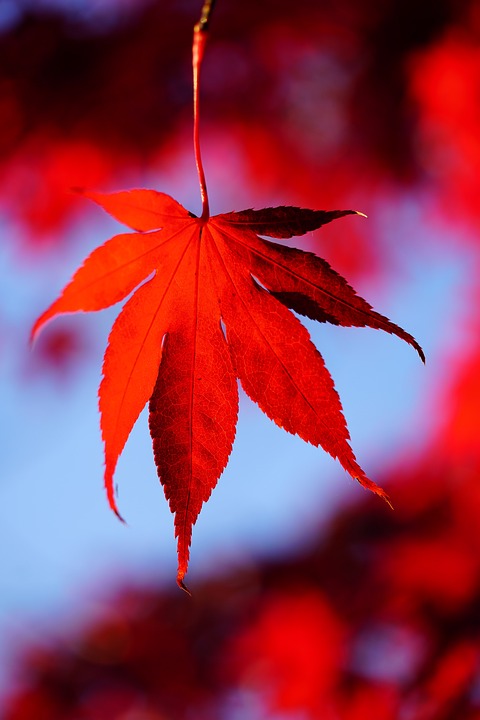
Python Map: A Secret Ingredient for High-Performing Code
In the world of programming, efficiency and performance are key. As developers, we are constantly looking for ways to optimize our code and make it run faster. One powerful tool that can help us achieve this is the Python map function.
The map function is a built-in Python function that applies a given function to each item of an iterable (such as a list or a tuple) and returns a new iterable with the results. It takes two arguments: the function to be applied and the iterable.
One of the main advantages of using the map function is that it allows us to write more concise and readable code. Instead of using a for loop to iterate over each item in a list and apply a function to it, we can simply use the map function. This not only saves us lines of code but also makes our code easier to understand and maintain.
Let’s look at an example to better understand the power of the map function. Suppose we have a list of numbers and we want to calculate their squares. Without using the map function, we would have to write a for loop to iterate over each number and calculate its square. However, with the map function, we can achieve the same result in just one line of code:
“`
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x**2, numbers))
“`
In this example, the lambda function `lambda x: x**2` is applied to each number in the `numbers` list, resulting in a new list `squared_numbers` containing the squares of the original numbers.
Another advantage of using the map function is that it can significantly improve the performance of our code. The map function is implemented in C, which means it is highly optimized and can process large amounts of data very efficiently. By using the map function instead of a for loop, we can often achieve significant speed improvements, especially when dealing with large datasets.
Furthermore, the map function supports parallel processing, which can further enhance the performance of our code. By using multiple processors or cores, the map function can distribute the workload across them, allowing for faster computation. This is particularly useful when working with computationally intensive tasks that can benefit from parallel execution.
It is important to note that the map function returns an iterable, not a list. To obtain a list, we need to convert the result of the map function using the `list()` function, as shown in the previous example. Alternatively, we can use the map function in combination with other built-in functions like `filter()` or `reduce()` for more complex operations.
In conclusion, the Python map function is a secret ingredient for high-performing code. It allows us to write more concise and readable code, improves the performance of our code, and supports parallel processing. By leveraging the power of the map function, we can optimize our code and achieve faster and more efficient results. So the next time you find yourself in need of iterating over a list and applying a function to each item, remember the map function and unlock its potential for high-performing code.