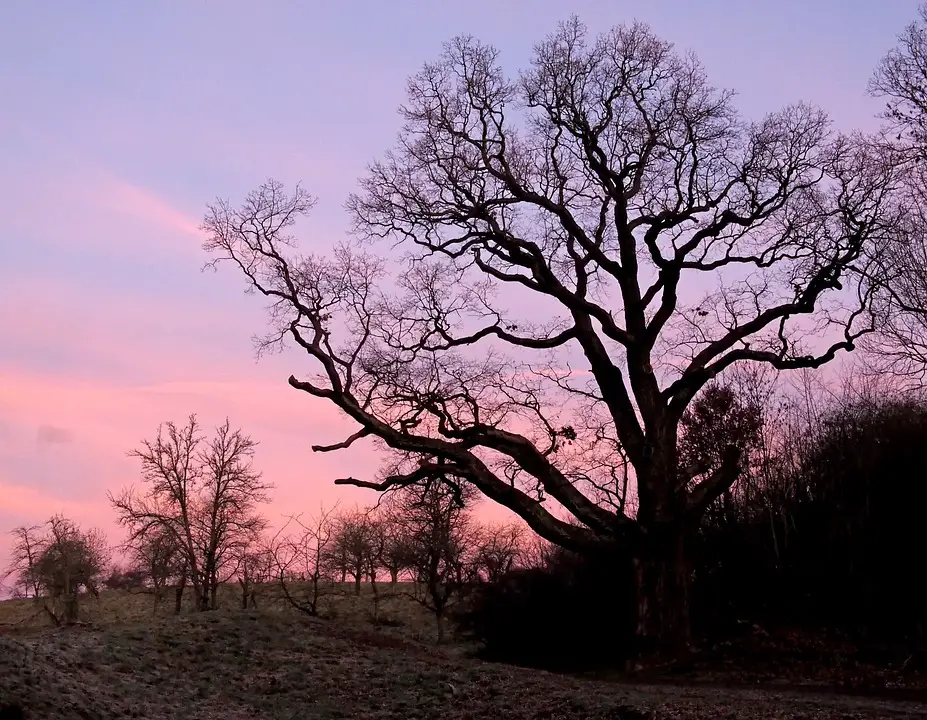
Python list comprehensions are a powerful tool that allows you to simplify your code and make it more concise. They provide a compact and efficient way to create lists in Python by combining loops and conditional statements in a single line.
List comprehensions are a great way to iterate over a sequence, such as a list, and perform operations on each element. They can also be used to filter elements based on certain conditions. This makes them a versatile and efficient tool for data manipulation and transformation.
To create a list comprehension, you start with a set of square brackets [], inside which you write an expression that defines the elements of the new list. This expression can include a loop that iterates over a sequence and an optional conditional statement that filters out elements based on a certain condition.
For example, suppose you have a list of numbers and you want to create a new list that contains only the even numbers. You can do this using a list comprehension like this:
“` python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [x for x in numbers if x % 2 == 0]
“`
In this example, the list comprehension iterates over the numbers list and filters out the elements that are not divisible by 2, resulting in a new list that contains only the even numbers.
List comprehensions can also be nested, allowing you to create more complex data structures in a single line of code. For example, you can create a list of tuples by combining elements from two different lists:
“` python
letters = [‘a’, ‘b’, ‘c’]
numbers = [1, 2, 3]
pairs = [(letter, number) for letter in letters for number in numbers]
“`
In this example, the list comprehension iterates over both the letters and numbers lists, creating a new list of tuples that combine each letter with each number.
List comprehensions are not only more concise than traditional for loops, but they also tend to be more efficient in terms of performance. They are optimized for speed and memory usage, making them a great choice for processing large datasets or performing complex data transformations.
Overall, Python list comprehensions are a powerful feature that can help you simplify your code and make it more readable. By combining loops and conditional statements in a single line, list comprehensions allow you to express complex data manipulations in a concise and efficient way. Whether you are working with lists, tuples, sets, or dictionaries, list comprehensions can help you streamline your code and make it more elegant and efficient.