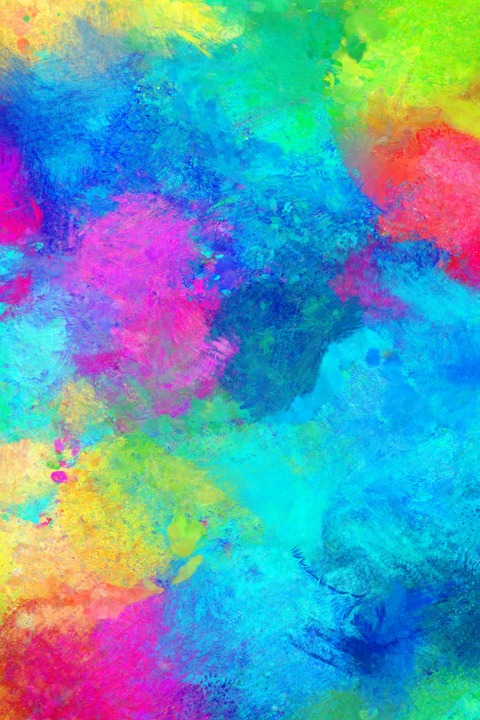
Python Deep Learning: How to Build and Train Neural Networks
Deep learning is a subfield of machine learning that focuses on building and training neural networks to solve complex problems. Python has become the programming language of choice for many deep learning practitioners due to its simplicity and flexibility. In this article, we will discuss how to build and train neural networks using Python.
Building a Neural Network
The first step in building a neural network is to define its architecture. This involves deciding on the number of layers, the number of neurons in each layer, and the activation functions to be used. In Python, this can be done using popular deep learning libraries such as TensorFlow, Keras, or PyTorch.
For example, to create a simple feedforward neural network with two hidden layers using TensorFlow, you can define the model as follows:
“` python
import tensorflow as tf
model = tf.keras.Sequential([
tf.keras.layers.Dense(128, activation=’relu’, input_shape=(784,)),
tf.keras.layers.Dense(64, activation=’relu’),
tf.keras.layers.Dense(10, activation=’softmax’)
])
“`
Training a Neural Network
Once the neural network architecture is defined, the next step is to train the model on a dataset. This involves feeding the input data into the network, computing the output, comparing it with the actual labels, and adjusting the weights of the network to minimize the error.
In Python, this can be done using the `fit` method provided by deep learning libraries. For example, to train the model defined above on the MNIST dataset, you can use the following code:
“` python
model.compile(optimizer=’adam’, loss=’sparse_categorical_crossentropy’, metrics=[‘accuracy’])
model.fit(x_train, y_train, epochs=10, batch_size=32, validation_data=(x_valid, y_valid))
“`
In this code snippet, `x_train` and `y_train` are the input features and labels of the training dataset, while `x_valid` and `y_valid` are the input features and labels of the validation dataset. The `fit` method trains the model for 10 epochs with a batch size of 32, using the Adam optimizer and sparse categorical crossentropy loss.
Evaluating a Neural Network
After training the neural network, it is important to evaluate its performance on a separate test dataset to assess its generalization ability. In Python, this can be done using the `evaluate` method provided by deep learning libraries. For example, to evaluate the model defined above on the test dataset, you can use the following code:
“` python
test_loss, test_accuracy = model.evaluate(x_test, y_test)
print(f’Test loss: {test_loss}, Test accuracy: {test_accuracy}’)
“`
In this code snippet, `x_test` and `y_test` are the input features and labels of the test dataset. The `evaluate` method computes the loss and accuracy of the model on the test dataset and prints the results.
Conclusion
In this article, we have discussed how to build and train neural networks using Python. By following these steps, you can easily create and train deep learning models to solve a wide range of complex problems. Deep learning has become an essential tool in the field of artificial intelligence, and Python provides a powerful platform for building and training neural networks.