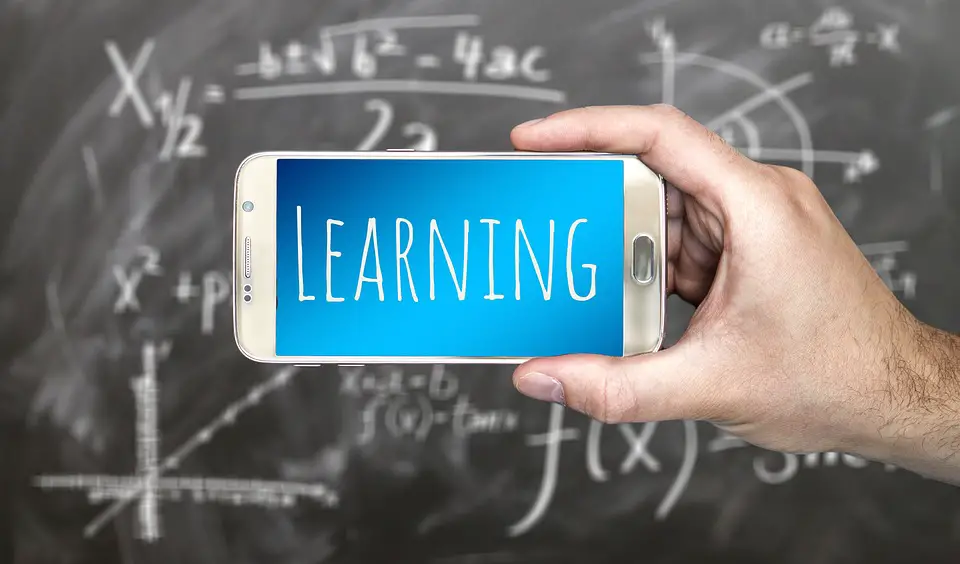
Python is a powerful and versatile programming language that is widely used for a variety of applications, from web development to data analysis. However, like any programming language, Python code can sometimes be slow and inefficient, particularly when dealing with large amounts of data. One way to improve the efficiency of your Python code is to use the map function.
The map function in Python is a built-in function that applies a given function to each element of an iterable (such as a list or tuple) and returns a new iterable with the results. By using the map function, you can avoid writing explicit loops and make your code more concise and readable.
However, simply using the map function is not enough to optimize your code. There are several strategies you can employ to make your code even more efficient when using the map function.
One strategy is to use lambda functions with the map function. Lambda functions are anonymous functions that can be defined in a single line of code. By using lambda functions with the map function, you can avoid defining separate functions for simple operations and make your code more compact.
For example, consider the following code snippet that uses a lambda function with the map function to compute the square of each element in a list:
“`
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x**2, numbers))
print(squared_numbers)
“`
Another strategy for optimizing your Python code with the map function is to use list comprehension. List comprehension is a concise way to create lists in Python, and it can often be more efficient than using the map function.
For example, consider the following code snippet that uses list comprehension to compute the square of each element in a list:
“`
numbers = [1, 2, 3, 4, 5]
squared_numbers = [x**2 for x in numbers]
print(squared_numbers)
“`
In general, list comprehension is more readable and easier to understand than using the map function with lambda functions. However, the choice between list comprehension and the map function ultimately depends on personal preference and the specific requirements of your code.
In conclusion, optimizing your Python code with the map function can help improve the efficiency of your code and make it more readable and maintainable. By using lambda functions, list comprehension, and other strategies, you can make your code more efficient and achieve better performance when working with large amounts of data.