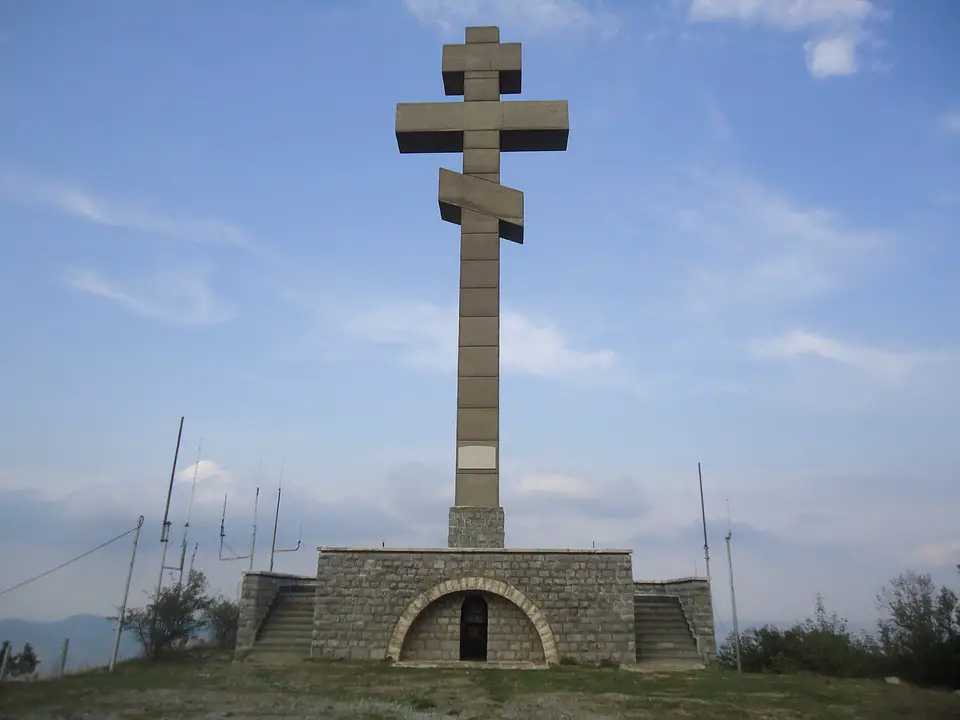
Python is a versatile and powerful programming language that is used in a wide variety of applications, from web development to data analysis. However, like any programming language, Python code can sometimes be slow and inefficient. One way to optimize your Python code and make it run faster is to use the range function.
The range function in Python is a built-in function that generates a sequence of numbers. It is commonly used in loops to iterate over a sequence of numbers. By using the range function effectively, you can make your code more efficient and improve its performance.
One way to optimize your Python code using the range function is to avoid creating unnecessary lists. For example, consider the following code snippet:
“`
numbers = list(range(1000000))
for number in numbers:
print(number)
“`
In this code, a list of numbers from 0 to 999999 is created using the range function, and then each number is printed out using a for loop. However, creating a list of such a large size can be memory-intensive and slow down your code. Instead, you can use the range function directly in the for loop like this:
“`
for number in range(1000000):
print(number)
“`
By using the range function directly in the for loop, you avoid creating a list of numbers and make your code more efficient.
Another way to optimize your Python code using the range function is to specify the step parameter. By default, the range function generates a sequence of numbers with a step size of 1. However, you can specify a different step size to iterate over the sequence in larger increments. For example:
“`
for number in range(0, 100, 10):
print(number)
“`
In this code, the range function generates a sequence of numbers from 0 to 100 with a step size of 10. This can be useful when you only need to iterate over a subset of numbers in a large range.
In conclusion, the range function in Python is a powerful tool that can help you optimize your code and make it run faster. By using the range function effectively, you can avoid creating unnecessary lists and specify the step size to iterate over a sequence in larger increments. Next time you are writing Python code, consider using the range function to optimize your code and improve its performance.