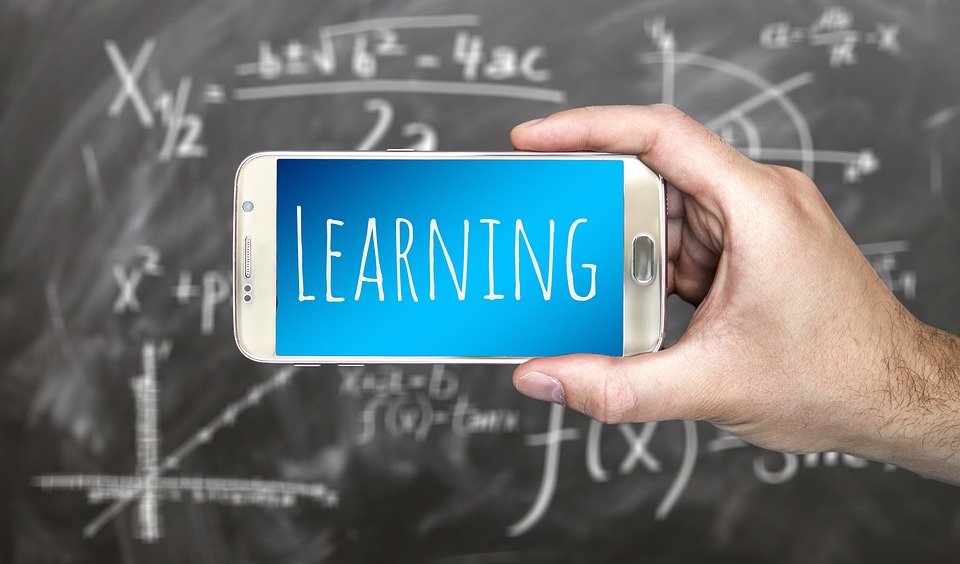
Python lists are a fundamental data structure in Python that allow for the storage and manipulation of a collection of elements. While lists are incredibly versatile and easy to use, they can also be a source of performance bottlenecks if not optimized properly. In this article, we will explore some tips and tricks for optimizing performance with Python lists.
1. Use List Comprehensions: List comprehensions are a concise and efficient way to create lists in Python. They allow for the creation of lists in a single line of code, which can be much faster than using traditional for loops. For example, instead of using a for loop to create a list of squares of numbers from 1 to 10, you can use a list comprehension like this:
squares = [x**2 for x in range(1, 11)]
2. Avoid Unnecessary Copying: In Python, lists are mutable objects, which means that they can be modified in place. However, when you perform operations on lists, such as slicing or concatenation, Python creates a new copy of the list. This can be inefficient, especially for large lists. To avoid unnecessary copying, consider using the extend() method instead of concatenating lists or using in-place operations when possible.
3. Use Generators: Generators are a powerful tool for optimizing performance with lists in Python. They allow for lazy evaluation of elements, which means that elements are computed only when they are needed. This can be especially useful when dealing with large lists or when memory is limited. To create a generator, you can use a generator expression or the yield keyword in a function.
4. Use the Built-in Functions: Python provides a variety of built-in functions for working with lists, such as map(), filter(), and reduce(). These functions can be more efficient than using for loops or list comprehensions in certain situations. For example, the map() function can be used to apply a function to each element in a list, while the filter() function can be used to filter elements based on a condition.
5. Use NumPy for Numeric Operations: If you are working with large arrays of numeric data, consider using NumPy, a powerful library for numerical computing in Python. NumPy provides efficient data structures and functions for performing operations on arrays, which can be much faster than using standard Python lists. NumPy arrays are also optimized for vectorized operations, which can further improve performance.
In conclusion, optimizing performance with Python lists involves using efficient techniques such as list comprehensions, avoiding unnecessary copying, using generators, leveraging built-in functions, and considering specialized libraries like NumPy for specific use cases. By following these tips and tricks, you can improve the performance of your Python code when working with lists.