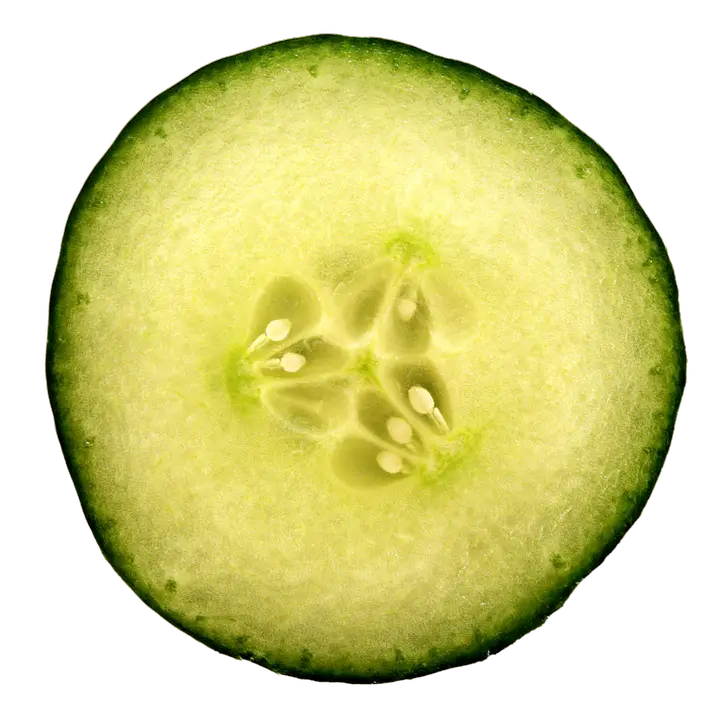
Python lists are a versatile and powerful data structure that is commonly used in a wide range of applications. However, as with any programming language, there are best practices that can be followed to optimize the performance of your code when working with lists. In this article, we will explore some of the best practices for working with Python lists to ensure fast and efficient code.
1. Use list comprehensions:
List comprehensions are a concise and efficient way to create lists in Python. They are often faster than using traditional for loops to create lists, as they are implemented in C and are optimized for speed. For example, instead of using a for loop to create a list of squared numbers, you can use a list comprehension like this:
“` python
squared_numbers = [x**2 for x in range(10)]
“`
2. Avoid appending in a loop:
Appending to a list in a loop can be inefficient, as each append operation involves resizing the list. Instead, consider preallocating the list if you know the final size, or using list comprehensions to create the list in one go. If you do need to append to a list in a loop, consider using the `extend` method to add multiple elements at once, rather than appending one element at a time.
3. Use built-in functions:
Python provides a number of built-in functions that can be used to manipulate lists efficiently. For example, the `sum` function can be used to quickly calculate the sum of a list of numbers, the `max` and `min` functions can be used to find the maximum and minimum values in a list, and the `sorted` function can be used to sort a list.
4. Use slicing for copying and modifying lists:
When you need to create a copy of a list or modify a list in place, consider using slicing. Slicing is a fast and efficient way to create a copy of a list or modify a list without creating a new list. For example, to create a copy of a list, you can use slicing like this:
“` python
original_list = [1, 2, 3, 4, 5]
copied_list = original_list[:]
“`
5. Use generators for large lists:
If you need to work with large lists or generate lists dynamically, consider using generators. Generators are a memory-efficient way to generate lists on the fly, as they only compute values as needed. This can be particularly useful when working with large datasets or when memory is limited.
In conclusion, optimizing performance with Python lists involves following best practices such as using list comprehensions, avoiding appending in loops, using built-in functions, using slicing for copying and modifying lists, and using generators for large lists. By following these best practices, you can ensure that your code is fast and efficient when working with Python lists.