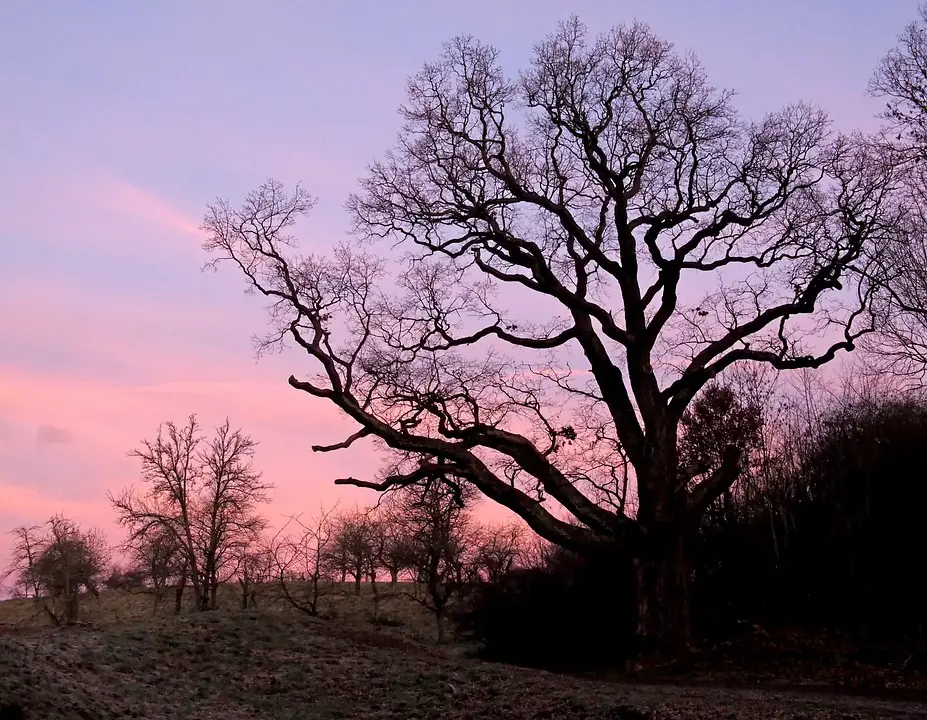
Python’s range function is a powerful tool that can help maximize efficiency when working with loops and sequences in Python. By understanding how to use the range function effectively, you can write more concise and optimized code that runs faster and uses less memory.
The range function in Python generates a sequence of numbers within a specified range. It can take up to three arguments: start, stop, and step. By default, the range function starts at 0 and increments by 1, but you can customize the range to fit your specific needs.
One of the key benefits of using the range function is that it allows you to iterate over a sequence of numbers without having to explicitly create a list of numbers. This can be particularly useful when working with large datasets or when memory efficiency is a concern.
For example, instead of writing a loop like this:
“`
for i in range(0, 10):
print(i)
“`
You can write the following more efficient code using the range function:
“`
for i in range(10):
print(i)
“`
This will produce the same output but without the overhead of creating a list of numbers from 0 to 10.
Another way to maximize efficiency with the range function is by using it in combination with other built-in functions, such as zip and enumerate. For example, you can use the range function to iterate over two lists simultaneously using the zip function:
“`
list1 = [1, 2, 3, 4]
list2 = [‘a’, ‘b’, ‘c’, ‘d’]
for i, j in zip(range(len(list1)), list2):
print(i, j)
“`
This code snippet will iterate over both lists simultaneously, printing the index from the range function and the corresponding element from list2.
Similarly, you can use the range function in conjunction with the enumerate function to iterate over a sequence while also keeping track of the index:
“`
my_list = [‘apple’, ‘banana’, ‘cherry’]
for i, item in enumerate(my_list):
print(i, item)
“`
This will output the index and the corresponding element from the list.
In conclusion, the range function in Python is a versatile tool that can help you maximize efficiency when working with loops and sequences. By understanding how to use the range function effectively, you can write more concise and optimized code that runs faster and uses less memory. So next time you are writing a loop in Python, consider using the range function to streamline your code and improve performance.