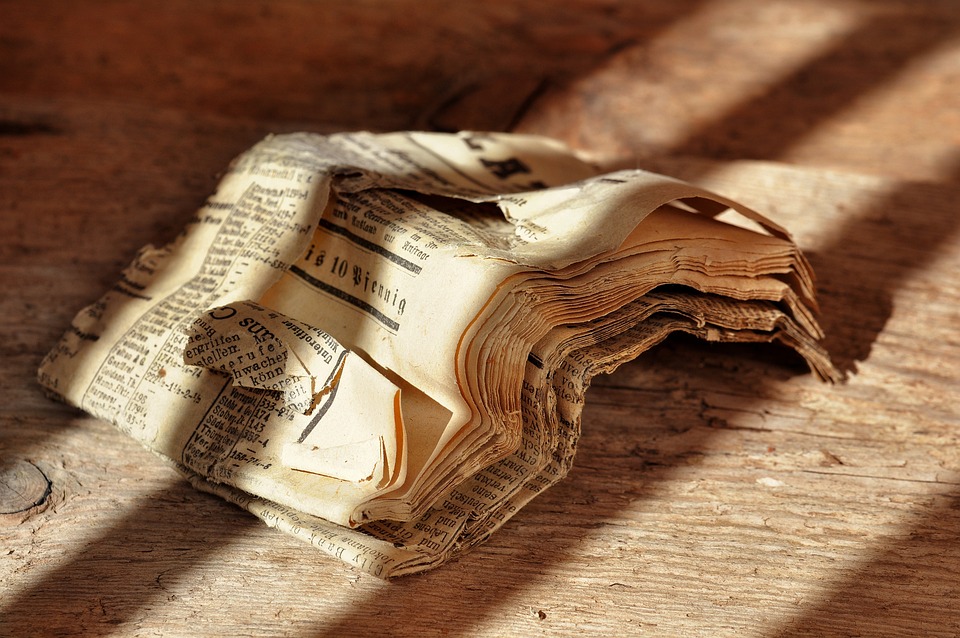
The JavaScript map function is a powerful tool that allows developers to efficiently transform and manipulate arrays. This function is commonly used in modern web development to streamline data processing and improve code readability. In this beginner’s guide, we will explore the basics of the map function and how to effectively use it in your projects.
The map function is a higher-order function in JavaScript that takes in a callback function as its argument. This callback function is then applied to each element of an array, creating a new array with the transformed elements. This allows developers to easily iterate over arrays and perform operations on each element without the need for traditional for loops.
To use the map function, simply call it on an array and pass in a callback function as an argument. The callback function should take in the current element, index, and array as parameters, and return the transformed value. Here is an example of how to use the map function to double each element in an array:
“`javascript
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map((number) => number * 2);
console.log(doubledNumbers); // [2, 4, 6, 8, 10]
“`
In this example, we create an array of numbers and use the map function to double each element. The callback function takes in the current number and returns the number multiplied by 2. The map function then creates a new array with the transformed elements.
One of the key advantages of the map function is that it creates a new array without mutating the original array. This makes it a safe and efficient way to transform data without affecting the original data structure. Additionally, the map function can be chained with other array methods like filter and reduce to create complex data transformations.
Another useful feature of the map function is that it can be used to iterate over objects by converting them into arrays. By using Object.entries() and Object.values() methods, developers can easily iterate over object properties and perform operations on them using the map function.
Overall, mastering the map function in JavaScript is essential for any developer looking to efficiently manipulate arrays and objects in their projects. By understanding the basics of the map function and practicing its usage, developers can streamline their code and improve their data processing capabilities. Whether you are a beginner or an experienced developer, the map function is a valuable tool to have in your JavaScript toolkit.