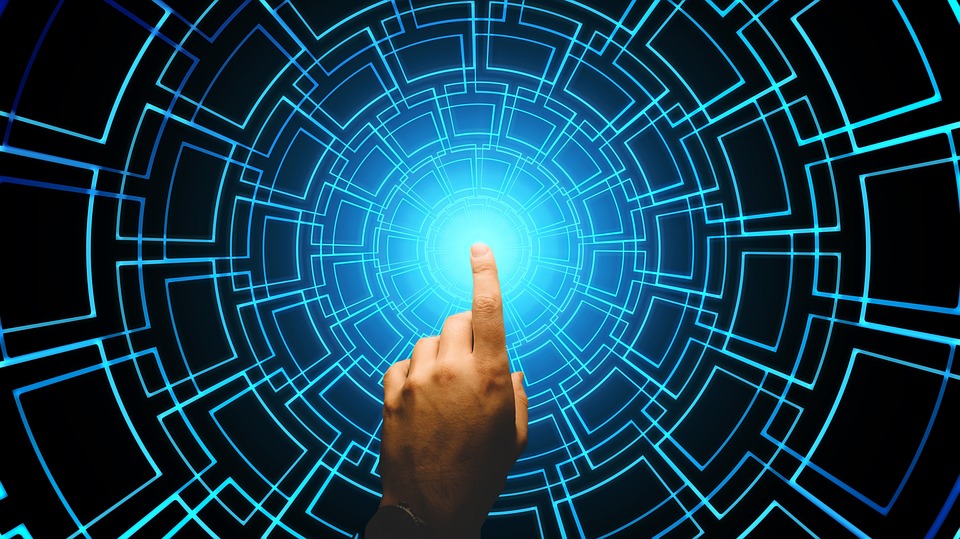
JavaScript filters are a powerful tool that allow developers to manipulate, sort, and filter data in a web application. By mastering the art of JavaScript filters, developers can create more efficient and user-friendly applications that provide a better user experience.
In this complete guide, we will explore the different types of filters available in JavaScript, how to use them effectively, and some best practices for implementing filters in your web applications.
Types of JavaScript Filters
There are several types of filters that can be used in JavaScript to manipulate data. Some of the most common types include:
1. Array.prototype.filter(): This method creates a new array with all elements that pass a certain test implemented by the provided function. It is commonly used to filter an array based on a specific condition.
2. Array.prototype.sort(): This method sorts the elements of an array in place and returns the sorted array. It can be used to sort an array based on a specific property or condition.
3. Array.prototype.map(): This method creates a new array with the results of calling a provided function on every element in the array. It is commonly used to transform data in an array.
4. Array.prototype.reduce(): This method applies a function against an accumulator and each element in the array (from left to right) to reduce it to a single value. It is commonly used to perform calculations on an array.
Using Filters Effectively
To use JavaScript filters effectively, it is important to understand the syntax and parameters of each filter method. Here are some tips for using filters effectively in your web applications:
1. Use filter() to filter an array based on a specific condition:
“`javascript
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers); // Output: [2, 4]
“`
2. Use sort() to sort an array based on a specific property or condition:
“`javascript
const users = [
{ name: ‘John’, age: 25 },
{ name: ‘Jane’, age: 30 },
{ name: ‘Alice’, age: 20 }
];
users.sort((a, b) => a.age – b.age);
console.log(users); // Output: [{ name: ‘Alice’, age: 20 }, { name: ‘John’, age: 25 }, { name: ‘Jane’, age: 30 }]
“`
3. Use map() to transform data in an array:
“`javascript
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map(num => num * num);
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
“`
4. Use reduce() to perform calculations on an array:
“`javascript
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((acc, curr) => acc + curr, 0);
console.log(sum); // Output: 15
“`
Best Practices for Implementing Filters
When implementing filters in your web applications, it is important to follow some best practices to ensure that the filters work efficiently and provide a good user experience. Here are some best practices for implementing filters in your web applications:
1. Provide clear filter options: Make sure that users can easily understand and use the filters in your application. Provide clear labels and options for filtering data.
2. Use debounce for live search filters: If you have a live search filter in your application, use debounce to delay the filter function and prevent it from being called too frequently.
3. Use pagination for large datasets: If you are filtering a large dataset, consider implementing pagination to display the filtered data in smaller chunks and improve performance.
4. Test filters on different devices and browsers: Make sure to test the filters on different devices and browsers to ensure that they work correctly and provide a consistent user experience.
By mastering the art of JavaScript filters, developers can create more efficient and user-friendly web applications that provide a better user experience. By understanding the different types of filters available, using filters effectively, and following best practices for implementing filters, developers can create powerful and dynamic web applications that meet the needs of users.