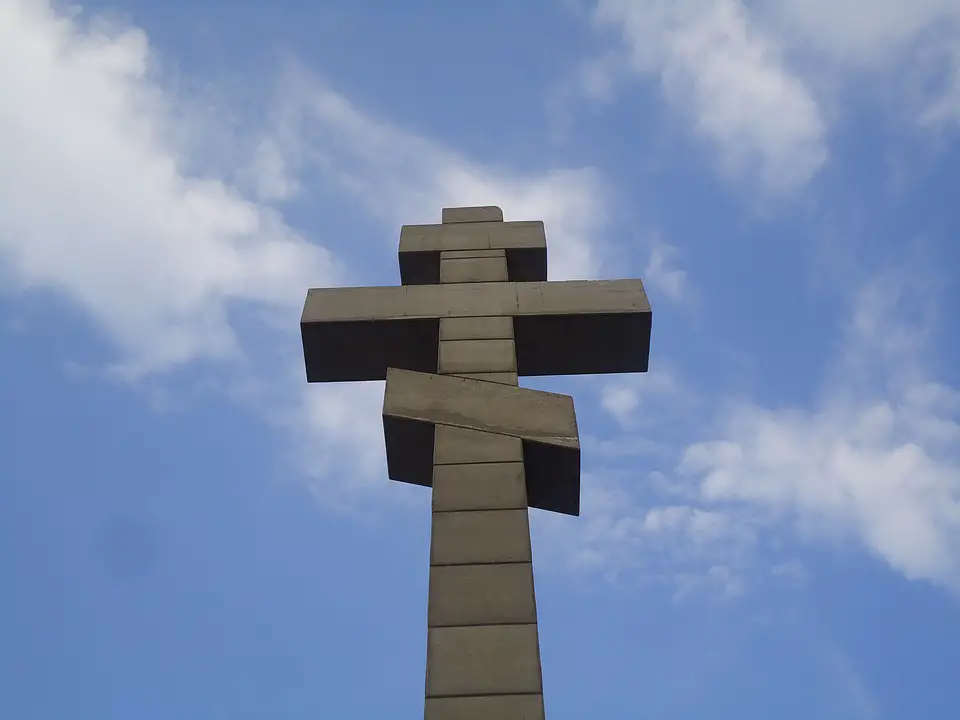
Text manipulation is a common task in programming, especially when dealing with large amounts of text data. Python, being a versatile and powerful programming language, offers several methods to manipulate text efficiently. One such method is the split() function, which allows you to split a string into a list of substrings based on a delimiter. In this article, we will explore how to master text manipulation with Python split() function through a step-by-step guide.
Step 1: Understanding the split() function
The split() function in Python takes a delimiter as an argument and splits the input string based on that delimiter. By default, the delimiter is a whitespace character, but you can specify any character or substring as the delimiter. The function returns a list of substrings where the input string has been split.
Step 2: Splitting a string using the default delimiter
Let’s start by splitting a string using the default delimiter, which is a whitespace character. Consider the following example:
“` python
text = “ Python is a versatile programming language”
words = text.split()
print(words)
“`
Output:
[‘ Python’, ‘is’, ‘a’, ‘versatile’, ‘programming’, ‘language’]In this example, the split() function splits the input string ‘text’ into a list of words based on the whitespace delimiter. The resulting list ‘words’ contains individual words from the input string.
Step 3: Splitting a string using a custom delimiter
You can also split a string using a custom delimiter of your choice. For example, if you want to split a string based on a comma delimiter, you can do so as follows:
“` python
text = “apple,banana,orange,grape”
fruits = text.split(‘,’)
print(fruits)
“`
Output:
[‘apple’, ‘banana’, ‘orange’, ‘grape’]In this example, the split() function splits the input string ‘text’ into a list of fruits based on the comma delimiter. The resulting list ‘fruits’ contains individual fruit names from the input string.
Step 4: Handling multiple delimiters
You can also split a string using multiple delimiters by passing a list of delimiters as an argument to the split() function. For example, if you want to split a string based on both comma and whitespace delimiters, you can do so as follows:
“` python
text = “apple, banana orange grape”
fruits = text.split([‘, ‘, ‘ ‘])
print(fruits)
“`
Output:
[‘apple’, ‘banana’, ‘orange’, ‘grape’]In this example, the split() function splits the input string ‘text’ into a list of fruits based on both comma and whitespace delimiters. The resulting list ‘fruits’ contains individual fruit names from the input string.
In conclusion, mastering text manipulation with Python split() function can greatly enhance your ability to manipulate text data efficiently. By understanding the various ways in which you can use the split() function, you can easily split strings based on different delimiters and handle complex text manipulation tasks with ease. Practice using the split() function with different examples to become more proficient in text manipulation with Python.