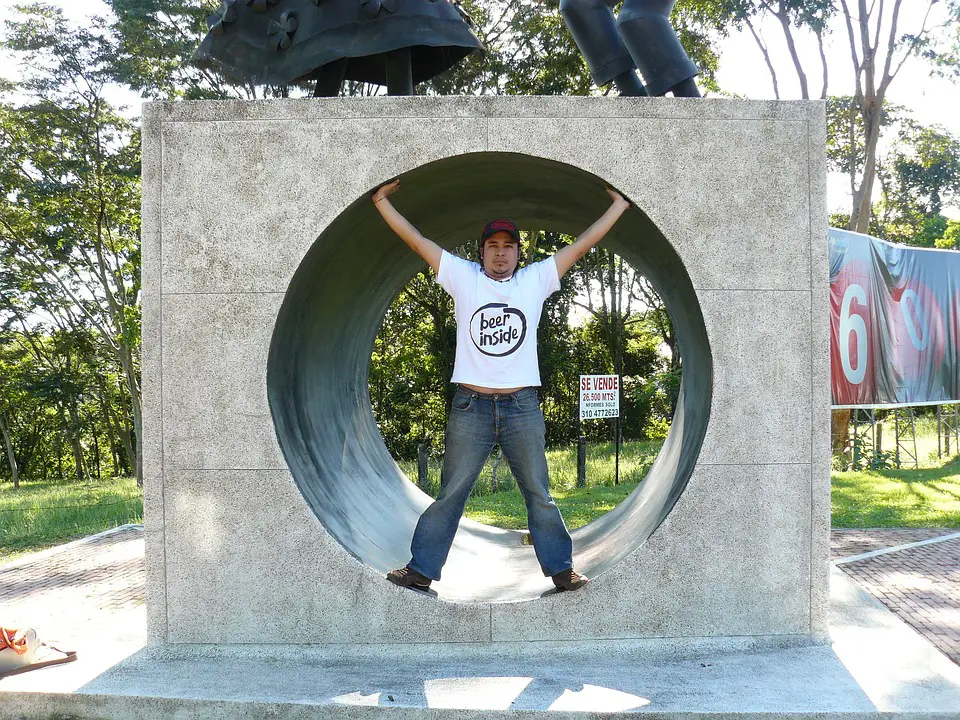
Python’s range function is a powerful tool that allows you to generate a sequence of numbers within a specified range. It is commonly used in loops and list comprehensions to iterate over a sequence of numbers. In this article, we will explore the ins and outs of Python’s range function and how you can master it for your programming needs.
The syntax of the range function is as follows:
range(start, stop, step)
The start parameter is the starting value of the sequence, while the stop parameter is the value at which the sequence ends (exclusive). The step parameter is the increment between each value in the sequence. By default, the start parameter is 0 and the step parameter is 1.
Here’s an example of how you can use the range function in a for loop:
“` python
for i in range(5):
print(i)
“`
This code will output the numbers 0, 1, 2, 3, and 4, as the range function generates a sequence of numbers from 0 to 5 (exclusive) with a step of 1.
You can also specify the start and stop parameters to generate a sequence within a specific range. For example:
“` python
for i in range(1, 6):
print(i)
“`
This code will output the numbers 1, 2, 3, 4, and 5, as the range function generates a sequence of numbers from 1 to 6 (exclusive) with a step of 1.
Additionally, you can specify the step parameter to generate a sequence with a custom increment. For example:
“` python
for i in range(0, 10, 2):
print(i)
“`
This code will output the numbers 0, 2, 4, 6, and 8, as the range function generates a sequence of numbers from 0 to 10 (exclusive) with a step of 2.
You can also create a list of numbers using the range function by converting it to a list:
“` python
numbers = list(range(5))
print(numbers)
“`
This code will output [0, 1, 2, 3, 4], as the range function generates a sequence of numbers from 0 to 5 (exclusive) and converts it to a list.
In addition to loops, the range function can also be used in list comprehensions to create a list of numbers based on a specific condition. For example:
“` python
even_numbers = [x for x in range(10) if x % 2 == 0]
print(even_numbers)
“`
This code will output [0, 2, 4, 6, 8], as the list comprehension generates a list of even numbers from 0 to 10 (exclusive).
In conclusion, mastering Python’s range function can greatly enhance your programming capabilities by allowing you to generate sequences of numbers efficiently. By understanding the syntax and various parameters of the range function, you can leverage its power in loops, list comprehensions, and other programming tasks. Practice using the range function in different scenarios to become proficient in its usage and unlock its full potential in your Python projects.