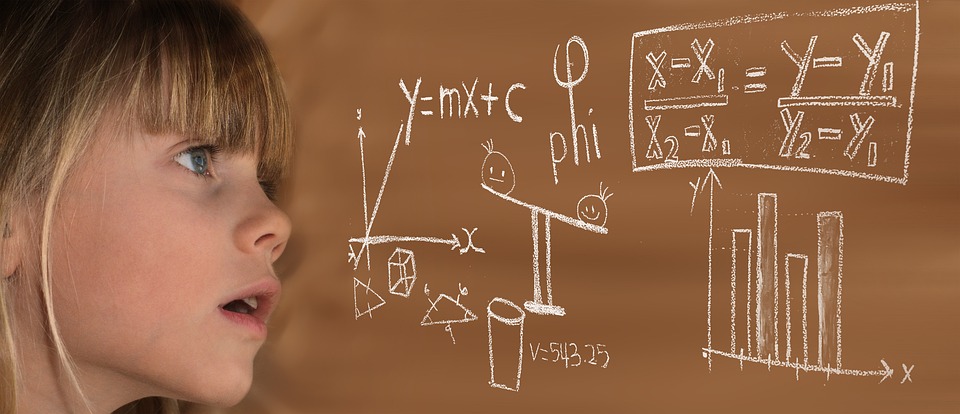
Python sets are a powerful data structure that allows you to store unique elements in an unordered collection. Sets are widely used in data analysis and manipulation as they provide efficient ways to perform various operations such as intersection, union, difference, and symmetric difference.
In this article, we will explore some advanced techniques for mastering Python sets in data analysis.
1. Creating sets:
To create a set in Python, you can use curly braces or the set() constructor. Sets can contain any immutable data types such as integers, strings, tuples, and frozensets. For example:
“` python
set1 = 1, 2, 3, 4, 5
set2 = set([4, 5, 6, 7, 8])
“`
2. Set operations:
Python sets support several operations that are useful for data analysis. Here are some common set operations:
– Union: Combines two sets and returns a new set containing all unique elements from both sets.
“` python
union_set = set1.union(set2)
“`
– Intersection: Returns a new set containing elements that are common in both sets.
“` python
intersection_set = set1.intersection(set2)
“`
– Difference: Returns a new set containing elements that are present in the first set but not in the second set.
“` python
difference_set = set1.difference(set2)
“`
– Symmetric difference: Returns a new set containing elements that are present in either of the sets, but not in both sets.
“` python
symmetric_difference_set = set1.symmetric_difference(set2)
“`
3. Set comprehension:
Similar to list comprehension, you can also use set comprehension to create sets in a concise way. Set comprehension uses curly braces and a loop to iterate over elements. For example:
“` python
set3 = x for x in range(10) if x % 2 == 0
“`
4. Set methods:
Python sets provide various methods that are useful for data analysis. Some of the commonly used set methods include:
– add(): Adds an element to the set.
“` python
set1.add(6)
“`
– remove(): Removes a specified element from the set. Raises an error if the element is not present in the set.
“` python
set1.remove(5)
“`
– discard(): Removes a specified element from the set. Does not raise an error if the element is not present in the set.
“` python
set1.discard(5)
“`
– clear(): Removes all elements from the set.
“` python
set1.clear()
“`
5. Set operations on multiple sets:
You can perform set operations on multiple sets using the set methods or operators. For example:
“` python
set1.update(set2) # Updates set1 with elements from set2
set1 |= set2 # Equivalent to set1.update(set2)
“`
Overall, mastering Python sets is essential for efficient data analysis and manipulation. By using advanced techniques such as set operations, set comprehension, and set methods, you can perform complex data operations with ease. Experiment with Python sets in your data analysis projects to leverage their power and flexibility.