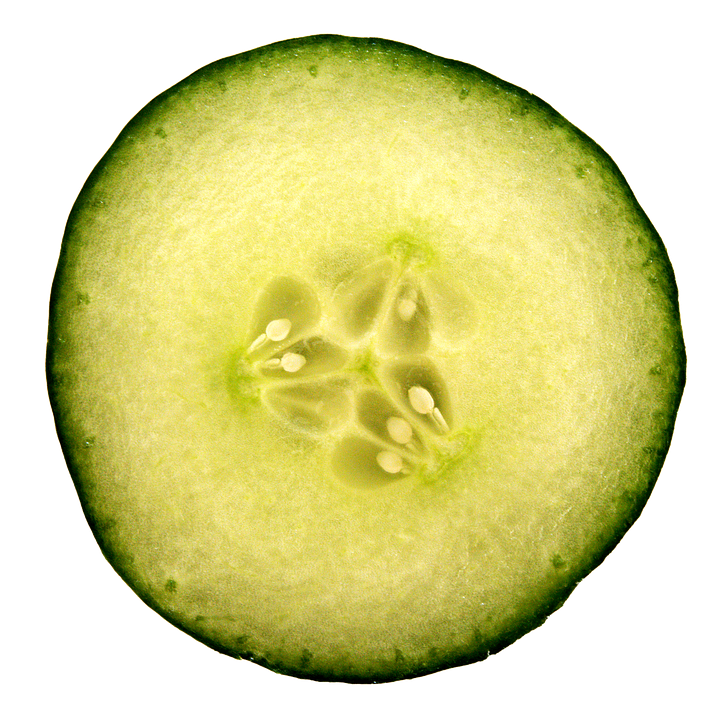
Python’s map function is a powerful tool for efficiently manipulating data in Python. By applying a function to each element in an iterable, map allows you to quickly transform and process large amounts of data with minimal code.
In this article, we will explore some tips and tricks for mastering Python map and using it effectively in your data manipulation projects.
1. Understanding the Basics:
Before diving into the advanced features of map, it’s important to understand the basics. The map function takes two arguments – a function and an iterable. The function is applied to each element in the iterable, and the result is returned as a new iterable.
For example, consider the following code snippet:
“` python
numbers = [1, 2, 3, 4, 5]
squared_numbers = map(lambda x: x**2, numbers)
print(list(squared_numbers))
“`
In this example, we use a lambda function to square each element in the list of numbers. The result is a new list of squared numbers.
2. Using Multiple Iterables:
One of the key features of map is that it can take multiple iterables as arguments. This allows you to apply a function to elements from different iterables in parallel.
For example, consider the following code snippet:
“` python
numbers1 = [1, 2, 3, 4, 5]
numbers2 = [6, 7, 8, 9, 10]
sum_numbers = map(lambda x, y: x + y, numbers1, numbers2)
print(list(sum_numbers))
“`
In this example, we use map to add corresponding elements from two lists. The result is a new list of sums.
3. Using map with Other Functions:
In addition to lambda functions, you can also use other functions with map. This allows you to apply more complex transformations to your data.
For example, consider the following code snippet:
“` python
def double(x):
return x * 2
numbers = [1, 2, 3, 4, 5]
doubled_numbers = map(double, numbers)
print(list(doubled_numbers))
“`
In this example, we define a simple function that doubles each element in the list of numbers. The result is a new list of doubled numbers.
4. Combining map with Filter and Reduce:
Map can also be combined with other functions like filter and reduce to perform more complex data manipulations.
For example, consider the following code snippet:
“` python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
filtered_numbers = filter(lambda x: x % 2 == 0, numbers)
sum_even_numbers = reduce(lambda x, y: x + y, map(lambda x: x**2, filtered_numbers))
print(sum_even_numbers)
“`
In this example, we first filter out the even numbers from the list, then square each of them, and finally sum them up using reduce.
In conclusion, mastering Python map can greatly improve your efficiency in data manipulation tasks. By understanding the basics, using multiple iterables, combining map with other functions, and leveraging different types of functions, you can take full advantage of map’s capabilities. Practice using map with different types of data and functions to become proficient in using this powerful tool for data manipulation in Python.