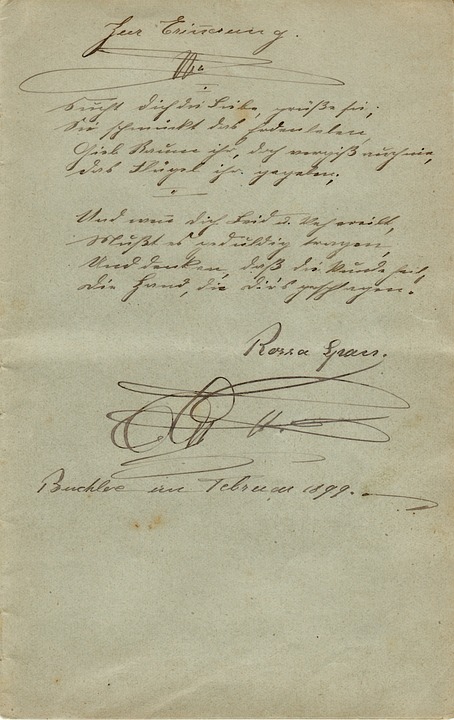
JavaScript is a powerful programming language that is commonly used to create interactive websites and web applications. One of the key features of JavaScript is its ability to manipulate strings, which are sequences of characters. Substring functions in JavaScript allow developers to extract a portion of a string, making it easier to work with specific parts of the text.
In this beginner’s guide, we will explore how to use JavaScript substring functions to master string manipulation.
Getting Started with Substring Functions
To use substring functions in JavaScript, you will first need to have a basic understanding of strings. Strings in JavaScript are enclosed in single quotes, double quotes, or backticks. Here is an example of a string variable in JavaScript:
“`
let myString = “Hello, world!”;
“`
Now that you have a string variable, you can start using substring functions to extract a portion of the text. The two main substring functions in JavaScript are `substring()` and `substr()`.
Using the `substring()` Function
The `substring()` function in JavaScript is used to extract a portion of a string based on the specified start and end indexes. Here is the syntax for the `substring()` function:
“`
substring(startIndex, endIndex)
“`
The `startIndex` parameter specifies the index at which to start extracting the substring, and the `endIndex` parameter specifies the index at which to stop extracting the substring (but not including the character at that index).
Let’s see an example of how to use the `substring()` function:
“`
let myString = “Hello, world!”;
let substring = myString.substring(0, 5);
console.log(substring); // Output: Hello
“`
In this example, we are extracting the substring starting from index 0 (the first character) up to index 5 (not including the character at index 5).
Using the `substr()` Function
The `substr()` function in JavaScript is similar to the `substring()` function, but it takes a different set of parameters. Here is the syntax for the `substr()` function:
“`
substr(startIndex, length)
“`
The `startIndex` parameter specifies the index at which to start extracting the substring, and the `length` parameter specifies the number of characters to extract.
Let’s see an example of how to use the `substr()` function:
“`
let myString = “Hello, world!”;
let substring = myString.substr(7, 5);
console.log(substring); // Output: world
“`
In this example, we are extracting the substring starting from index 7 (the eighth character) and extracting a total of 5 characters.
Conclusion
Substrings functions in JavaScript are powerful tools for manipulating strings and extracting specific parts of text. By mastering these functions, you can enhance your skills as a JavaScript developer and build more dynamic and interactive web applications.
In this beginner’s guide, we covered the basics of using the `substring()` and `substr()` functions in JavaScript. Practice using these functions with different strings and parameters to become more comfortable with string manipulation in JavaScript.