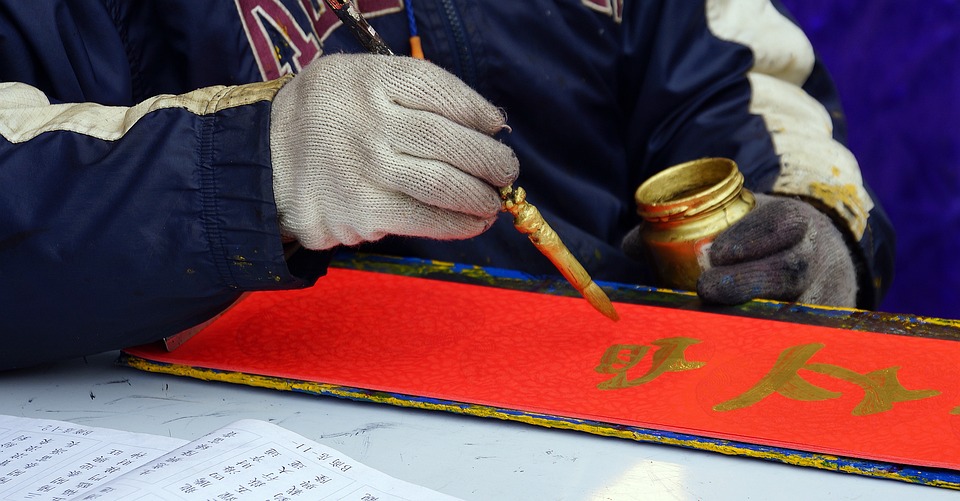
JavaScript is a powerful programming language that is widely used for creating interactive websites and web applications. One key feature of JavaScript is the `forEach` method, which allows developers to iterate over arrays and perform actions on each element. Mastering the `forEach` method is essential for any JavaScript developer looking to enhance their skills and create more efficient code.
In this comprehensive guide, we will explore the ins and outs of the `forEach` method in JavaScript, and provide tips and tricks for mastering this essential tool.
What is the `forEach` method?
The `forEach` method is a built-in method in JavaScript that allows developers to iterate over each element in an array and perform a specified action on each element. This method is commonly used for looping through arrays and performing tasks such as data manipulation, filtering, or rendering elements on a webpage.
Syntax of the `forEach` method:
The syntax of the `forEach` method is simple and easy to understand. It takes a callback function as an argument, which is executed for each element in the array.
“`
array.forEach(function(element, index, array) {
// code to be executed on each element
})
“`
The `forEach` method takes three parameters:
1. The `element` parameter represents the current element being processed in the array.
2. The `index` parameter represents the index of the current element in the array.
3. The `array` parameter represents the array that the `forEach` method is being called on.
Using the `forEach` method:
To use the `forEach` method, simply call it on an array and pass in a callback function to specify the action to be performed on each element. Here is an example of how to use the `forEach` method to log each element in an array to the console:
“`
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number) {
console.log(number);
});
“`
This will output:
“`
1
2
3
4
5
“`
Tips for mastering the `forEach` method:
1. Use arrow functions for concise syntax: Arrow functions provide a more concise syntax for defining callback functions. Instead of using the `function` keyword, you can use an arrow function to make your code cleaner and more readable.
“`
numbers.forEach((number) => {
console.log(number);
});
“`
2. Use the index parameter for additional functionality: The `index` parameter in the `forEach` method can be used to access the index of each element in the array. This can be useful for performing actions based on the position of elements in the array.
“`
numbers.forEach((number, index) => {
console.log(`Element at index ${index} is ${number}`);
});
“`
3. Avoid using `return` statements: Unlike other looping methods in JavaScript, the `forEach` method does not return a new array. Therefore, using `return` statements within the callback function will not have any effect. If you need to create a new array based on the elements of the original array, consider using methods like `map` or `filter`.
In conclusion, mastering the `forEach` method in JavaScript is essential for any developer looking to create efficient and clean code. By understanding the syntax and functionality of the `forEach` method, and following these tips and tricks, you can take your JavaScript skills to the next level and create more dynamic and interactive web applications.