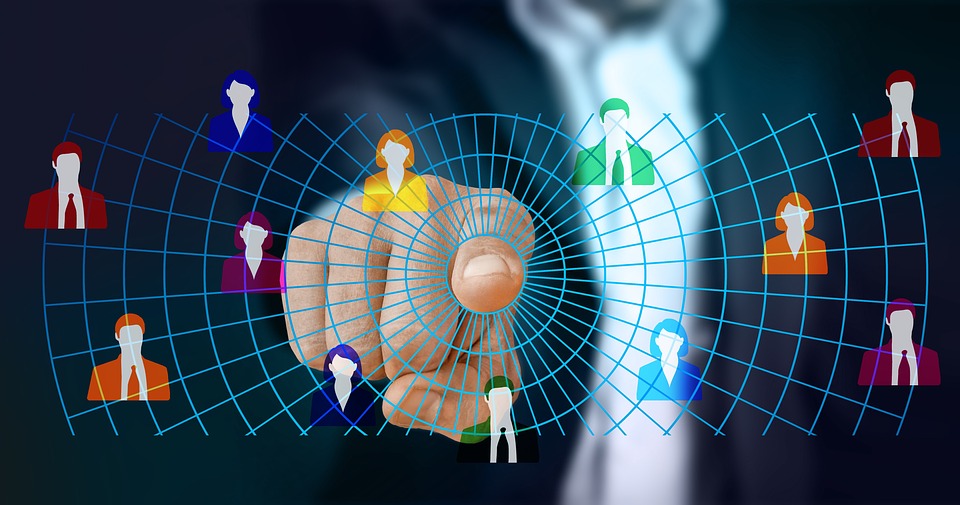
JavaScript is a versatile and powerful programming language that is widely used for creating dynamic and interactive websites. One of the key features of JavaScript is its ability to manipulate arrays and iterate over their elements. The forEach method is a handy tool for iterating over arrays in JavaScript, and mastering it can greatly enhance your coding skills.
The forEach method is used to iterate over the elements of an array and perform a specified action on each element. It is a higher-order function, which means that it takes another function as an argument. This function is known as the callback function, and it is called for each element in the array.
To use the forEach method, you simply call it on the array that you want to iterate over, and pass in the callback function as an argument. The callback function takes three arguments: the current element being processed, the index of that element in the array, and the array itself.
Here is an example of how to use the forEach method to iterate over an array of numbers and log each element to the console:
“`
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number, index, array) {
console.log(`Element ${number} at index ${index}`);
});
“`
In this example, the callback function logs each element of the array along with its index.
One important thing to note about the forEach method is that it does not return anything. It simply iterates over the array and applies the callback function to each element. If you need to perform some kind of transformation on the elements of the array and create a new array, you may want to use the map method instead.
Another thing to keep in mind when using the forEach method is that it does not allow for early termination. Once you start iterating over the array, the forEach method will continue to the end, even if you use a break statement inside the callback function. If you need to break out of the loop early, you may want to use the some or every methods instead.
In conclusion, mastering the forEach method in JavaScript can greatly improve your ability to manipulate arrays and iterate over their elements. By understanding how the forEach method works and practicing using it in your code, you can become a more efficient and effective JavaScript developer. So go ahead and start experimenting with the forEach method in your own projects, and see how it can help you take your coding skills to the next level.