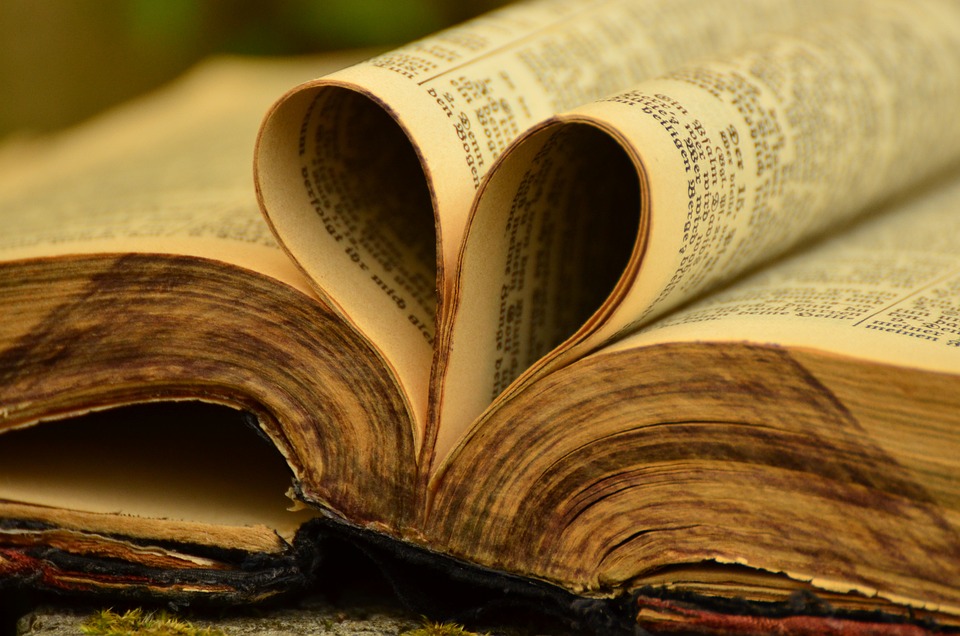
JavaScript is a powerful programming language that is widely used for developing dynamic and interactive web applications. One of the key features of JavaScript is the ability to iterate over arrays and perform operations on each element. One of the most popular methods for iterating over arrays in JavaScript is the forEach method.
The forEach method is a built-in method in JavaScript that allows you to iterate over an array and perform a specified operation on each element. This method is commonly used for performing tasks such as updating the values of array elements, filtering out certain elements, or performing calculations on each element.
Mastering the forEach method in JavaScript can greatly enhance your ability to work with arrays and perform complex operations on them. In this comprehensive guide, we will cover everything you need to know about the forEach method and how to use it effectively in your JavaScript code.
Syntax of the forEach Method:
The syntax of the forEach method is as follows:
array.forEach(callbackFunction(element, index, array) {
//perform operation on each element
});
The forEach method takes a callback function as an argument, which is executed for each element in the array. The callback function takes three parameters: the current element being processed, the index of the current element, and the array itself.
Using the forEach Method:
To use the forEach method, simply call it on an array and pass in a callback function that defines the operation to be performed on each element. Here is an example of how to use the forEach method to log each element in an array to the console:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach((number) => {
console.log(number);
});
In this example, the callback function logs each element in the numbers array to the console.
Common Use Cases for the forEach Method:
There are many use cases for the forEach method in JavaScript. Some common examples include:
– Updating the values of array elements
– Filtering out certain elements based on a condition
– Performing calculations on each element
– Executing asynchronous code for each element
It is important to note that the forEach method does not return a new array and does not modify the original array. If you need to create a new array based on the results of the forEach operation, you can use methods like map or filter.
Conclusion:
Mastering the forEach method in JavaScript is essential for working with arrays and performing complex operations on them. By understanding the syntax and use cases of the forEach method, you can efficiently iterate over arrays and perform operations on each element. Practice using the forEach method in your JavaScript code to become more proficient in working with arrays and enhancing the functionality of your web applications.