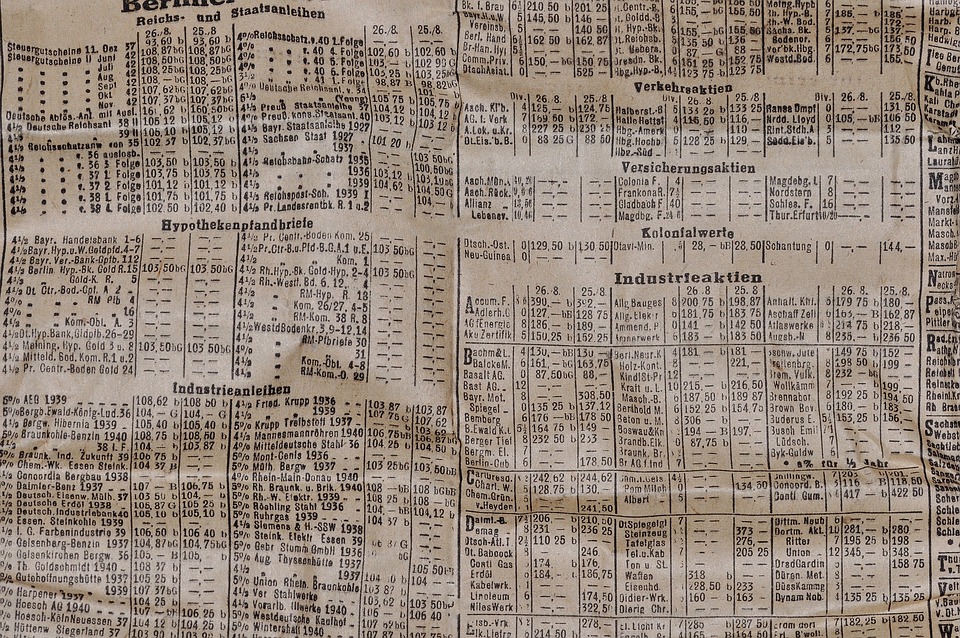
JavaScript is a powerful language that has become a staple in web development. One of the most useful features of JavaScript is the filter method, which allows developers to easily manipulate arrays and filter out elements based on certain criteria. In this article, we will discuss how to master the filter method in JavaScript and provide a complete guide for developers looking to improve their skills.
The filter method in JavaScript is used to create a new array with all elements that pass a certain test implemented by the provided function. This function is executed for each element in the array, and if the function returns true, the element is included in the new array. If the function returns false, the element is excluded from the new array.
Here is a basic example of how the filter method works:
“`javascript
const numbers = [1, 2, 3, 4, 5];
const filteredNumbers = numbers.filter(num => num > 3);
console.log(filteredNumbers); // Output: [4, 5]
“`
In this example, we have an array of numbers and we use the filter method to create a new array containing only the numbers that are greater than 3. The arrow function `num => num > 3` is the test function that determines whether each element should be included in the new array.
Now that we have a basic understanding of how the filter method works, let’s dive deeper into some more advanced techniques and best practices for using the filter method in JavaScript.
1. Chaining filter methods: One of the great things about the filter method is that it can be chained with other array methods such as map, reduce, and sort. This allows developers to perform complex operations on arrays with ease. Here is an example of chaining filter methods:
“`javascript
const numbers = [1, 2, 3, 4, 5];
const filteredNumbers = numbers
.filter(num => num > 2)
.map(num => num * 2);
console.log(filteredNumbers); // Output: [6, 8, 10]
“`
In this example, we first filter the array to include only numbers greater than 2, and then we use the map method to multiply each number by 2.
2. Using the index parameter: The filter method also provides an optional second parameter, which represents the index of the current element being processed. This can be useful when you need to perform a different test based on the index of the element. Here is an example:
“`javascript
const numbers = [1, 2, 3, 4, 5];
const filteredNumbers = numbers.filter((num, index) => index % 2 === 0);
console.log(filteredNumbers); // Output: [1, 3, 5]
“`
In this example, we filter the array to include only elements whose index is even.
3. Handling complex data structures: The filter method can be used to filter out elements from arrays of complex data structures such as objects. Here is an example:
“`javascript
const users = [
{ name: ‘John’, age: 30 },
{ name: ‘Jane’, age: 25 },
{ name: ‘Bob’, age: 35 }
];
const filteredUsers = users.filter(user => user.age > 30);
console.log(filteredUsers); // Output: [{ name: ‘Bob’, age: 35 }]
“`
In this example, we filter out users whose age is greater than 30.
In conclusion, mastering the filter method in JavaScript is essential for any developer looking to manipulate arrays efficiently. By understanding the basics of how the filter method works and practicing with more advanced techniques, developers can improve their skills and become more proficient in using JavaScript. By following the tips and examples provided in this article, developers can take their filter method skills to the next level and create more powerful and dynamic web applications.