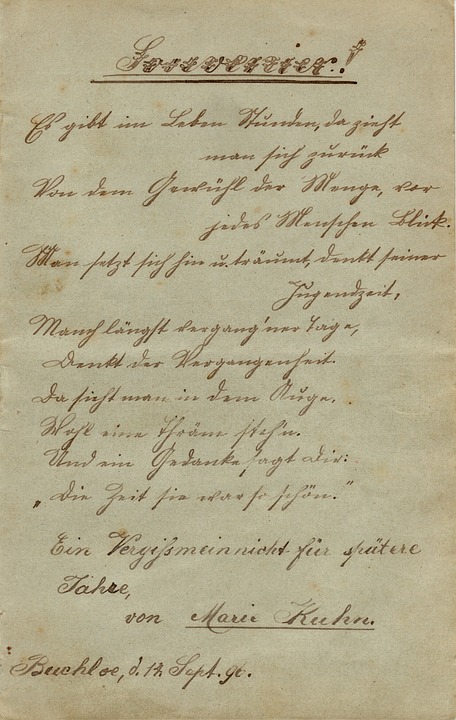
JavaScript is a powerful programming language that is widely used for web development. One of the most useful features of JavaScript is the filter method, which allows you to manipulate arrays in a variety of ways. In this article, we will explore how to master the JavaScript filter method and provide a complete guide on how to use it effectively.
The filter method in JavaScript is used to create a new array with all elements that pass a certain condition. This can be useful for tasks such as filtering out unwanted data, finding specific items in an array, or transforming data in a specific way.
To use the filter method, you first need to have an array that you want to filter. Here is an example of an array of numbers that we will use for demonstration:
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
Now, let’s say we want to create a new array that only contains numbers greater than 5. We can use the filter method to achieve this:
const filteredNumbers = numbers.filter(number => number > 5);
console.log(filteredNumbers);
In this example, we are using an arrow function as the filter condition. The filter method will iterate over each element in the array and pass it to the arrow function. If the condition inside the arrow function evaluates to true, the element will be added to the new array. In this case, only numbers greater than 5 will be included in the filteredNumbers array.
You can also use the filter method to filter objects in an array. For example, let’s say we have an array of objects representing cars and we want to filter out only the cars that are red:
const cars = [
{ make: ‘Toyota’, model: ‘Camry’, color: ‘blue’ },
{ make: ‘Ford’, model: ‘Mustang’, color: ‘red’ },
{ make: ‘Chevrolet’, model: ‘Camaro’, color: ‘red’ },
{ make: ‘Honda’, model: ‘Accord’, color: ‘silver’ }
];
const redCars = cars.filter(car => car.color === ‘red’);
console.log(redCars);
In this example, we are filtering out only the cars that have a color property with a value of ‘red’. The filter method will create a new array containing only the red cars.
The filter method also allows you to chain multiple filter conditions together. For example, let’s say we want to filter out only the cars that are red and made by Ford:
const redFordCars = cars.filter(car => car.color === ‘red’)
.filter(car => car.make === ‘Ford’);
console.log(redFordCars);
In this example, we are using two filter methods chained together. The first filter method filters out only the red cars, and the second filter method filters out only the cars made by Ford. The result is a new array containing only the red Ford cars.
In conclusion, the filter method in JavaScript is a powerful tool for manipulating arrays. By mastering the filter method, you can easily filter out unwanted data, find specific items in an array, or transform data in a specific way. Practice using the filter method with different examples to become more comfortable with its syntax and functionality. With enough practice, you will be able to use the filter method effectively in your JavaScript projects.