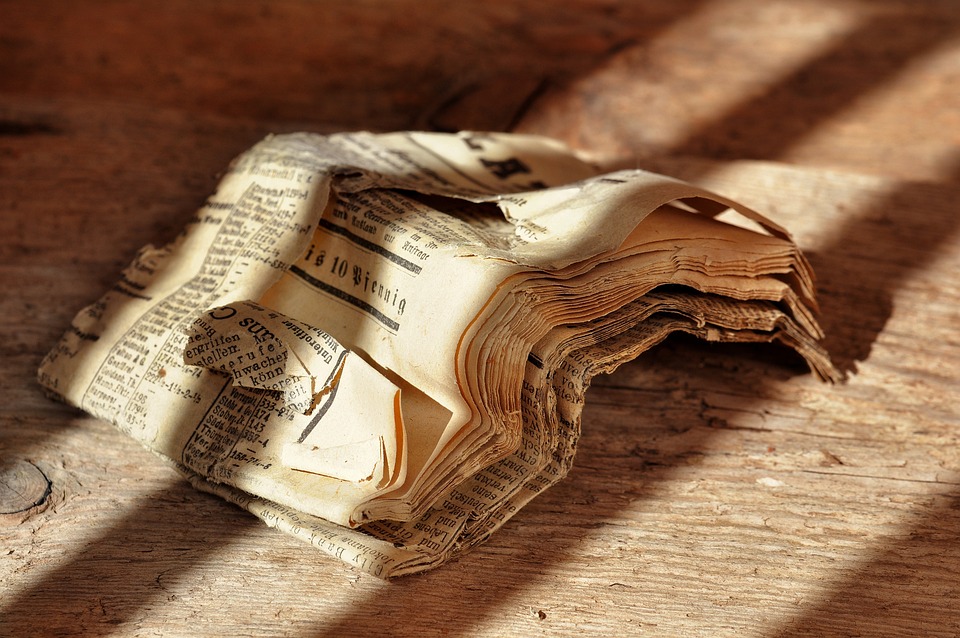
JavaScript arrays are a fundamental data structure in the language, allowing developers to store and manipulate collections of data efficiently. With the right techniques, you can master JavaScript arrays and make your code more efficient and readable.
Here are some tips and tricks for efficient data manipulation using JavaScript arrays:
1. Use Array Methods: JavaScript Arrays come with a variety of built-in methods that make data manipulation easier. Some commonly used array methods include map(), filter(), reduce(), and forEach(). These methods allow you to iterate over arrays, filter out elements based on a condition, and perform calculations on array elements.
2. Avoid Nesting Arrays: While it is possible to nest arrays within arrays, it is generally not recommended as it can make your code more complex and harder to read. If you find yourself nesting arrays, consider using objects or other data structures instead.
3. Use Spread Operator: The spread operator (…) can be used to easily concatenate arrays, copy arrays, and pass arrays as arguments to functions. This can save you time and make your code more concise.
4. Avoid Mutating Arrays: When manipulating arrays, it is important to avoid mutating the original array if possible. Instead, consider using methods like map() or filter() to create a new array with the desired changes.
5. Use Destructuring: Destructuring allows you to easily unpack values from arrays and objects, making it easier to work with complex data structures. This can save you time and make your code more readable.
6. Use Array.isArray() to Check for Arrays: If you need to check if a variable is an array, you can use the Array.isArray() method. This can help prevent errors in your code.
7. Use Array.from() to Convert Arrays: If you have an array-like object that you want to convert to an array, you can use the Array.from() method. This can be useful when working with DOM elements or other array-like objects.
By mastering JavaScript arrays and using these tips and tricks, you can make your code more efficient and easier to maintain. Practice implementing these techniques in your projects to become a more proficient JavaScript developer.