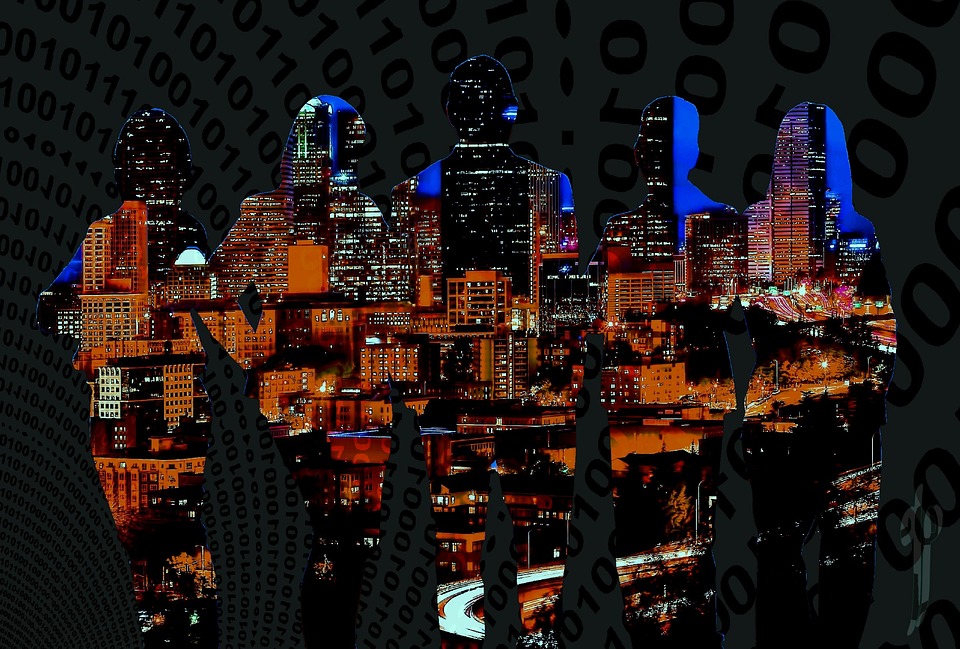
JavaScript arrays are a fundamental part of any developer’s toolkit. They allow us to store and manipulate collections of data in a flexible and efficient way. In this comprehensive guide, we will explore the ins and outs of JavaScript arrays, from basic operations to advanced techniques for mastering them.
Creating Arrays
To create an array in JavaScript, you can use the array literal syntax, which consists of square brackets enclosing a list of elements separated by commas. For example:
const myArray = [1, 2, 3, 4, 5];
You can also create an empty array and add elements to it later using the push method:
const myArray = [];
myArray.push(1);
myArray.push(2);
myArray.push(3);
Accessing Elements
You can access individual elements in an array using square bracket notation, with the index of the element you want to retrieve. Remember that array indexes are zero-based, meaning the first element in the array has an index of 0. For example:
const myArray = [1, 2, 3, 4, 5];
console.log(myArray[0]); // Output: 1
console.log(myArray[2]); // Output: 3
Iterating Over Arrays
There are several ways to iterate over the elements of an array in JavaScript. One common method is using a for loop:
const myArray = [1, 2, 3, 4, 5];
for (let i = 0; i {
console.log(element);
});
Manipulating Arrays
JavaScript provides several methods for manipulating arrays, such as adding and removing elements, sorting, filtering, and mapping. Some common array methods include push, pop, shift, unshift, splice, concat, sort, filter, and map. Here’s an example of using the splice method to remove elements from an array:
const myArray = [1, 2, 3, 4, 5];
myArray.splice(2, 1); // Removes the element at index 2
console.log(myArray); // Output: [1, 2, 4, 5]
Advanced Techniques
JavaScript arrays are versatile and offer many advanced techniques for working with data. For example, you can use the map method to transform each element in an array into a new value:
const myArray = [1, 2, 3, 4, 5];
const squaredArray = myArray.map(element => element ** 2);
console.log(squaredArray); // Output: [1, 4, 9, 16, 25]
You can also use the reduce method to perform calculations on the elements of an array and return a single value:
const myArray = [1, 2, 3, 4, 5];
const sum = myArray.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
console.log(sum); // Output: 15
In conclusion, mastering JavaScript arrays is essential for any developer looking to work with data in a flexible and efficient way. By understanding the basics of creating, accessing, iterating, and manipulating arrays, as well as advanced techniques like mapping and reducing, you can take your JavaScript skills to the next level. Practice implementing these concepts in your projects to become a proficient array master.