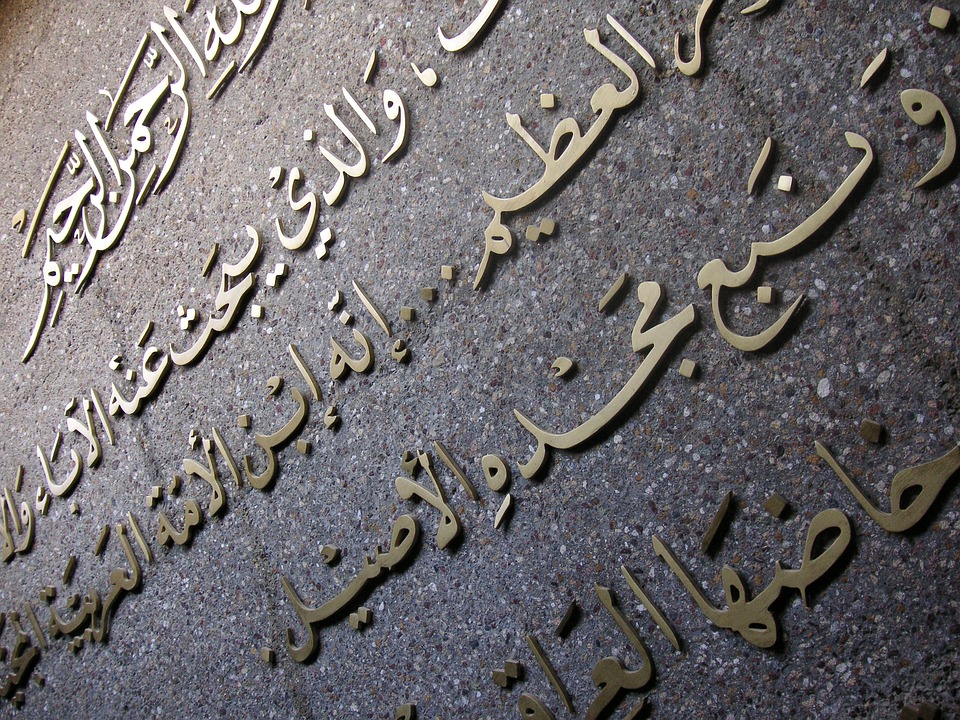
JavaScript arrays are an essential part of any web developer’s toolkit. They allow you to store and manipulate collections of data efficiently. However, working with arrays can sometimes be tricky, especially when it comes to manipulating them. In this article, we will explore various techniques and methods for mastering JavaScript array manipulation.
1. Declaring an Array:
Before we dive into manipulation techniques, let’s start with the basics. To declare an array, use square brackets and separate each element with a comma. For example:
“`
let fruits = [‘apple’, ‘banana’, ‘orange’];
“`
2. Accessing Array Elements:
To access specific elements in an array, use square brackets and specify the index position. Remember that arrays are zero-indexed, meaning the first element is at index 0. For example:
“`
console.log(fruits[0]); // Output: ‘apple’
“`
3. Adding Elements:
To add elements to an array, you can use the `push()` method. It adds one or more elements to the end of the array. For example:
“`
fruits.push(‘grape’);
console.log(fruits); // Output: [‘apple’, ‘banana’, ‘orange’, ‘grape’]
“`
4. Removing Elements:
To remove elements from an array, you can use the `pop()` method. It removes the last element from the array and returns it. For example:
“`
fruits.pop();
console.log(fruits); // Output: [‘apple’, ‘banana’, ‘orange’]
“`
5. Finding the Length:
To find the length of an array, use the `length` property. It returns the number of elements in the array. For example:
“`
console.log(fruits.length); // Output: 3
“`
6. Looping through an Array:
To iterate over each element in an array, you can use a loop. The most common loop for array manipulation is the `for` loop. For example:
“`
for (let i = 0; i