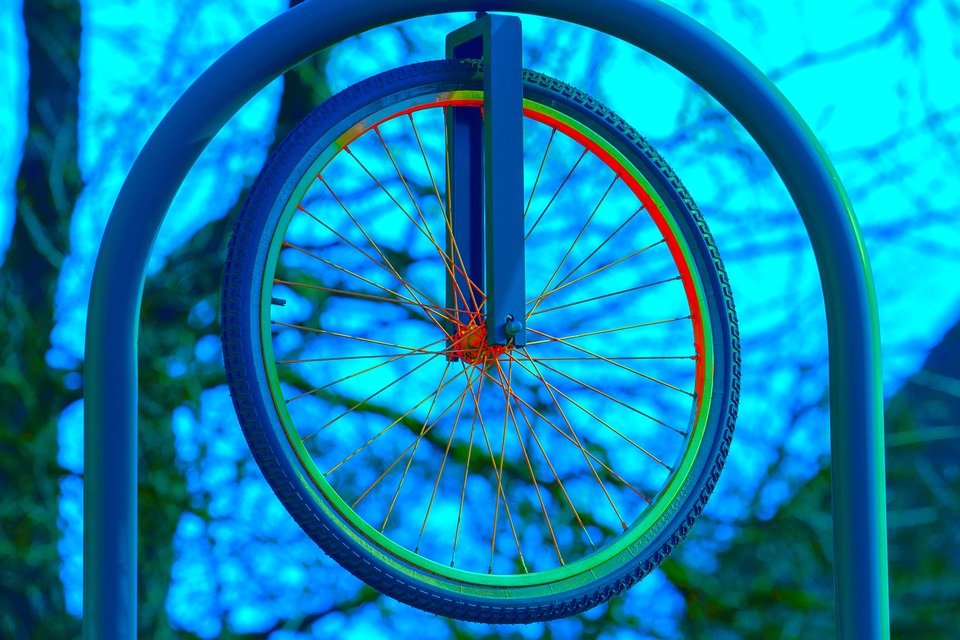
Mastering Go: Practical Code Examples to Elevate Your Programming Skills
Go, also known as Golang, is a powerful programming language that has gained popularity among developers in recent years. It offers a unique combination of simplicity, efficiency, and performance, making it an excellent choice for building scalable and reliable software applications. If you’re looking to take your programming skills to the next level, mastering Go is a great way to do so.
To help you on your journey to becoming a Go master, we’ve compiled a list of practical code examples that will help you understand the language’s key features and improve your programming skills.
1. Hello World
Let’s start with the classic “Hello World” program in Go:
“`go
package main
import “fmt”
func main() {
fmt.Println(“Hello, World!”)
}
“`
This simple program demonstrates the basic structure of a Go program. The `package main` declaration tells the compiler that this is the entry point of the program. The `import “fmt”` statement imports the fmt package, which provides functions for formatting and printing output. The `func main()` function is where the program execution begins, and `fmt.Println(“Hello, World!”)` prints the message “Hello, World!” to the console.
2. Variables and Constants
In Go, you can declare variables using the `var` keyword and constants using the `const` keyword. Here’s an example:
“`go
package main
import “fmt”
func main() {
var name string = “Alice”
const age = 30
fmt.Printf(“%s is %d years old\n”, name, age)
}
“`
In this example, we declare a variable `name` of type `string` and initialize it with the value “Alice”. We also declare a constant `age` with the value 30. The `fmt.Printf` function is used to print the values of `name` and `age` to the console.
3. Arrays and Slices
Arrays and slices are important data structures in Go. Here’s an example of how to declare an array and a slice in Go:
“`go
package main
import “fmt”
func main() {
// Array
var numbers [5]int
numbers[0] = 1
numbers[1] = 2
fmt.Println(numbers)
// Slice
names := []string{“Alice”, “Bob”, “Charlie”}
fmt.Println(names)
}
“`
In this example, we declare an array `numbers` of type `int` with a length of 5 and initialize its first two elements. We then declare a slice `names` of type `string` and initialize it with three names. The `fmt.Println` function is used to print the contents of the array and slice.
4. Functions
Functions are essential building blocks in Go. Here’s an example of how to define and call a function in Go:
“`go
package main
import “fmt”
func add(a, b int) int {
return a + b
}
func main() {
sum := add(3, 5)
fmt.Println(sum)
}
“`
In this example, we define a function `add` that takes two integer parameters `a` and `b` and returns their sum. We then call the `add` function with arguments 3 and 5 and print the result to the console.
5. Goroutines and Channels
Goroutines and channels are powerful features in Go for concurrent programming. Here’s an example of how to use them:
“`go
package main
import “fmt”
func worker(id int, jobs