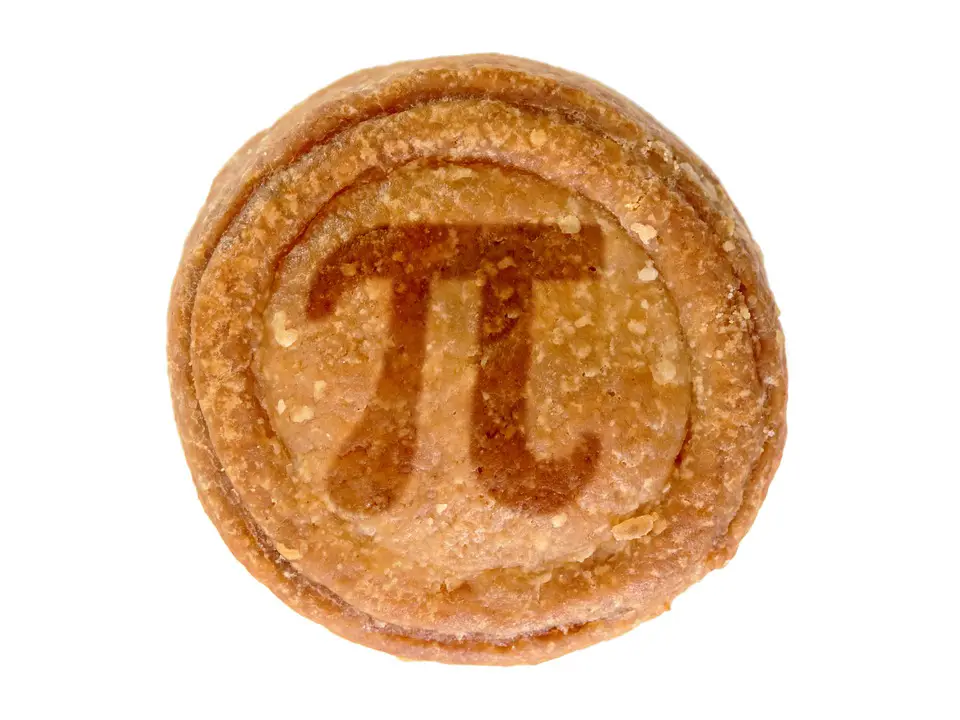
Python’s split method is a versatile and powerful tool that allows you to easily split strings into substrings based on a specified delimiter. Whether you are a beginner or an experienced Python programmer, mastering the split method can greatly enhance your coding abilities and efficiency. In this article, we will provide you with expert tips on how to effectively use the split method in Python.
1. Understand the Basics
The split method in Python is used to split a string into a list of substrings based on a specified delimiter. By default, the delimiter is a space character, but you can also specify any other character or sequence of characters as the delimiter. The syntax for the split method is as follows:
“` python
string.split(delimiter)
“`
where `string` is the string that you want to split and `delimiter` is the character or sequence of characters that you want to use as the delimiter.
2. Use the split method with different delimiters
One of the key advantages of the split method is its flexibility in terms of specifying the delimiter. In addition to using a single character as the delimiter, you can also use multiple characters or even a regular expression pattern. For example, if you want to split a string based on a comma delimiter, you can do so as follows:
“` python
string = “apple,banana,orange”
fruits = string.split(“,”)
print(fruits)
“`
Output:
“`
[‘apple’, ‘banana’, ‘orange’]
“`
3. Handle edge cases
When using the split method, it is important to consider edge cases where the delimiter may not be present in the string or where the string is empty. To handle these cases, you can use conditional statements to check for the presence of the delimiter before splitting the string. For example:
“` python
string = “apple,banana,orange”
if “,” in string:
fruits = string.split(“,”)
print(fruits)
else:
print(“Delimiter not found”)
“`
4. Use the maxsplit parameter
The split method in Python also allows you to specify the maximum number of splits to perform. This can be useful when you only want to split the string into a certain number of substrings. The syntax for using the maxsplit parameter is as follows:
“` python
string.split(delimiter, maxsplit)
“`
For example, if you want to split a string into two substrings based on a comma delimiter, you can do so as follows:
“` python
string = “apple,banana,orange”
fruits = string.split(“,”, 1)
print(fruits)
“`
Output:
“`
[‘apple’, ‘banana,orange’]
“`
5. Use list comprehension
Another useful technique when working with the split method is to use list comprehension to process the resulting list of substrings. This allows you to perform additional operations on each substring in a concise and efficient manner. For example, if you want to convert all substrings to uppercase after splitting the string, you can do so as follows:
“` python
string = “apple,banana,orange”
fruits = string.split(“,”)
uppercase_fruits = [fruit.upper() for fruit in fruits]
print(uppercase_fruits)
“`
In conclusion, mastering the split method in Python can greatly enhance your coding abilities and efficiency. By understanding the basics, using different delimiters, handling edge cases, utilizing the maxsplit parameter, and leveraging list comprehension, you can effectively split strings into substrings and process them in a variety of ways. With these expert tips, you can confidently use the split method in Python to tackle a wide range of programming tasks.