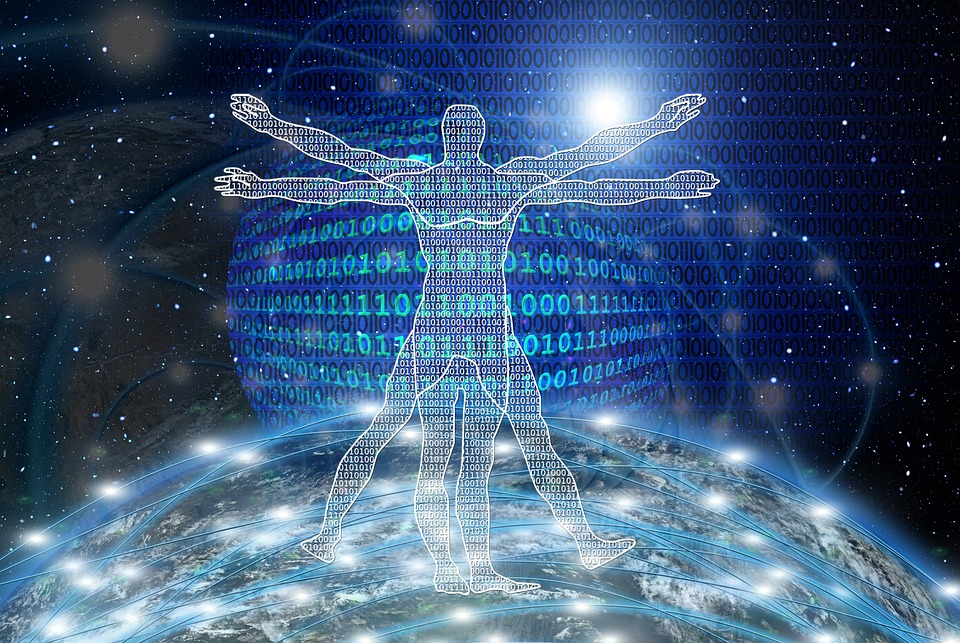
JavaScript is a versatile and powerful programming language that is widely used in web development. One of the most commonly used methods in JavaScript is the forEach method, which allows you to iterate over elements in an array and perform a specific action on each element. Mastering the forEach method can help you write more efficient and concise code. In this article, we will provide you with expert tips on how to make the most of the forEach method in JavaScript.
1. Understand the Syntax:
Before diving into using the forEach method, it is important to understand its syntax. The forEach method takes a callback function as an argument, which is executed for each element in the array. The callback function can take up to three arguments: the current element being processed, the index of the current element, and the array itself.
“`
array.forEach(function (element, index, array) {
// code to be executed
});
“`
2. Use Arrow Functions:
Arrow functions are a concise way to write functions in JavaScript, and they work particularly well with the forEach method. Instead of using a traditional function declaration, you can use an arrow function to make your code more readable and compact.
“`
array.forEach((element, index, array) => {
// code to be executed
});
“`
3. Avoid Using the Return Statement:
The forEach method does not return a value, so using the return statement inside the callback function will not have any effect. If you need to return a new array or perform some other operation on the elements, consider using the map method instead.
“`
const newArray = [];
array.forEach((element) => {
newArray.push(element * 2);
});
“`
4. Use the Index Parameter:
The index parameter in the callback function can be useful if you need to access the index of the current element in the array. This can be helpful when you need to perform different actions based on the position of the element in the array.
“`
array.forEach((element, index) => {
if (index % 2 === 0) {
console.log(element);
}
});
“`
5. Encapsulate Complex Logic in Functions:
If the code inside the callback function is complex or requires multiple lines of code, consider encapsulating that logic in a separate function. This can make your code more readable and maintainable.
“`
function processElement(element) {
// complex logic here
}
array.forEach((element) => {
processElement(element);
});
“`
By following these expert tips, you can master the JavaScript forEach method and use it effectively in your code. Remember to understand the syntax, use arrow functions, avoid using the return statement, leverage the index parameter, and encapsulate complex logic in functions. With these tips in mind, you can write more efficient and concise code using the forEach method in JavaScript.