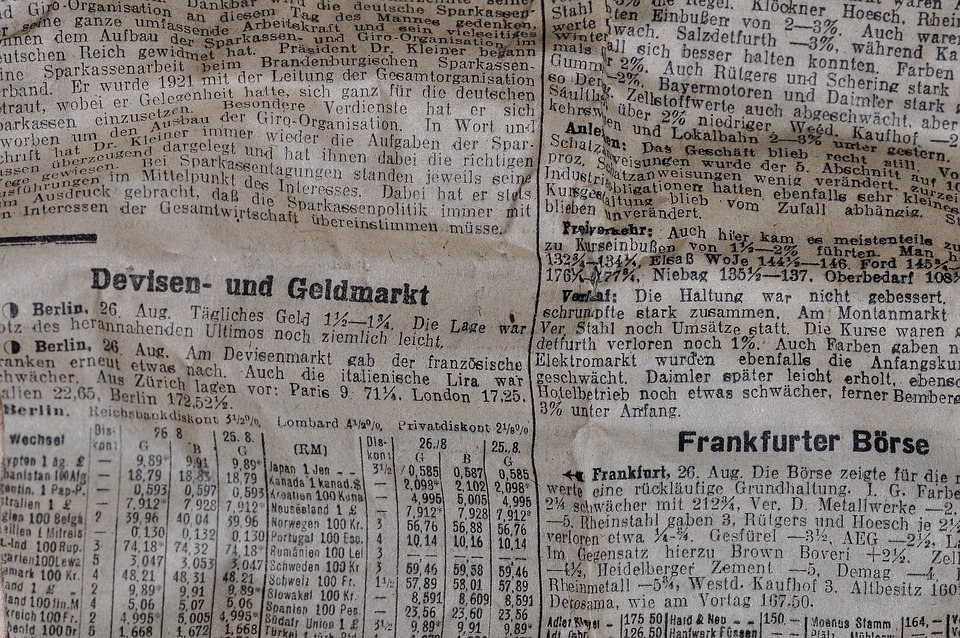
JavaScript is a powerful programming language that is essential for creating dynamic and interactive web pages. Whether you are a beginner or a seasoned developer, mastering the basics of JavaScript is crucial for building successful websites and applications.
To help beginners get started with JavaScript, we have put together a beginner’s guide that covers the fundamental concepts and techniques that you need to know. By mastering the basics, you will be well on your way to becoming a proficient JavaScript developer.
Variables and Data Types
One of the fundamental concepts in JavaScript is variables. A variable is a container that holds a value, which can be a number, string, boolean, or any other data type. To declare a variable in JavaScript, you use the `var`, `let`, or `const` keywords followed by the variable name and optional initial value.
For example:
“`
var x = 10;
let message = “Hello, World!”;
const PI = 3.14;
“`
JavaScript supports several data types, including numbers, strings, booleans, arrays, and objects. Understanding data types and how to work with them is essential for writing effective JavaScript code.
Functions
Functions are reusable blocks of code that perform a specific task. In JavaScript, functions are defined using the `function` keyword followed by the function name and optional parameters.
For example:
“`
function greet(name) {
return “Hello, ” + name + “!”;
}
“`
Functions can take parameters and return values, allowing you to create modular and flexible code. Mastering functions is essential for organizing your code and making it more readable and maintainable.
Control Structures
Control structures allow you to control the flow of your code based on certain conditions. JavaScript supports several control structures, including if statements, for loops, while loops, and switch statements.
For example:
“`
if (x > 0) {
console.log(“x is positive”);
} else {
console.log(“x is negative”);
}
“`
Understanding how to use control structures effectively is essential for creating dynamic and interactive web applications.
DOM Manipulation
The Document Object Model (DOM) is a programming interface that allows you to manipulate the elements of a web page. JavaScript allows you to interact with the DOM by selecting elements, changing their attributes, and responding to user events.
For example:
“`
let heading = document.getElementById(“heading”);
heading.textContent = “Hello, World!”;
“`
Mastering DOM manipulation is crucial for creating dynamic and interactive web pages that respond to user input.
Conclusion
Mastering the basics of JavaScript is essential for anyone looking to become a proficient web developer. By understanding variables, functions, control structures, and DOM manipulation, you will be well-equipped to create dynamic and interactive web pages and applications.
If you are a beginner looking to learn JavaScript, consider starting with online tutorials, courses, and practice exercises. By practicing regularly and building small projects, you will gain hands-on experience and improve your skills.
Remember, mastering JavaScript takes time and dedication, so be patient and keep learning. With practice and perseverance, you will become a proficient JavaScript developer in no time.